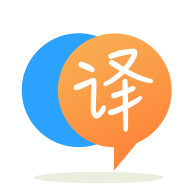
[英]Change action icon of playerNotificationManager like play/pause/stop
[英]How can I receive action of PlayerNotificationManager notification button like stop?
我使用 Exoplayer 并与 PlayerNotificationManager 绑定来处理玩家对通知的操作。 它工作得很好,但我想在通知中按下停止按钮时得到一个监听器或接收器。 现在,当我点击停止按钮时,播放器卡住了。
playerNotificationManager = PlayerNotificationManager.createWithNotificationChannel(
this,
"playback_channel",
R.string.exo_download_notification_channel_name,
1,
object : PlayerNotificationManager.MediaDescriptionAdapter {
override fun createCurrentContentIntent(player: Player?): PendingIntent? {
val intent = Intent(context, PlayerExoActivity::class.java)
return PendingIntent.getActivity(
context,
1,
intent,
PendingIntent.FLAG_UPDATE_CURRENT
)
}
override fun getCurrentContentText(player: Player?): String? {
return "Day " + chapterName
}
override fun getCurrentContentTitle(player: Player?): String {
return courseName!!
}
override fun getCurrentLargeIcon(
player: Player?,
callback: PlayerNotificationManager.BitmapCallback?
): Bitmap? {
return largeIcon
}
}
)
这是在 Exoplayer state 更改时处理其他事情的接收器。
override fun onPlayerStateChanged(playWhenReady: Boolean, playbackState: Int) {
if (playbackState == ExoPlayer.STATE_BUFFERING) {
val intent = Intent("com.example.exoplayer.PLAYER_STATUS")
intent.putExtra("state", PlaybackStateCompat.STATE_BUFFERING)
broadcaster?.sendBroadcast(intent)
} else if (playbackState == ExoPlayer.STATE_READY) {
val intent = Intent("com.example.exoplayer.PLAYER_STATUS")
if (playWhenReady) {
intent.putExtra("state", PlaybackStateCompat.STATE_PLAYING)
} else {
intent.putExtra("state", PlaybackStateCompat.STATE_PAUSED)
}
broadcaster?.sendBroadcast(intent)
} else if (playbackState == ExoPlayer.STATE_ENDED) {
val intent = Intent("com.example.exoplayer.PLAYER_STATUS")
intent.putExtra("state", PlaybackStateCompat.STATE_STOPPED)
broadcaster?.sendBroadcast(intent)
}
}
创建您自己的 BroadcastReceiver 实现:
class NotificationReceiver : BroadcastReceiver() {
companion object {
val intentFilter = IntentFilter().apply {
addAction(PlayerNotificationManager.ACTION_NEXT)
addAction(PlayerNotificationManager.ACTION_PREVIOUS)
addAction(PlayerNotificationManager.ACTION_PAUSE)
addAction(PlayerNotificationManager.ACTION_STOP)
}
}
override fun onReceive(context: Context?, intent: Intent?) {
when (intent?.action) {
PlayerNotificationManager.ACTION_NEXT -> {
}
PlayerNotificationManager.ACTION_PREVIOUS -> {
}
PlayerNotificationManager.ACTION_PAUSE -> {
}
PlayerNotificationManager.ACTION_STOP -> {
//do what you want here!!!
}
}
}
}
然后注册:
registerReceiver(notificationReceiver, NotificationReceiver.intentFilter)
不要忘记注销它(避免 memory 泄漏):
unregisterReceiver(notificationReceiver)
回答有点晚了,但也许它会对某人有所帮助。
您可以为您的 playerNotificationManager 设置自定义 ControlDispatcher。 使用 ControlDispatcher,您可以控制许多通知按钮。
playerNotificationManager.setUseStopAction(true)
playerNotificationManager.setControlDispatcher(object : DefaultControlDispatcher() {
override fun dispatchStop(player: Player, reset: Boolean): Boolean {
//Do whatever you want on stop button click.
Log.e("AudioPlayerService","Notification stop button clicked")
return true
}
})
首先在你的 onCreate()
@Override
protected void onCreate(Bundle savedInstanceState) {
...
createPlayerNotificationManager();
....}
createPlayerNotificationManager()
private void createPlayerNotificationManager() {
playerNotificationManager = new PlayerNotificationManager.Builder(this, 151, "1234")
.setMediaDescriptionAdapter(new PlayerNotificationManager.MediaDescriptionAdapter() {
@Override
public CharSequence getCurrentContentTitle(Player player) {
return videoTitle; // your video title
}
@Nullable
@Override
public PendingIntent createCurrentContentIntent(Player player) {
return null;
}
@Nullable
@Override
public CharSequence getCurrentContentText(Player player) {
return null;
}
@Nullable
@Override
public Bitmap getCurrentLargeIcon(Player player, PlayerNotificationManager.BitmapCallback callback) {
if (!VideoPlayerActivity.this.isDestroyed()) {
Glide.with(VideoPlayerActivity.this)
.asBitmap()
.load(new File(mVideoFiles.get(position).getPath()))
.into(new CustomTarget<Bitmap>() {
@Override
public void onResourceReady(@NonNull Bitmap resource, @Nullable Transition<? super Bitmap> transition) {
}
@Override
public void onLoadCleared(@Nullable Drawable placeholder) {
}
});
}
return null;
}
}).build();
playerNotificationManager.setColor(ResourcesCompat.getColor(this.getResources(), R.color.light_red, null));
playerNotificationManager.setColorized(true);
playerNotificationManager.setDefaults(151);
playerNotificationManager.setUseFastForwardAction(false);
playerNotificationManager.setUsePreviousAction(true);
playerNotificationManager.setUseNextAction(true);
playerNotificationManager.setVisibility(NotificationCompat.VISIBILITY_PUBLIC);
playerNotificationManager.setUseRewindAction(false);
playerNotificationManager.setPriority(NotificationCompat.PRIORITY_HIGH);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
playerNotificationManager.setBadgeIconType(NotificationCompat.BADGE_ICON_LARGE);
}
playerNotificationManager.setSmallIcon(R.drawable.dropdown_icon);
}
然后在 initPlayer()
playerNotificationManager.setPlayer(player);
在 releasePlayer()
playerNotificationManager.setPlayer(null);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.