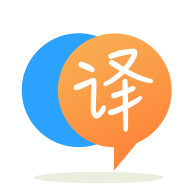
[英]How to pass test-case forcibly in Async await Node.js Unit Testing code using Chai and mocha
[英]Async await Node.js Unit Testing code using Chai and mocha
我对节点和快递很陌生。 并且一直在尝试用mocha、chai编写测试代码。 这是源代码的一部分。
googleService.js:
const axios = require('./axios');
exports.hitGoogle = async (requestUrl, payload) => {
let response = {};
await axios.post(`${requestUrl}`, payload)
.then((resp) => {
response = resp;
console.log(response);
})
.catch((err) => {
console.error(err);
});
return response;
};
阅读Google.js
const googleService = require('./googleService');
exports.execute = async (data) => {
console.log(`data ${JSON.stringify(data)}`);
console.log(`Name ${data.name}`);
console.log(`Salary ${data.salary}`);
console.log(`Age ${data.age}`);
const payload = { name: data.name, salary: data.salary, age: data.age };
await googleService.hitGoogle('http://dummy.restapiexample.com/api/v1/create', payload)
.then((response) => {
if (response.status === 200 || response.status === 201) {
console.log(response.status);
return true;
}
return false;
})
.catch((err) => {
console.log(err);
});
return true;
};
这是我的单元测试文件:
const readGoogle = require('./readGoogle');
const jmsPayload = { age: '23', name: 'test', salary: '123' };
describe('Google ', () => {
it('POST: Google.', async () => {
readGoogle.execute(jmsPayload).then((result) => {
results = result;
console.log(`Final Result : ${results.toString()}`);
});
});
当我执行这个测试文件时,发布成功,
hitGoogle url=http://dummy.restapiexample.com/api/v1/create
hitGoogle payload=[object Object]
{ status: 200,
statusText: 'OK',
从测试 class 传递到 readGoogle.js 的输入值也在其中读取,
data {"age":"23","name":"test","salary":"123"}
Name test
Salary 123
Age 23
**But I am getting Promise as output.**
**SO I have rendered the promised value to a normal string and getting 'true' as final output.**
还,
1)这是测试异步等待模块的正确方法吗?
2) 我们是否需要在此测试代码中添加任何其他内容,例如 Settimeout 或 beforeEach 或 afterEach 块?
3) 我们是否需要使用 Sinon 来监视或添加存根到 googleService.js 中的“有效负载”?
任何人都可以帮助或建议我正确的道路吗?
尝试了按如下顺序一一执行测试用例的异步方式,
const readGoogle = require('./readGoogle');
const jmsPayload = { age: '23', name: 'test', salary: '123' };
const common = require('./common');
const chaiHttp = common.chaiHttp;
common.chai.use(chaiHttp);
describe('Google ', () => {
common.mocha.before((done) => {
// Wait for the server to start up
setTimeout(() => {
done();
}, 1000);
});
it('POST: Google.', async () => {
const result = readGoogle.execute(jmsPayload);
console.log(`Final Result : ${result.toString()}`);
});
it('POST: Google.', async () => {
const result = readGoogle.execute(jmsPayload);
console.log(`Final Result : ${result.toString()}`);
setTimeout(() => {
}, 1000);
});
it('POST: Google.', async () => {
const result = readGoogle.execute(jmsPayload);
console.log(`Final Result : ${result.toString()}`);
});
it('POST: Google.', async () => {
const result = readGoogle.execute(jmsPayload);
console.log(`Final Result : ${result.toString()}`);
});
it('POST: Google.', async () => {
const result = readGoogle.execute(jmsPayload);
console.log(`Final Result : ${result.toString()}`);
});
it('POST: Google.', async () => {
const result = readGoogle.execute(jmsPayload);
console.log(`Final Result : ${result.toString()}`);
});
it('POST: Google.', async () => {
const result = readGoogle.execute(jmsPayload);
console.log(`Final Result : ${result.toString()}`);
});
});
但后来我得到,
错误:超过 2000 毫秒的超时。 对于异步测试和钩子,确保调用了“done()”; 如果返回 Promise,请确保它解析
提前致谢
您可以使用以下 2 个解决方案:
1)回调约定:
describe('Google ', () => {
it('POST: Google.', (done) => {
readGoogle.execute(jmsPayload).then((result) => {
results = result;
console.log(`Final Result : ${results.toString()}`);
done();
}).catch((e) => {
done();
});
});
});
2)异步/等待约定
describe('Google ', () => {
it('POST: Google.', async () => {
const results = await readGoogle.execute(jmsPayload);
console.log(`Final Result : ${results.toString()}`);
});
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.