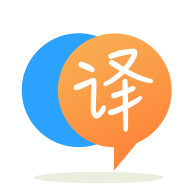
[英]Implementing Bucket Sort and Counting Sort Without Using Dynamic Memory Allocation
[英]dynamic memory allocation using vectors and bubble sort
我需要帮助添加用户以输入成为数组大小的值,并将通过冒泡排序对数组进行排序,但是我需要用户输入该值并使其成为数组的值,即。 动态分配内存
#include <iostream>
#include <vector>
//function to swap values
//need to pass by reference to sort the original values and not just these copies
void Swap (int *a, int *b)
{
int temp = *a;
*a = *b;
*b = temp;
}
void BubbleSort (std::vector<int> &array)
{
std::cout<<"Elements in the array: "<<array.size()<<std::endl;
//comparisons will be done n times
for (int i = 0; i < array.size(); i++)
{
//compare elemet to the next element, and swap if condition is true
for(int j = 0; j < array.size() - 1; j++)
{
if (array[j] > array[j+1])
Swap(&array[j], &array[j+1]);
}
}
}
//function to print the array
void PrintArray (std::vector<int> array)
{
for (int i = 0; i < array.size(); i++)
std::cout<<array[i]<<" ";
std::cout<<std::endl;
}
int main()
{
std::cout<<"Enter array to be sorted (-1 to end)\n";
std::vector<int> array;
int num = 0;
while (num != -1)
{
std::cin>>num;
if (num != -1)
//add elements to the vector container
array.push_back(num);
}
//sort the array
BubbleSort(array);
std::cout<<"Sorted array is as\n";
PrintArray(array);
return 0;
}
我尝试cin
使用 while 但是数组不打印
我修改了您的版本并向您展示了如何从用户那里获取要排序的元素数量以及如何在数组中动态分配内存。
您只需使用std::vector
的构造函数来定义大小。 看起来像: std::vector<int> array(numberOfElements);
.
整个改编的代码:
#include <iostream>
#include <vector>
//function to swap values
//need to pass by reference to sort the original values and not just these copies
void Swap(int* a, int* b)
{
int temp = *a;
*a = *b;
*b = temp;
}
void BubbleSort(std::vector<int>& array)
{
std::cout << "Elements in the array: " << array.size() << std::endl;
//comparisons will be done n times
for (size_t i = 0; i < array.size(); i++)
{
//compare elemet to the next element, and swap if condition is true
for (size_t j = 0; j < array.size() - 1; j++)
{
if (array[j] > array[j + 1])
Swap(&array[j], &array[j + 1]);
}
}
}
//function to print the array
void PrintArray(std::vector<int> array)
{
for (size_t i = 0; i < array.size(); i++)
std::cout << array[i] << " ";
std::cout << std::endl;
}
int main()
{
std::cout << "Enter the number of data to sort: ";
size_t numberOfElements{ 0 };
std::cin >> numberOfElements;
std::vector<int> array(numberOfElements);
size_t counter{ 0 };
std::cout << "\nEnter "<< numberOfElements << " data\n";
int num = 0;
while ((counter < numberOfElements) &&(std::cin >> num) )
{
array[counter] = num;
++counter;
}
//sort the array
BubbleSort(array);
std::cout << "Sorted array is as\n";
PrintArray(array);
return 0;
}
但是,我建议使用现代 C++ 元素,例如解决问题的算法。
看:
#include <iostream>
#include <vector>
#include <iterator>
#include <algorithm>
int main()
{
size_t numberOfElements{ 0 };
std::vector<int> array{};
std::cout << "Enter the number of data to sort: ";
std::cin >> numberOfElements;
std::cout << "\nEnter " << numberOfElements << " data values:\n";
std::copy_n(std::istream_iterator<int>(std::cin), numberOfElements, std::back_inserter(array));
std::sort(array.begin(), array.end());
std::cout << "\n\nSorted data:\n\n";
std::copy(array.begin(), array.end(), std::ostream_iterator<int>(std::cout, "\n"));
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.