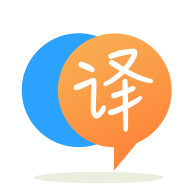
[英]SwiftUI NavigationLink: how to call a function before showing destination view
[英]SwiftUI NavigationLink loads destination view immediately, without clicking
使用以下代码:
struct HomeView: View {
var body: some View {
NavigationView {
List(dataTypes) { dataType in
NavigationLink(destination: AnotherView()) {
HomeViewRow(dataType: dataType)
}
}
}
}
}
奇怪的是,当HomeView
出现时, NavigationLink
立即加载AnotherView
。 结果,所有AnotherView
依赖项也被加载,即使它在屏幕上还不可见。 用户必须单击该行才能使其出现。 我的AnotherView
包含一个DataSource
,其中发生了各种事情。 问题是此时加载了整个DataSource
,包括一些计时器等。
难道我做错了什么..? 如何以这种方式处理它,一旦用户按下HomeViewRow
就会加载AnotherView
?
我发现解决这个问题的最好方法是使用惰性视图。
struct NavigationLazyView<Content: View>: View {
let build: () -> Content
init(_ build: @autoclosure @escaping () -> Content) {
self.build = build
}
var body: Content {
build()
}
}
然后 NavigationLink 将如下所示。 您可以将要显示的视图放在()
NavigationLink(destination: NavigationLazyView(DetailView(data: DataModel))) { Text("Item") }
编辑:请参阅@MwcsMac 的答案以获得更简洁的解决方案,该解决方案将视图创建包装在闭包中,并且仅在视图呈现后才对其进行初始化。
由于函数构建器确实必须评估表达式,因此需要自定义ForEach
来执行您的要求
NavigationLink(destination: AnotherView()) {
HomeViewRow(dataType: dataType)
}
为了能够显示HomeViewRow(dataType:)
每个可见行,在这种情况下AnotherView()
必须初始化AnotherView()
。
所以为了避免这种情况,自定义ForEach
是必要的。
import SwiftUI
struct LoadLaterView: View {
var body: some View {
HomeView()
}
}
struct DataType: Identifiable {
let id = UUID()
var i: Int
}
struct ForEachLazyNavigationLink<Data: RandomAccessCollection, Content: View, Destination: View>: View where Data.Element: Identifiable {
var data: Data
var destination: (Data.Element) -> (Destination)
var content: (Data.Element) -> (Content)
@State var selected: Data.Element? = nil
@State var active: Bool = false
var body: some View {
VStack{
NavigationLink(destination: {
VStack{
if self.selected != nil {
self.destination(self.selected!)
} else {
EmptyView()
}
}
}(), isActive: $active){
Text("Hidden navigation link")
.background(Color.orange)
.hidden()
}
List{
ForEach(data) { (element: Data.Element) in
Button(action: {
self.selected = element
self.active = true
}) { self.content(element) }
}
}
}
}
}
struct HomeView: View {
@State var dataTypes: [DataType] = {
return (0...99).map{
return DataType(i: $0)
}
}()
var body: some View {
NavigationView{
ForEachLazyNavigationLink(data: dataTypes, destination: {
return AnotherView(i: $0.i)
}, content: {
return HomeViewRow(dataType: $0)
})
}
}
}
struct HomeViewRow: View {
var dataType: DataType
var body: some View {
Text("Home View \(dataType.i)")
}
}
struct AnotherView: View {
init(i: Int) {
print("Init AnotherView \(i.description)")
self.i = i
}
var i: Int
var body: some View {
print("Loading AnotherView \(i.description)")
return Text("hello \(i.description)").onAppear {
print("onAppear AnotherView \(self.i.description)")
}
}
}
我遇到了同样的问题,我可能有一个包含 50 个项目的列表,然后为调用 API 的详细视图加载了 50 个视图(这导致下载了 50 个额外的图像)。
我的答案是使用 .onAppear 来触发所有需要在视图出现在屏幕上时执行的逻辑(比如关闭你的计时器)。
struct AnotherView: View {
var body: some View {
VStack{
Text("Hello World!")
