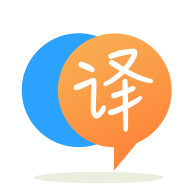
[英]Returning a value from within an if statement has a “mismatched types” error
[英]Why is there a mismatched types error when comparing a value after parsing it from a string?
我不明白为什么在成功解析后比较两个值时会出现类型不匹配错误。 我的大部分工作都是使用动态语言完成的,因此这可能会让我失望。 这会在其他语言(例如C ++或C#)中发生吗?
该代码无效。
use std::io;
fn main() {
let mut input_text = String::new();
io::stdin()
.read_line(&mut input_text)
.expect("Failed to read line");
let num_of_books = input_text.trim();
match num_of_books.parse::<u32>() {
Ok(i) => {
if num_of_books > 4 {
println!("Wow, you read a lot!");
} else {
println!("You're not an avid reader!");
}
}
Err(..) => println!("This was not an integer."),
};
}
error[E0308]: mismatched types
--> src/main.rs:12:31
|
12 | if num_of_books > 4 {
| ^ expected &str, found integer
|
= note: expected type `&str`
found type `{integer}`
虽然此代码有效。
use std::io;
fn main() {
let mut input_text = String::new();
io::stdin()
.read_line(&mut input_text)
.expect("Failed to read line");
let num_of_books = input_text.trim();
match num_of_books.parse::<u32>() {
Ok(i) => {
if num_of_books > "4" {
println!("Wow, you read a lot!");
} else {
println!("You're not an avid reader!");
}
}
Err(..) => println!("This was not an integer."),
};
}
您的问题实际上是由于在您的匹配分支中使用了错误的变量。 这将是任何静态类型语言的编译时错误。
当您在Ok(i)
上对匹配进行模式化时,您会说:“在Ok
内包装了一些变量-我将调用此变量i
并在此match分支作用域内对其执行某些操作。”
您想要的是:
use std::io;
fn main() {
let mut input_text = String::new();
io::stdin()
.read_line(&mut input_text)
.expect("Failed to read line");
let num_of_books = input_text.trim();
match num_of_books.parse::<u32>() {
Ok(i) => {
if i > 4 {
println!("Wow, you read a lot!");
} else {
println!("You're not an avid reader!");
}
}
Err(..) => println!("This was not an integer."),
};
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.