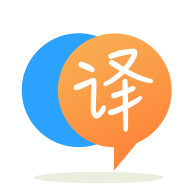
[英]javascript JSON : Sum two or more json having only numeric values with same keys
[英]Finding sum of keys in json having same values
所以我有一个包含板球比赛信息的数组。 我想显示一个团队在一场比赛中得分的totalRun,同时还显示了与其他团队相对的totalRun。
数组包含
module.exports.matchScore = [
{
"fk_matchID": 234017,
"fk_teamID": 198708,
"inning": 1,
"isDeclare": 0,
"isForfeited": 0,
"isFollowOn": 0,
"isAllOut": 0,
"totalRun": 404,
"totalWicket": 10
},
{
"fk_matchID": 234017,
"fk_teamID": 198752,
"inning": 2,
"isDeclare": 0,
"isForfeited": 0,
"isFollowOn": 0,
"isAllOut": 0,
"totalRun": 280,
"totalWicket": 10
},
{
"fk_matchID": 234017,
"fk_teamID": 198708,
"inning": 3,
"isDeclare": 1,
"isForfeited": 0,
"isFollowOn": 0,
"isAllOut": 0,
"totalRun": 81,
"totalWicket": 4
},
{
"fk_matchID": 234017,
"fk_teamID": 198752,
"inning": 4,
"isDeclare": 0,
"isForfeited": 0,
"isFollowOn": 0,
"isAllOut": 0,
"totalRun": 15,
"totalWicket": 0
},
let matchScore = require('./array');
let matchScoreData = matchScore.matchScore;
let forMatchDetail = matchScore.filter(function (matchScoreDetail) {
return (matchScoreDetail.fk_matchID === matchScoreDetail.fk_matchID) && (matchScoreDetail.fk_teamID === matchScoreDetail.fk_teamID);
});
console.log(forMatchDetail);
let againstMatchDetail = matchScore.filter(function (matchScore) {
return (matchScore.fk_matchID === matchScoreData.fk_matchID) && (matchScore.fk_teamID != matchScoreData.fk_teamID);
});
console.log(againstMatchDetail);
所以我想从同一比赛中的不同局对象添加一个团队totalRun(相同的比赛ID)
因此,团队198708的总跑步次数将为485 404 + 81,而团队198752的得分将为295 280 + 15
因此,团队198708的得分为485,反对将为295,而小组198752的得分为295,反对将为485
我得到了你们给出的答案,但是我该如何将我的答案换成车队的totalRun,以便与run socred即
“ 198708”:295,“ 198752”:485,
在示例中,我在数组中也有一些相似的匹配项。 所以我需要在matchID中显示它。
您需要遍历数组并找到正确的团队ID,然后将团队ID插入具有分数的新对象中。 如果对象中已经有团队ID,则添加分数。 以下是为您准备的虚拟逻辑。
var match_results = [ { "fk_matchID": 234017, "fk_teamID": 198708, "inning": 1, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 404, "totalWicket": 10 }, { "fk_matchID": 234017, "fk_teamID": 198752, "inning": 2, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 280, "totalWicket": 10 }, { "fk_matchID": 234017, "fk_teamID": 198708, "inning": 3, "isDeclare": 1, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 81, "totalWicket": 4 }, { "fk_matchID": 234017, "fk_teamID": 198752, "inning": 4, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 15, "totalWicket": 0 }]; var desired_result = {}; for (let i = 0; i< match_results.length; i++){ // console.log(match_results[i]); if(desired_result[match_results[i]["fk_teamID"]] == undefined){ desired_result[match_results[i]["fk_teamID"]] = match_results[i]["totalRun"]; }else{ desired_result[match_results[i]["fk_teamID"]] += match_results[i]["totalRun"]; } } console.log(desired_result);
使用减少方法
var match_results = [ { "fk_matchID": 234017, "fk_teamID": 198708, "inning": 1, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 404, "totalWicket": 10 }, { "fk_matchID": 234017, "fk_teamID": 198752, "inning": 2, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 280, "totalWicket": 10 }, { "fk_matchID": 234017, "fk_teamID": 198708, "inning": 3, "isDeclare": 1, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 81, "totalWicket": 4 }, { "fk_matchID": 234017, "fk_teamID": 198752, "inning": 4, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 15, "totalWicket": 0 }]; // using reduce function. var desired_result_2 = match_results.reduce(function(accu, value, currentIndex, array) { if(accu[value["fk_teamID"]] == undefined){ accu[value["fk_teamID"]] = value["totalRun"]; }else{ accu[value["fk_teamID"]] += value["totalRun"]; } return accu; },{}); console.log(desired_result_2);
请尝试此代码并进行所需的更改
var matchScore = [ { "fk_matchID": 234017, "fk_teamID": 198708, "inning": 1, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 404, "totalWicket": 10 }, { "fk_matchID": 234017, "fk_teamID": 198752, "inning": 2, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 280, "totalWicket": 10 }, { "fk_matchID": 234017, "fk_teamID": 198708, "inning": 3, "isDeclare": 1, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 81, "totalWicket": 4 }, { "fk_matchID": 234017, "fk_teamID": 198752, "inning": 4, "isDeclare": 0, "isForfeited": 0, "isFollowOn": 0, "isAllOut": 0, "totalRun": 15, "totalWicket": 0 } ]; var matchScoreCopy = matchScore; var jsonObj = []; var obj; var getJsonObject = function (matchScoreCopy) { if (matchScoreCopy.length === 0) { return -1; } else { var elementId = matchScoreCopy[0].fk_teamID; var totrun = 0; for(var i = 0; i < matchScoreCopy.length; i++) { var obj = matchScoreCopy[i]; if (obj.fk_teamID === elementId) { totrun += obj.totalRun; matchScoreCopy.splice(i, 1); if (matchScoreCopy.length === 1) { i--; } } } obj = { 'fk_teamID': elementId, 'totalRun': totrun } jsonObj.push(obj); getJsonObject(matchScoreCopy); } } getJsonObject(matchScoreCopy); console.log(jsonObj);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.