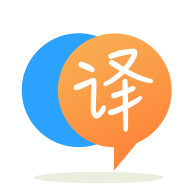
[英]React Functional Component Re-renders exponentially with array state change
[英]Restricting re-renders to specific state change in functional component
如果我有一个具有三种不同状态的组件,并且只希望当该组件中的一个特定状态发生更改时重新渲染该组件,我该怎么做呢?
下面,我提供了一个代码示例。 说我只希望在调用setSecondState()时重新渲染此组件,而不是在调用setFirstState()或setThirdState()时重新渲染。
const ExampleComponent = () => {
const [firstState, setFirstState] = React.useState(0);
const [secondState, setSecondState] = React.useState(false);
const [thirdState, setThirdState] = React.useState("");
return (
<div>
Rendered
</div>
);
}
不要将其置于使用ref的状态。 您可以通过更新ref.current来更新ref值,不会引起重新渲染。
const ExampleComponent = () => {
const firstRef = React.useRef(0);
const [secondState, setSecondState] = React.useState(false);
const secondRef = React.useRef("");
const someFunction = () => {
firstRef.current = 10;
secondRef.current = "foo";
}
return (
<div>
Rendered
</div>
);
}
您可以使用shouldComponentUpdate生命周期函数。 这将返回一个布尔值,指示是否响应渲染。
在下面的示例中,当v1值更改时,它不会触发重新渲染,而更改v2则触发重新渲染。
class App extends React.Component { state = { v1: 0, v2: 0 } shouldComponentUpdate(nextProps, nextState){ console.log('v1: ', nextState.v1, ', v2: ', nextState.v2); return this.state.v1 === nextState.v1 } render(){ const { v1, v2 } = this.state; return ( <div> <button onClick={() => this.setState(({ v1 }) => ({ v1: v1 + 1 }))}>{`Increase but do not render: ${v1}`}</button><br /> <button onClick={() => this.setState(({ v2 }) => ({ v2: v2 + 1 }))}>{`Increase and render: ${v2}`}</button> </div> ) } } ReactDOM.render(<App />, document.getElementById('root'));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/16.6.3/umd/react.production.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/16.6.3/umd/react-dom.production.min.js"></script> <div id="root"></div>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.