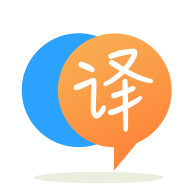
[英]How to force update of view titles after having changed the locale and restarted?
[英]How to update list view with 'titles'
我正在尝试按照 Udemy“完整的 Android N 开发人员课程”实现一个新闻阅读器应用程序。使用列表视图。
根据我已正确遵循的说明,但在执行以下主要活动时,尽管需要更新带有标题的列表项,但在列表视图中没有显示任何内容。 即使在 Android Monitor 中也没有错误。
任何建议找到问题,请。
谢谢!
public class MainActivity extends AppCompatActivity {
ArrayList<String > titles = new ArrayList<>();
ArrayList<String> content = new ArrayList<>();
ArrayAdapter arrayAdapter;
SQLiteDatabase articleDB ;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ListView listView = (ListView) findViewById(R.id.listView );
arrayAdapter = new ArrayAdapter(this,android.R.layout.simple_list_item_1,titles);
listView.setAdapter(arrayAdapter);
articleDB = this.openOrCreateDatabase("articles",MODE_PRIVATE,null);
articleDB.execSQL("CREATE TABLE IF NOT EXISTS articles (id INTEGER PRIMARY KEY, articleID INTEGER,title VARCHAR,content VARCHAR)");
updateListView();
DownloadTask task = new DownloadTask();
try {
task.execute("https://hacker-news.firebaseio.com/v0/topstories.json?print=pretty");
}catch(Exception e){
e.printStackTrace();
}
}
//update table
public void updateListView(){
Cursor c = articleDB.rawQuery("SELECT * FROM articles", null);
int contentIndex = c.getColumnIndex("content");
int titleIndex = c.getColumnIndex("title");
if(c.moveToFirst()){
titles.clear();
content.clear();
do{
titles.add(c.getString(titleIndex));
content.add(c.getString(contentIndex));
}while (c.moveToNext());
arrayAdapter.notifyDataSetChanged();
}
}
public class DownloadTask extends AsyncTask<String, Void, String>{
@Override
protected String doInBackground(String... strings) {
String result = "";
URL url;
HttpsURLConnection urlConnection = null;
try {
url = new URL (strings[0]);
urlConnection = (HttpsURLConnection) url.openConnection();
InputStream in = urlConnection.getInputStream();
InputStreamReader reader = new InputStreamReader(in);
int data = reader.read();
while (data != -1){
char current = (char) data;
result += current;
data = reader.read();
}
//Log.i("URLContent",result);
JSONArray jsonArray = new JSONArray(result);
int numberOfItems = 20;
if(jsonArray.length() <20){
numberOfItems = jsonArray.length();
}
//to clear the table before add data
articleDB.execSQL("DELETE FROM articles"); //will clear everything and add a new data
for (int i=0;i<numberOfItems;i++ ){
//Log.i("JSONItem",jsonArray.getString(i));
String articleId = jsonArray.getString(i);
url = new URL("https://hacker-news.firebaseio.com/v0/item/"+articleId+".json?print=pretty");
urlConnection = (HttpsURLConnection) url.openConnection();
in = urlConnection.getInputStream();
reader = new InputStreamReader(in);
data = reader.read();
String articleInfo = "";
while (data!= -1){
char current = (char) data;
articleInfo += current;
data = reader.read();
}
//Log.i("ArticleInfo",articleInfo);
//separate title and URL
JSONObject jsonObject = new JSONObject(articleInfo);
if (!jsonObject.isNull("title") && !jsonObject.isNull("url")){
String articleTitle = jsonObject.getString("title");
String articleURL = jsonObject.getString("url");
//Log.i("info",articleTitle + articleURL);
url = new URL(articleURL);
urlConnection = (HttpsURLConnection) url.openConnection();
in = urlConnection.getInputStream();
reader = new InputStreamReader(in);
data = reader.read();
String articleContent = "";
while (data!= -1){
char current = (char) data;
articleContent += current;
data = reader.read();
}
//Log.i("articleContent",articleContent);
String sql = "INSERT INTO articles(articleID,title,content) VALUES(? , ? , ?)";
SQLiteStatement statement = articleDB.compileStatement(sql);
statement.bindString(1,articleId);
statement.bindString(2,articleTitle);
statement.bindString(3,articleContent);
statement.execute();
}
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(String s) {
super.onPostExecute(s);
//run when the download task is completed
updateListView();
}
}
}
我不相信您的问题是基于 SQLite,而是与数据的检索有关。
问题可能是:-
您没有相应的权限。 因此,您需要检查您的清单是否具有权限以及您是否已请求运行时权限。 请参阅 请求应用程序权限
检索时未使用适当的连接类型,即切换到 HTTP url 的 HTTP 连接。 请参阅下面的代码进行修复(可能是更好的选择)。
例如,修改您的代码(注释掉 中的代码)以跳过数据检索并添加用于测试的数据插入显示插入的数据。
例如:-
protected String doInBackground(String... strings) {
String result = "";
URL url;
HttpsURLConnection urlConnection = null;
HttpURLConnection xurlConnection = null; //ADDED for stage 2 testing
/* <<<<<<<<<<< COMMENT OUT DATA RETRIEVAL >>>>>>>>>>
try {
url = new URL (strings[0]);
