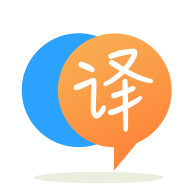
[英]abc.filter().map() ==> to reduce() How should I use it? JavaScript
[英]How to achieve map functionality with the use of filter in JavaScript, as we can use reduce as map and filter?
假设我有一个数组var arr = [1,2,3]
并且如果我做var result = arr.filter(callback)
我希望result
值将是[2,4,6]
通过使用过滤器。 我只想定义回调函数才能这样做。 使用地图可以轻松完成,但我只想使用过滤器。
Array.prototype.filter
仅过滤数组中的现有值,有效地创建一个新数组,该数组可以根据过滤函数保存相同的值、它们的子集或空数组。
您可以使用filter()
来做到这一点:
const arr = [1, 2, 3]; arr.filter((c, i) => { arr[i] = +arr[i] * 2; return true; }); console.log(arr)
我们总是返回true
,所以filter()
在这种情况下没有意义。
正如多次所述,您没有理由应该这样做。
不可能像 map 那样做,因为 map 返回一个新数组。 您可以更改原始数组,也可以复制原始数组,以免更改它。
// this modifies the orginal array var arr1 = [1,2,3] arr1.filter((v,index,arr)=>{ arr[index] = v * 2; return true }) console.log(arr1) // or you can clone it and do the same thing // this modifies the cloned array var arr2 = [1,2,3] var arr3 = arr2.slice() arr3.filter((v,index,arr)=>{ arr[index] = v * 2; return true }) console.log(arr2, arr3)
所以不,你不能用过滤器重新创建地图,因为你必须修改原始数组或欺骗并使用数组的副本。
所以我不确定我是否理解你问题的第二部分,但至于第一部分:
filter
的callback
有三个参数,其中两个是可选的。 第一个参数是遍历中的当前元素,第二个和第三个参数(可选的)是当前元素的从 0 开始的索引,以及对原始数组的引用。 这第三个参数对于您要执行的操作很有用。
let myArr = [1, 2, 3]; myArr.filter((el, ind, orig) => { orig[ind] = orig[ind] + 1; // Or, whatever map logic you want to use return true; // since a Boolean condition must be returned here });
这样你甚至不需要打破函数的作用域就可以做到!
如果你想这样做而不必有一个变量来最初调用filter
(你必须传递一个数组),你可以使用原型和call
方法:
Array.prototype.filter.call([1, 2, 3], (el, ind, orig) => { orig[ind] = orig[ind] + 1; return true; });
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.