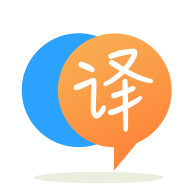
[英]EDIT: How do I send this post Request with parameters AND form-data like this postman screenshot in JAVA?
[英]How can I do a Post request like this in java?
我有一个Curl这样的Post请求程序。 我该如何在Java中准确地完成这项工作?
curl -X POST \
-H "api_key: xxxxxxxxxxxxx" \
-H "speed: 0" \
-H "voice: male" \
-H "prosody: 1" \
-H "Cache-Control: no-cache" \
-d 'This is the text to transfer' \
"http://somewhere.com"
我使用Apache创建POST
请求的可运行示例
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
...
public static void main(String[] args) throws IOException {
HttpClient httpclient = HttpClients.createDefault();
HttpPost httppost = new HttpPost("https://reqres.in/api/register");
// Simple data
httppost.setEntity(
new StringEntity("{\"email\":\"eve.holt@reqres.in\",\"password\":\"pistol\"}",ContentType.create("application/json", StandardCharsets.UTF_8)));
// Simple Header
httppost.setHeader("cache-control", "no-cache");
// Execute and get the response.
HttpResponse response = httpclient.execute(httppost);
HttpEntity entity = response.getEntity();
System.out.println("Status: " + response.getStatusLine().toString());
if (entity != null) {
try (InputStream instream = entity.getContent()) {
System.out.println("response: " + IOUtils.toString(instream, StandardCharsets.UTF_8));
}
}
}
来自System.out.println()
响应
状态:HTTP / 1.1 200 OK
响应:{“ id”:4,“令牌”:“ QpwL5tke4Pnpja7X4”}
用Java转换代码
String url = ""http://somewhere.com";
HttpClient client = new DefaultHttpClient();
HttpPost post = new HttpPost(url);
// add header
post.setHeader("api_key", "xxxxxxxxxxxxx");
post.setHeader("speed", "0");
post.setHeader("voice", "male");
post.setHeader("prosody", "1");
post.setHeader("Cache-Control", "no-cache");
post.setEntity(new StringEntity("This is the text to transfer",ContentType.create("text/plain", "UTF-8")));
HttpResponse response = client.execute(post);
System.out.println("\nSending 'POST' request to URL : " + url);
System.out.println("Post parameters : " + post.getEntity());
System.out.println("Response Code : " +
response.getStatusLine().getStatusCode());
BufferedReader rd = new BufferedReader(
new InputStreamReader(response.getEntity().getContent()));
StringBuffer result = new StringBuffer();
String line = "";
while ((line = rd.readLine()) != null) {
result.append(line);
}
System.out.println(result.toString());
希望能帮助到你。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.