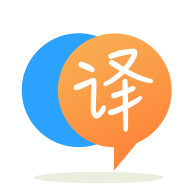
[英]How to write loop of data in the same excel sheet in Java using jlx?
[英]How to write data in excel sheet in specific cell using servlet?
我是 Servlet 的新手,请帮助我。 我创建了一个 Servlet 文件,其中一个 excel 文件包含来自用户的一些数据。 数据通过字符串打印。 现在,大部分数据都按照预期打印,但现在我想在 Excel 工作表的特定单元格中以特定格式打印数组存储的数据。 请参阅提供的照片以了解我所期望的格式。 并参考我的代码以获得更好的理解。 主要目标是在excel表的特定单元格中打印数组存储的数据。 请帮助我编写代码,因为我对 Servlet 非常陌生。 参考这个链接
测试Excel
@WebServlet("/testExcel")
public class testExcel extends HttpServlet {
private static final long serialVersionUID = 1L;
String emails = "xyz@gmail.com";
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
processRequest(req, resp);
}
protected void processRequest(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
OutputStream writer = response.getOutputStream();
// FileWriter writer =null;
ResultSet rs = null;
ResultSet rs1 = null;
Connection con = null;
ArrayList data_ar = new ArrayList();
String str = "";
PreparedStatement ps = null;
PreparedStatement ps1 = null;
try {
String fileName = emails + "data.csv";
System.out.println(fileName);
ServletContext context = getServletContext();
String mimeType = context.getMimeType(fileName);
if (mimeType == null) {
mimeType = "application/octet-stream";
}
response.setContentType(mimeType);
String headerKey = "Content-Disposition";
String headerValue = String.format("attachment; filename=\"%s\"", fileName);
response.setHeader(headerKey, headerValue);
ConnectionClass cn = new ConnectionClass();
con = cn.connectDb();
System.out.println("fileName" + fileName);
//Write the CSV file header
CSVUtil.writeLine(writer, Arrays.asList("NAME", "email", " ", " ", " ", "NAME AND EMAIL"));
ps = con.prepareStatement("select name,email from user");
rs = ps.executeQuery();
ps1 = con.prepareStatement("select name,email from user");
rs1 = ps1.executeQuery();
while (rs.next()) {
System.out.println(rs.getString("name"));
CSVUtil.writeLine(writer, Arrays.asList(rs.getString("name"), rs.getString("email")));
}
while (rs1.next()) {
data_ar.add(rs1.getString("name") + " " + rs1.getString("email") + "\n");
}
str = String.join(" ", data_ar);
CSVUtil.writeLine(writer, Arrays.asList(" ", " ", " ", " ", " ", str));
writer.flush();
writer.close();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
rs.close();
ps.close();
con.close();
} catch (Exception e) {
}
}
}
}
csvutil.java
public class CSVUtil {
private static final char DEFAULT_SEPARATOR = ',';
public static void writeLine(OutputStream w, List<String> values) throws IOException {
writeLine(w, values, DEFAULT_SEPARATOR, ' ');
}
public static void writeLine(OutputStream w, List<String> values, char separators) throws IOException {
writeLine(w, values, separators, ' ');
}
// https://tools.ietf.org/html/rfc4180
private static String followCVSformat(String value) {
String result = value;
if (result.contains("\"")) {
result = result.replace("\"", "\"\"");
}
return result;
}
public static void writeLine(OutputStream w, List<String> values, char separators, char customQuote) throws IOException {
boolean first = true;
// default customQuote is empty
if (separators == ' ') {
separators = DEFAULT_SEPARATOR;
}
StringBuilder sb = new StringBuilder();
for (String value : values) {
if (!first) {
sb.append(separators);
}
if (customQuote == ' ') {
sb.append(followCVSformat(value));
} else {
sb.append(customQuote).append(followCVSformat(value)).append(customQuote);
}
first = false;
}
sb.append("\n");
String str = sb.toString();
byte[] bytes = str.getBytes(Charset.forName("UTF-8"));
w.write(bytes);
}
}
简单快速的回答:
您应该尝试使用Apache POI :
Maven 依赖:
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.15</version>
</dependency>
在excel文件中写入列表的简单类:
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class ApachePOIExcelWrite {
private static final String FILE_NAME = "/tmp/MyFirstExcel.xlsx";
public static void main(String[] args) {
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet = workbook.createSheet("Datatypes in Java");
Object[][] datatypes = {
{"Datatype", "Type", "Size(in bytes)"},
{"int", "Primitive", 2},
{"float", "Primitive", 4},
{"double", "Primitive", 8},
{"char", "Primitive", 1},
{"String", "Non-Primitive", "No fixed size"}
};
int rowNum = 0;
System.out.println("Creating excel");
for (Object[] datatype : datatypes) {
Row row = sheet.createRow(rowNum++);
int colNum = 0;
for (Object field : datatype) {
Cell cell = row.createCell(colNum++);
if (field instanceof String) {
cell.setCellValue((String) field);
} else if (field instanceof Integer) {
cell.setCellValue((Integer) field);
}
}
}
try {
FileOutputStream outputStream = new FileOutputStream(FILE_NAME);
workbook.write(outputStream);
workbook.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("Done");
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.