urlConnection = (HttpsURLConnection) url.openConnection();
InputStream in = urlConnection.getInputStream();
InputStreamReader reader = new InputStreamReader(in);
int data = reader.read();
while (data != -1){
char current = (char) data;
result += current;
data = reader.read();
}
//Log.i("URLContent",result);
JSONArray jsonArray = new JSONArray(result);
int numberOfItems = 20;
if(jsonArray.length() <20){
numberOfItems = jsonArray.length();
}
//to clear the table before add data
articleDB.execSQL("DELETE FROM articles"); //will clear everything and add a new data
for (int i=0;i<numberOfItems;i++ ){
//Log.i("JSONItem",jsonArray.getString(i));
String articleId = jsonArray.getString(i);
url = new URL("https://hacker-news.firebaseio.com/v0/item/"+articleId+".json?print=pretty");
urlConnection = (HttpsURLConnection) url.openConnection();
in = urlConnection.getInputStream();
reader = new InputStreamReader(in);
data = reader.read();
String articleInfo = "";
while (data!= -1){
char current = (char) data;
articleInfo += current;
data = reader.read();
}
//Log.i("ArticleInfo",articleInfo);
//separate title and URL
JSONObject jsonObject = new JSONObject(articleInfo);
if (!jsonObject.isNull("title") && !jsonObject.isNull("url")){
String articleTitle = jsonObject.getString("title");
String articleURL = jsonObject.getString("url");
//Log.i("info",articleTitle + articleURL);
url = new URL(articleURL);
xurlConnection = (HttpURLConnection) url.openConnection();
in = xurlConnection.getInputStream();
reader = new InputStreamReader(in);
data = reader.read();
String articleContent = "";
while (data!= -1){
char current = (char) data;
articleContent += current;
data = reader.read();
}
//Log.i("articleContent",articleContent);
String sql = "INSERT INTO articles(articleID,title,content) VALUES(? , ? , ?)";
SQLiteStatement statement = articleDB.compileStatement(sql);
statement.bindString(1,articleId);
statement.bindString(2,articleTitle);
statement.bindString(3,articleContent);
statement.execute();
}
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
}
<<<<<<<<<< END OF COMMENTED OUT CODE >>>>>>>>>> */
ContentValues cv = new ContentValues();
cv.put("articleID",1);
cv.put("title","Title");
cv.put("content","Some content");
articleDB.insert("articles",null,cv);
return null;
}
结果是 :-
删除注释掉的代码,最初会Caused by: java.lang.SecurityException: Permission denied (missing INTERNET permission?)
使用 API 24 之前的设备(为了方便不必请求运行时权限)并更改清单以包含<uses-permission android:name="android.permission.INTERNET"></uses-permission>
结果是
Caused by: java.lang.ClassCastException: com.android.okhttp.internal.huc.HttpURLConnectionImpl cannot be cast to javax.net.ssl.HttpsURLConnection
at aso.aso57271930listview.MainActivity$DownloadTask.doInBackground(MainActivity.java:117)
at aso.aso57271930listview.MainActivity$DownloadTask.doInBackground(MainActivity.java:67)
第 117 行是urlConnection = (HttpsURLConnection) url.openConnection();
在第 117 行添加断点并在调试模式下运行会导致:-
按以下方式添加行:-
protected String doInBackground(String... strings) {
String result = "";
URL url;
HttpsURLConnection urlConnection = null;
HttpURLConnection xurlConnection = null; //<<<<<<<<<< ADDED for stage 2 testing
然后更改为使用:-
if (!jsonObject.isNull("title") && !jsonObject.isNull("url")){
String articleTitle = jsonObject.getString("title");
String articleURL = jsonObject.getString("url");
//Log.i("info",articleTitle + articleURL);
url = new URL(articleURL);
//urlConnection = (HttpsURLConnection) url.openConnection(); //<<<<<<<<<< commented out
xurlConnection = (HttpURLConnection) url.openConnection(); //<<<<<<<<<< added to replace commented out line.
in = urlConnection.getInputStream();
reader = new InputStreamReader(in);
data = reader.read();
String articleContent = "";
while (data!= -1){
char current = (char) data;
articleContent += current;
data = reader.read();
}
//Log.i("articleContent",articleContent);
String sql = "INSERT INTO articles(articleID,title,content) VALUES(? , ? , ?)";
SQLiteStatement statement = articleDB.compileStatement(sql);
statement.bindString(1,articleId);
statement.bindString(2,articleTitle);
statement.bindString(3,articleContent);
statement.execute();
}
结果是 :-
您的表未在 db 中创建,请更改
articleDB.execSQL("CREATE TABLE IF NOT EXISTS articles (id INTEGER PRIMARY KEY, articleID INTEGER,title VARCHAR,content VARCHAR)");
到
articleDB.execSQL("CREATE TABLE IF NOT EXISTS articles (id INTEGER PRIMARY KEY, articleID INTEGER,title TEXT,content TEXT)");
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.