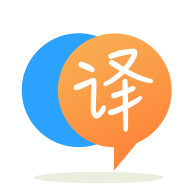
[英]Change label “username” to “account number” in WooCommerce registration
[英]Require user to have account number and username to change password
我希望用户输入他们的帐号和用户名,以确认他们的身份并更改密码。
当我只输入要确认的用户名但我似乎无法让 sql 中的额外注入工作时,该代码有效。
它只适用于一个但不能同时使用所需的帐号和用户名来确认他们的身份。
我希望看到最后能够将用户名的输入添加到表单中,并通过用户名和帐号进行确认。
<?php
// Initialize the session
session_start();
// Include config file
require_once "db.php";
// Define variables and initialize with empty values
$new_password = $confirm_password = $account = "";
$new_password_err = $confirm_password_err = $account_err = "";
// Processing form data when form is submitted
if($_SERVER["REQUEST_METHOD"] == "POST"){
// Check if account is empty
if(empty(trim($_POST["a"]))){
$account_err = "Please enter account number.";
} else{
$account = trim($_POST["a"]);
}
// Validate new password
if(empty(trim($_POST["new_password"]))){
$new_password_err = "Please enter the new password.";
} else if(strlen(trim($_POST["new_password"])) < 6){
$new_password_err = "Password must have atleast 6 characters.";
} else{
$new_password = trim($_POST["new_password"]);
}
// Validate confirm password
if(empty(trim($_POST["confirm_password"]))){
$confirm_password_err = "Please confirm the password.";
} else{
$confirm_password = trim($_POST["confirm_password"]);
if(empty($new_password_err) && ($new_password != $confirm_password)){
$confirm_password_err = "Password did not match.";
}
}
// Check input errors before updating the database
if(empty($account_err) && empty($new_password_err) && empty($confirm_password_err)){
// Prepare an update statement
$sql = "UPDATE vendors SET password = ? WHERE account = ?";
if($stmt = mysqli_prepare($link, $sql)){
// Bind variables to the prepared statement as parameters
mysqli_stmt_bind_param($stmt, "si", $param_password, $param_account);
// Set parameters
$param_password = password_hash($new_password, PASSWORD_DEFAULT);
$param_account = $account;
// Attempt to execute the prepared statement
if(mysqli_stmt_execute($stmt)){
// Store result
mysqli_stmt_store_result($stmt);
// Check if account exists, if yes then verify password
if(mysqli_stmt_num_rows($stmt) !== 1){
// Bind result variables
mysqli_stmt_bind_result($account);
// submited
session_start();
// Store data in session variables
$_SESSION["loggedin"] = true;
$_SESSION["id_users"] = $id_users;
$_SESSION["account"] = $account;
echo "you made it";
} else{
// Display an error message if password is not valid
$password_err = "The password you entered was not valid.";
}
}
} else{
// Display an error message if account doesn't exist
$account_err = "No account found with that Security Number.";
}
} else{
echo "Oops! Something went wrong. Please try again later.";
}
// Close statement
mysqli_stmt_close($stmt);
}
// Close connection
mysqli_close($link);
?>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.css" rel="stylesheet"/>
<div class="wrapper">
<h2>Reset Password</h2>
<p>Please fill out this form to reset your password.</p>
<form action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>" method="post">
<div class="form-group <?php echo (!empty($account_err)) ? 'has-error' : ''; ?>">
<label>Account Number</label>
<input type="text" name="a" class="form-control" value="<?php echo $account; ?>">
<span class="help-block"><?php echo $account_err; ?></span>
</div>
<div class="form-group <?php echo (!empty($new_password_err)) ? 'has-error' : ''; ?>">
<label>New Password</label>
<input type="password" name="new_password" class="form-control" value="<?php echo $new_password; ?>">
<span class="help-block"><?php echo $new_password_err; ?></span>
</div>
<div class="form-group <?php echo (!empty($confirm_password_err)) ? 'has-error' : ''; ?>">
<label>Confirm Password</label>
<input type="password" name="confirm_password" class="form-control">
<span class="help-block"><?php echo $confirm_password_err; ?></span>
</div>
<div class="form-group">
<input type="submit" class="btn btn-primary" value="Submit">
<a class="btn btn-link" href="welcome.php">Cancel</a>
</div>
</form>
</div>
您的表单不会同时要求提供用户名和帐号。 也不确定你在哪里打电话,看看它是否真实
你的 mysql 调用应该是
$sql = $dbh->prepare("SELECT * from user where userName = '$userName'
