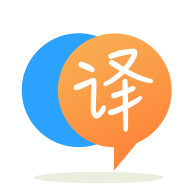
[英]JUnit Mockito always returns false in assertEquals when comparing boolean values
[英]Junit test always returns false
我有一项服务,它具有检查产品是否存在的方法。 如果找到则返回 true,否则返回 false。 但是当我创建一个单元测试 class 并调用该方法时,即使产品存在,它也总是返回 false。 当我使用 postman 运行应用程序和测试时,它按预期工作,当发现其他错误时返回 true。
服务类(当我通过控制器使用 postman 使用 api 时按预期工作)
@Service
@Transactional
public class ProductService {
private ProductRepository productRepository;
@Autowired
public ProductService(ProductRepository productRepository) {this.productRepository = productRepository;}
//check if a product exists
public boolean checkIfProductExists(String name)
{
Product product = productRepository.findByName(name);
if(product != null)
{
return true;
}
return false;
}
}
考试
@RunWith(SpringRunner.class)
public class ProductServiceTests {
@MockBean
private ProductService productService;
@Test
public void checkIfProductExistsTest()
{
//result is supposed to be true because ARVs exists
//when i consume api using postman it returns true, but here false
//why is it always false no matter the input
boolean result = productService.checkIfProductExists("ARVs");
//therefore test fails but it is supposed to pass
assertEquals(true,result);
}
}
您需要模拟productRepository
的响应
when(productRepository.findByName("ARVs")).thenReturn(myProduct);
Mocking ProductService
将始终导致false
。 您应该做的是 mocking ProductRepository
并将其注入ProductService
。 此外,如果您想重现“ARV”存在的情况,您应该指示您的模拟。
@RunWith(SpringRunner.class)
public class ProductServiceTests {
@MockBean
private ProductRepository productRepository;
@Autowired
private ProductService productService;
@Test
public void checkIfProductExistsTest()
{
Product myProduct = new Product();
Mockito.when(productRepository.findByName("ARVs")).thenReturn(myProduct);
boolean result = productService.checkIfProductExists("ARVs");
assertEquals(true,result);
}
}
如果你不告诉模拟的 object 如何表现,那么它总是会为你返回一个null
对象和一个false
boolean
变量。
您正在对ProductService
进行单元测试,并且您与 mocking 相同。 这不是单元测试的工作方式。
您模拟ProductService
的依赖关系,在这种情况下是ProductRepository
。
所以,你的测试应该看起来像
@RunWith(SpringRunner.class)
public class ProductServiceTest {
@MockBean
private ProductRepository repository;
@Autowired
private ProductService productService;
@Test
public void test() {
Mockito.when(repository.findProductByName(Mockito.anyString())).thenReturn(new Product());
boolean result = productService.checkIfProductExists("some product name");
assertEquals(true, result);
}
@TestConfiguration
static class TestConfig {
@Bean
public ProductService productService(final ProductRepository repository) {
return new ProductService(repository);
}
}
}
这种测试没有意义。 我相信你的意思是检查ProductService
的代码,但是你为它创建了一个 Mockito 的模拟,默认情况下,它会在调用带有任何参数的checkIfProductxists
时返回 false。
如果您的意图是检查ProductService
- 它不能是模拟的(使用@Autowired
而不是模拟 bean`)。 您可能需要考虑使用 mocking 存储库来避免数据库调用。
所以你应该@MockBean ProductRepository productRepository;
然后在上面注册期望:
@RunWith(SpringRunner.class) // you might need to supply some kind of context configuration here as well so that spring will know what beans should be really loaded
public class ProductServiceTest {
@Autowired ProductService productService;
@MockBean ProductRepository productRepo;
@Test
public void checkIfProductExistsTest() {
when(productRepo.findByName("ARVs")).thenReturn(<some_product_instance>);
boolean result = productService.checkIfProductExists("ARVs");
assertTrue(result);
}
}
我想你会为测试 class 寻找类似的东西:
@RunWith(SpringRunner.class)
public class ProductServiceTests {
@Autoired
private ProductService productService;
@MockBean ProductRepository productRepository;
@Test
public void checkTrueIfProductExists()
{
Product product = new Product();
Mockito.when(productRepository.findByName(Mockito.anyString())).thenReturn(product)
boolean result = productService.checkIfProductExists("foo");
assertTrue(result);
}
@Test
public void checkFalseIfProductDoesntExists()
{
Mockito.when(productRepository.findByName(Mockito.anyString())).thenReturn(null)
boolean result = productService.checkIfProductExists("bar");
assertFalse(result);
}
}
您之前将您的 ProductService 声明为模拟,实际上我们确实希望将其连接到然后模拟存储库。 然后,这允许我们使用 Mockito 之类的东西来控制存储库带回的结果。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.