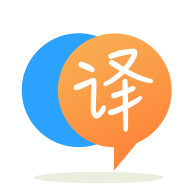
[英]How can i implement Hint View without using showcase library or any other third party library
[英]How can I implement CoroutineWorker using a third-party library that uses callbacks?
我正在尝试实现一个CoroutineWorker,以便在Android应用程序中执行一些后台工作。 我正在使用的第三方库使用诸如onConnected,onChanged等回调。如何在CoroutineWorker中使用该库?
这就是我到目前为止
override suspend fun doWork(): Result {
return try {
val appContext = applicationContext
var mReporter: StepCountReporter?
val mStepCountObserver = object : StepCountReporter.StepCountObserver {
override fun onChanged(count: Int) {
Log.d(APP_TAG, "Step reported : $count")
// This is where the work is completed
}
}
val mConnectionListener = object : HealthDataStore.ConnectionListener {
override fun onConnected() {
Log.d(APP_TAG, "Health data service is connected.")
mReporter = StepCountReporter(mStore!!)
if (isPermissionAcquired) {
mReporter!!.start(mStepCountObserver)
} else {
Log.e(APP_TAG, "permissions not acquired")
}
}
override fun onConnectionFailed(error: HealthConnectionErrorResult) {
Log.d(APP_TAG, "Health data service is not available.")
}
override fun onDisconnected() {
Log.d(APP_TAG, "Health data service is disconnected.")
}
}
mStore = HealthDataStore(appContext, mConnectionListener)
mStore!!.connectService()
// wait for mStepCountObserver.onChanged to be called
} catch (error: Throwable) {
Result.failure()
}
}
我正在尝试完成mStepCountObserver.onChanged中的协程,但看起来我应该在函数末尾调用Result.success
。
您可以使用suspendCoroutine函数,该函数有助于将协程与回调连接起来。
'suspendCoroutine'暂停从其调用的协程,并且仅在调用'resume()'或'resumeWithException()'时恢复该协程。
就你而言
override suspend fun doWork(): Result {
return try {
val outputCount = suspendCoroutine<Int> {
val appContext = applicationContext
var mReporter: StepCountReporter?
val mStepCountObserver = object : StepCountReporter.StepCountObserver {
override fun onChanged(count: Int) {
Log.d(APP_TAG, "Step reported : $count")
// This is where the work is completed
it.resume(Result.success(count))
}
}
val mConnectionListener = object : HealthDataStore.ConnectionListener {
override fun onConnected() {
Log.d(APP_TAG, "Health data service is connected.")
mReporter = StepCountReporter(mStore!!)
if (isPermissionAcquired) {
mReporter!!.start(mStepCountObserver)
} else {
Log.e(APP_TAG, "permissions not acquired")
it.resumeWithException(Exception("permissions not acquired"))
}
}
override fun onConnectionFailed(error: HealthConnectionErrorResult) {
Log.d(APP_TAG, "Health data service is not available.")
it.resumeWithException(Exception("Health data service is not available."))
}
override fun onDisconnected() {
Log.d(APP_TAG, "Health data service is disconnected.")
it.resumeWithException(Exception("Health data service is disconnected."))
}
}
mStore = HealthDataStore(appContext, mConnectionListener)
mStore!!.connectService()
// wait for mStepCountObserver.onChanged to be called
}
Result.success()
} catch (error: Throwable) {
Result.failure()
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.