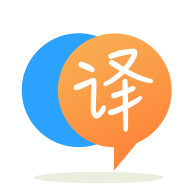
[英]compare to object based on one object key and other object value and push result to new array using map function?
[英]Return one array object instead all the object based on the condition using map function
我想基于ReactJS中的条件使用map函数从数组中获得一个索引值。
目前,如果条件通过,我将获得所有索引值,但是一旦条件为真,我想停止该条件。 这样我在返回时将索引一个...
JSON对象:
question: [
{
'question_type': 'TEXT',
'question': 'how long have the founders known each other?',
'question_status': 'Done',
'expectedAnswer': "",
'answer': '5 yrs'
},
{
'question_type': 'EITHER',
'question': 'does any founder have an exit?',
'question_status': 'Pending',
'expectedAnswer':['Yes','No'],
'answer': "",
},
{
'question_type': 'DROPDOWN',
'question': 'Where are you from?',
'question_status': 'Pending',
'expectedAnswer':['india','USA','UK'],
'answer': "",
},
]
在做过滤器的React功能
const currentQuestion = question.map(function (data, i) {
if (data.question_status === 'Pending') {
return (
<Row key={i} className='chat-box'>
<Col xl='1' lg='1' md='1' className="question-image">
<img src='images/chatbot/rectangle-26.png' />
</Col>
<Col xl='11' lg='11' md='11' className="question-text">
{data.question}
</Col>
<Col xl="11" lg="11" md="11" className="answer-box ">
{/* <img className="answer-image pull-right" src='images/chatbot/group-9.png' /> */}
<div className="pull-right custome-radio">
{data.question_type === 'EITHER'? this.renderEitherField(data.expectedAnswer):null}
</div>
</Col>
</Row>
);
}
}.bind(this));
预期产量:
{
'question_type': 'EITHER',
'question': 'does any founder have an exit?',
'question_status': 'Pending',
'expectedAnswer':['Yes','No'],
'answer': "",
}
我想仅过滤第一个索引值,而不是第一个和第二个。 目前,我正在获取第一和第二索引值,因为两个question_status均为“ Pending”,但是在这里我只想获取第一索引值(一旦第一次出现,它必须停止返回值)
如果这不是最好的方法,请告诉我。
您可以使用Array.find方法。 它从满足条件(未定义)的数组中返回第一个值。
const data = [ { 'question_type': 'TEXT', 'question': 'how long have the founders known each other?', 'question_status': 'Done', 'expectedAnswer': "", 'answer': '5 yrs' }, { 'question_type': 'EITHER', 'question': 'does any founder have an exit?', 'question_status': 'Pending', 'expectedAnswer':['Yes','No'], 'answer': "", }, { 'question_type': 'DROPDOWN', 'question': 'Where are you from?', 'question_status': 'Pending', 'expectedAnswer':['india','USA','UK'], 'answer': "", }, ] const out = data.find(x => x.question_status === 'Pending') console.log(out)
请记住,IE不支持
Array.find
。 您需要使用Polyfill。另一种方法可以是返回已过滤数组的第0个索引。 如果存在,它将返回该值,否则未定义。
您可以使用for循环。
let arr = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (let a of arr) {
console.log(a);
if (a === 5) {
break;
}
}
您可以将此示例用作满足您条件的参考。
您可以使用question_status
使用filter
功能过滤出结果
const question = [
{
'question_type': 'TEXT',
'question': 'how long have the founders known each other?',
'question_status': 'Done',
'expectedAnswer': "",
'answer': '5 yrs'
},
{
'question_type': 'EITHER',
'question': 'does any founder have an exit?',
'question_status': 'Pending',
'expectedAnswer':['Yes','No'],
'answer': "",
},
{
'question_type': 'DROPDOWN',
'question': 'Where are you from?',
'question_status': 'Pending',
'expectedAnswer':['india','USA','UK'],
'answer': "",
},
]
const arr = question.filter(q => q.question_status === 'Pending')
console.log(arr);
在上面的代码中,输出将如下所示,
[ { question_type: 'EITHER',
question: 'does any founder have an exit?',
question_status: 'Pending',
expectedAnswer: [ 'Yes', 'No' ],
answer: '' },
{ question_type: 'DROPDOWN',
question: 'Where are you from?',
question_status: 'Pending',
expectedAnswer: [ 'india', 'USA', 'UK' ],
answer: '' } ]
因此,您可以简单地从输出中获取第一个元素。
for
声明它满足您的需求
const data = [
{
'question_type': 'TEXT',
'question': 'how long have the founders known each other?',
'question_status': 'Done',
'expectedAnswer': "",
'answer': '5 yrs'
},
{
'question_type': 'EITHER',
'question': 'does any founder have an exit?',
'question_status': 'Pending',
'expectedAnswer':['Yes','No'],
'answer': "",
},
{
'question_type': 'DROPDOWN',
'question': 'Where are you from?',
'question_status': 'Pending',
'expectedAnswer':['india','USA','UK'],
'answer': "",
},
]
function currentQuestion() {
for (var i=0; i<data.length; i++) {
if (data[i].question_status === "Pending") return data[i];
}
}
条件满足后,此处的声明将消失,该函数将为您提供所需的值
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.