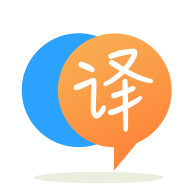
[英]Download contents of SQL Server table, and put the contents in to a CSV file?
[英]Is there a way to download a csv file of the data from a SQL Server database table on button click in Angular
I need to get all of the data in my SQL Server database table into a .csv
file when I click a button in a front end Angular web page.
I have already written a Web API in C# to access all the data from the SQL Server table and display it in my web page. 当我单击一个按钮时,我需要能够下载一个.csv
文件,该按钮包含我页面上显示的表格中的所有数据。
export class EmployeeService {
url = 'https://localhost:44395/Api/Employee';
constructor(private http: HttpClient) { }
getAllEmployee(): Observable<Employee[]> {
return this.http.get<Employee[]>(this.url + '/AllEmployeeDetails');
}
}
节省
Since you have to display the data in your angular application the best solution is to send the data as json and the use the following npm package: https://www.npmjs.com/package/xlsx to convert the json to xlsx file or csv
这是我为此编写的示例服务,只需创建此服务并在需要的地方调用 function:
excel.service.ts
import { Injectable } from '@angular/core';
import * as XLSX from 'xlsx';
@Injectable({
providedIn: 'root'
})
export class ExcelService {
constructor() { }
jsonToExcelSheet(data: any[], file_name = 'temp.xlsx') {
const workBook = XLSX.utils.book_new(); // create a new blank book
const workSheet = XLSX.utils.json_to_sheet(data);
let wscols = [{ wpx: 150 }, { wpx: 200 }, { wpx: 150 }, { wpx: 150 }];
workSheet['!cols'] = wscols; // set cell width
XLSX.utils.book_append_sheet(workBook, workSheet, 'data'); // add the worksheet to the book
return XLSX.writeFile(workBook, file_name); // initiate a file download in browser
}
jsonToCSV(data: any[], file_name = 'temp') {
const workBook = XLSX.utils.book_new(); // create a new blank book
const workSheet = XLSX.utils.json_to_sheet(data);
XLSX.utils.book_append_sheet(workBook, workSheet, 'data'); // add the worksheet to the book
return XLSX.writeFile(workBook, `${file_name}.csv`); // initiate a file download in browser
}
}
现在,如果您想使用此服务,只需将其导入所需的component.ts
import { ExcelService } from 'src/services/excel.service';
constructor(private _excelService: ExcelService){}
async downloadWorksheet() {
let downloadData = {} // load your data here
// export the json as excelsheet
await this._excelService.jsonToExcelSheet(downloadData);
}
您可以使用文件保护程序package 从 blob 下载文件。 加载数据并生成 CSV 字符串,该字符串可以转换为 Blob object。
npm i 文件保护程序
npm i @types/file-saver
app.component.ts :
import { saveAs } from 'file-saver'
download(){
// Variable to store data as CSV string
let csvStr = '';
// Fetch data from service
this.employeeService.getAllEmployee().subscribe(
response => {
// Manipulate data to generate CSV string
},error => {}
);
// Convert string to blob
var csvBlob = new Blob([csvStr], {
type: 'text/plain'
});
// Download
saveAs(csvBlob,'data.csv')
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.