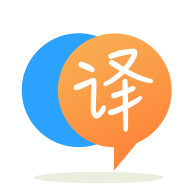
[英]How to save the count on a counter app and present that count in a list showing previous counts made? (using react)
[英]Reset count function in counter app not setting count to 0
我在计数器应用程序中将计数重置为 0 时遇到问题。 它不会将任何计数器的计数重置为 0,我已经尝试过console.log
每个 counter.count 但它显示undefined
。
App.js
:
import React from "react";
import "./App.css";
import Counter from "./components/Counter";
export class App extends React.Component {
state = {
counters: []
};
addCounter = () => {
this.setState({
counters: [...this.state.counters, Counter]
});
};
reset = () => {
const counters = this.state.counters.map(counter => {
counter.count = 0;
return counter;
});
// console.log(counters.count);
this.setState({ counters });
};
render() {
return (
<div className="App">
<button onClick={this.reset}>Reset</button>
<button onClick={this.addCounter}>Add Counter</button>
{this.state.counters.map((Counter, index) => (
<Counter key={index} />
))}
</div>
);
}
}
export default App;
Counter.js
:
import React, { Component } from 'react'
export class Counter extends Component {
state={
count:0,
}
increment=()=>{
this.setState({ count: this.state.count + 1 });
}
decrement=()=>{
this.setState({ count: this.state.count - 1 });
}
render() {
return (
<div>
<span><button onClick={this.increment}>+</button></span>
<span>{this.state.count}</span>
<span><button onClick={this.decrement}>-</button></span>
</div>
)
}
}
export default Counter
重置计数器应将所有计数器的计数重置为 0。
当你使用
this.setState({
counters: [...this.state.counters, Counter]
});
您将React Class
保存到counters
中。 因此,当您将 map 重置为 0 时,您需要New Class
来获取state.count
,如下所示:
reset = () => {
const counters = this.state.counters.map(counter => {
(new counter()).state.count = 0;
return counter;
});
for (let counter of counters) {
console.log((new counter()).state);
}
this.setState({ counters });
};
是时候抬起 state 了。
将所有increment
、 decrement
和reset
函数移至父App
。
此外,添加一个单独的阵列count
state 以监控每个计数器的当前计数。
// App
export class App extends React.Component {
state = {
counters: [],
count: [] // additional state to store count for each counter
};
addCounter = () => {
this.setState({
counters: [...this.state.counters, Counter],
count: [...this.state.count, 0]
});
};
reset = () => {
this.setState({
count: this.state.count.map(c => 0)
});
};
// lifted up from Counter
increment = index => {
this.setState({
count: this.state.count.map((c, i) => (i === index ? c + 1 : c))
});
};
// lifted up from Counter
decrement = index => {
this.setState({
count: this.state.count.map((c, i) => (i === index ? c - 1 : c))
});
};
render() {
return (
<div className="App">
<button onClick={this.addCounter}>Add Counter</button>
<button onClick={this.reset}>Reset</button>
{this.state.counters.map((Counter, index) => (
<Counter
key={index}
increment={() => this.increment(index)}
decrement={() => this.decrement(index)}
count={this.state.count[index]}
/>
))}
</div>
);
}
}
// Counter
export class Counter extends React.Component {
render() {
return (
<div>
<span>
<button onClick={this.props.increment}>+</button>
</span>
<span>{this.props.count}</span>
<span>
<button onClick={this.props.decrement}>-</button>
</span>
</div>
);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.