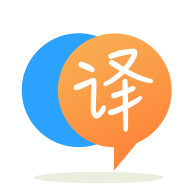
[英]Best way to combine data of object/array to an attribute in JavaScript
[英]Is there a good way to combine array and object in JavaScript?
有一个对象和一个数组。
list: [
{
oldData: {
title: 'abc',
id: 1,
date: '1982-09-30',
budget: 250000,
},
newData: [
{
key: 1,
data: null,
value: 5,
},
{
key: 2,
data: null,
value: 22,
},
...
],
},
{
oldData: {
title: 'blablablaaaaa',
id: 2,
date: '2012-02-23',
budget: 350000,
},
newData: [
{
key: 1,
data: null,
value: 35,
},
{
key: 2,
data: null,
value: 41,
},
...
],
},
... some more datas...
]
同上,同类型的数据比较多。
我需要一起使用oldData and newData
,所以我想将两者结合起来。
如何组合oldData and newData
以便有多组oldData and newData pairs
?
例如, [{ combineData: {...} }, { combineData: {...} }, ... }]
在这里。
我知道如何组合数组和数组、对象和对象,但我不知道该怎么做。
有什么好的解决办法吗?
您可以在数组上使用map()
。 并使用Object.assign()
和 spread 运算符将newData
中所有元素的所有属性newData
为一个对象。
const arr = [ { oldData: { a:10, b:20 }, newData: [ { c:30, d:40 }, { e:50, f:60 } ], } ] const res = arr.map(x => ({combinedData:{...x.oldData, ...Object.assign({}, ...x.newData)}})) console.log(res)
你想要的结果不清楚,但你确实说过你想要旧/新对。 这个答案与其他答案不同,因为它生成由旧/新对组成的组合数据对象数组,其中oldData
值被复制,以便与列表项中每个对应的newData
值一起出现。
第一个问题更新后的原始数据:
let list = [
{
oldData: { title: 'abc', id: 1, date: '1982-09-30', budget: 250000 },
newData: [
{ key: 1, data: null, value: 5 },
{ key: 2, data: null, value: 22 },
//...
],
},
{
oldData: { title: 'blablablaaaaa', id: 2, date: '2012-02-23', budget: 350000 },
newData: [
{ key: 1, data: null, value: 35 },
{ key: 2, data: null, value: 41 },
//...
],
},
//... some more datas...
];
此代码将每个{old,new[]}
列表项映射到[{old,new}, {old,new}, ...]
对的数组中,这些对组合在最终的reduce()
调用中:
var combinedDatas = list
.map(listItem => listItem.newData.map(newItem => ({
oldData: listItem.oldData,
newData: newItem
})))
.reduce();
console.log(JSON.stringify(oldNewCombos, null, 4));
生成非规范化对的列表:
[
{ list[0].oldData, list[0].newData[0] },
{ list[0].oldData, list[0].newData[1] },
//...rest of list[0] oldData with newData[n] combos
{ list[1].oldData, list[1].newData[0] },
{ list[1].oldData, list[1].newData[1] },
//...rest of list[1] oldData with newData[n] combos
{ list[2].oldData, list[2].newData[0] },
{ list[2].oldData, list[2].newData[1] },
//...rest of list[2] oldData with newData[n] combos
//...
]
您可以映射数组并使用对象解构 ( ...object
) 创建一个新的组合对象:
const data = [ { oldData: { foo: 'lorem', }, newData: { bar: 'ipsum', }, }, { oldData: { foo: 'dolor', }, newData: { bar: 'sit amet', }, }, ]; const combined = data.map(record => ({...record.oldData, ...record.newData})); console.log(combined);
但是,这将覆盖重复的键,例如:
{
oldData: {
message: 'a',
},
newData: {
message: 'b',
},
}
会变成:
{
message: 'b',
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.