and accountNumber = '$accountNumber'");
$sql->execute();
if($sql->fetch){
//this is true
}
<?php
// Initialize the session
session_start();
// Include config file
require_once "db.php";
// Define variables and initialize with empty values
$new_password = $confirm_password = $account = "";
$new_password_err = $confirm_password_err = $account_err = "";
// Processing form data when form is submitted
if($_SERVER["REQUEST_METHOD"] == "POST"){
// Check if account is empty
if(empty(trim($_POST["a"]))){
$account_err = "Please enter account number.";
} else{
$account = trim($_POST["a"]);
}
// Validate new password
if(empty(trim($_POST["new_password"]))){
$new_password_err = "Please enter the new password.";
} else if(strlen(trim($_POST["new_password"])) < 6){
$new_password_err = "Password must have atleast 6 characters.";
} else{
$new_password = trim($_POST["new_password"]);
}
// Validate confirm password
if(empty(trim($_POST["confirm_password"]))){
$confirm_password_err = "Please confirm the password.";
} else{
$confirm_password = trim($_POST["confirm_password"]);
if(empty($new_password_err) && ($new_password != $confirm_password)){
$confirm_password_err = "Password did not match.";
}
}
// Check input errors before updating the database
if(empty($account_err) && empty($new_password_err) && empty($confirm_password_err)){
// Prepare an update statement
$sql = "UPDATE vendors SET password = ".$new_password." WHERE account = ".$account." ";
// $sql = "UPDATE vendors SET password = ".$new_password." WHERE account = ".$account." AND username = ".$username." ";
if($stmt = mysqli_prepare($link, $sql)){
// Bind variables to the prepared statement as parameters
mysqli_stmt_bind_param($stmt, "si", $param_password, $param_account);
// Set parameters
$param_password = password_hash($new_password, PASSWORD_DEFAULT);
$param_account = $account;
// Attempt to execute the prepared statement
if(mysqli_stmt_execute($stmt)){
// Store result
mysqli_stmt_store_result($stmt);
// Check if account exists, if yes then verify password
if(mysqli_stmt_num_rows($stmt) !== 1){
// Bind result variables
mysqli_stmt_bind_result($account);
// submited
session_start();
// Store data in session variables
$_SESSION["loggedin"] = true;
$_SESSION["id_users"] = $id_users;
$_SESSION["account"] = $account;
echo "you made it";
} else{
// Display an error message if password is not valid
$password_err = "The password you entered was not valid.";
}
}
} else{
// Display an error message if account doesn't exist
$account_err = "No account found with that Security Number.";
}
} else{
echo "Oops! Something went wrong. Please try again later.";
}
// Close statement
mysqli_stmt_close($stmt);
}
// Close connection
// mysqli_close($link);
?>
<!DOCTYPE html>
<html>
<head>
<title>Ajax Upload</title>
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
<!-- Popper JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
</head>
<body>
<div class="wrapper">
<h2>Reset Password</h2>
<p>Please fill out this form to reset your password.</p>
<form action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>" method="post">
<div class="form-group <?php echo (!empty($account_err)) ? 'has-error' : ''; ?>">
<label>Account Number</label>
<input type="text" name="a" class="form-control" value="<?php echo $account; ?>">
<span class="help-block"><?php echo $account_err; ?></span>
</div>
<div class="form-group <?php echo (!empty($new_password_err)) ? 'has-error' : ''; ?>">
<label>New Password</label>
<input type="password" name="new_password" class="form-control" value="<?php echo $new_password; ?>">
<span class="help-block"><?php echo $new_password_err; ?></span>
</div>
<div class="form-group <?php echo (!empty($confirm_password_err)) ? 'has-error' : ''; ?>">
<label>Confirm Password</label>
<input type="password" name="confirm_password" class="form-control">
<span class="help-block"><?php echo $confirm_password_err; ?></span>
</div>
<div class="form-group">
<input type="submit" class="btn btn-primary" value="Submit">
<a class="btn btn-link" href="welcome.php">Cancel</a>
</div>
</form>
</div>
</body>
</html>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.