}.onAppear {
print("I only printed when the view appeared")
// trigger whatever you need to here instead of on init
}
}
}
在目标视图中,您应该监听onAppear
事件并将所有需要在新屏幕出现时执行的代码放在那里。 像这样:
struct DestinationView: View {
var body: some View {
Text("Hello world!")
.onAppear {
// Do something important here, like fetching data from REST API
// This code will only be executed when the view appears
}
}
}
我最近在这个问题上苦苦挣扎(对于表单的导航行组件),这对我有用:
@State private var shouldShowDestination = false
NavigationLink(destination: DestinationView(), isActive: $shouldShowDestination) {
Button("More info") {
self.shouldShowDestination = true
}
}
只需用NavigationLink
包装一个Button
,该激活将通过该按钮进行控制。
现在,如果您要在同一个视图中有多个按钮+链接,而不是每个按钮的激活State
属性,您应该依赖这个初始化程序
/// Creates an instance that presents `destination` when `selection` is set
/// to `tag`.
public init<V>(destination: Destination, tag: V, selection: Binding<V?>, @ViewBuilder label: () -> Label) where V : Hashable
https://developer.apple.com/documentation/swiftui/navigationlink/3364637-init
沿着这个例子的思路:
struct ContentView: View {
@State private var selection: String? = nil
var body: some View {
NavigationView {
VStack {
NavigationLink(destination: Text("Second View"), tag: "Second", selection: $selection) {
Button("Tap to show second") {
self.selection = "Second"
}
}
NavigationLink(destination: Text("Third View"), tag: "Third", selection: $selection) {
Button("Tap to show third") {
self.selection = "Third"
}
}
}
.navigationBarTitle("Navigation")
}
}
}
从https://www.hackingwithswift.com/articles/216/complete-guide-to-navigationview-in-swiftui (在“程序化导航”下)获取的更多信息(以及上面稍微修改的示例)。
或者,创建一个自定义视图组件(带有嵌入式NavigationLink
),例如这个
struct FormNavigationRow<Destination: View>: View {
let title: String
let destination: Destination
var body: some View {
NavigationLink(destination: destination, isActive: $shouldShowDestination) {
Button(title) {
self.shouldShowDestination = true
}
}
}
// MARK: Private
@State private var shouldShowDestination = false
}
并将其作为Form
(或List
)的一部分重复使用:
Form {
FormNavigationRow(title: "One", destination: Text("1"))
FormNavigationRow(title: "Two", destination: Text("2"))
FormNavigationRow(title: "Three", destination: Text("3"))
}
对于 iOS 14 SwiftUI。
延迟导航目标加载的非优雅解决方案,使用视图修饰符,基于这篇文章。
extension View {
func navigate<Value, Destination: View>(
item: Binding<Value?>,
@ViewBuilder content: @escaping (Value) -> Destination
) -> some View {
return self.modifier(Navigator(item: item, content: content))
}
}
private struct Navigator<Value, Destination: View>: ViewModifier {
let item: Binding<Value?>
let content: (Value) -> Destination
public func body(content: Content) -> some View {
content
.background(
NavigationLink(
destination: { () -> AnyView in
if let value = self.item.wrappedValue {
return AnyView(self.content(value))
} else {
return AnyView(EmptyView())
}
}(),
isActive: Binding<Bool>(
get: { self.item.wrappedValue != nil },
set: { newValue in
if newValue == false {
self.item.wrappedValue = nil
}
}
),
label: EmptyView.init
)
)
}
}
像这样调用它:
struct ExampleView: View {
@State
private var date: Date? = nil
var body: some View {
VStack {
Text("Source view")
Button("Send", action: {
self.date = Date()
})
}
.navigate(
item: self.$date,
content: {
VStack {
Text("Destination view")
Text($0.debugDescription)
}
}
)
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.