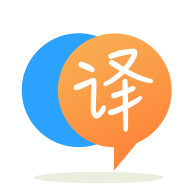
[英]Dynamically rendering objects from API in Javascript(object/API/dynamic rendering)
[英]problem with rendering an object property from API object
我已经在我的 Recipe 应用程序中请求 API 调用,并且可以访问我在首页中需要的所有数据,但是当我尝试从我的应用程序的另一个页面中的 API 调用中获取特定属性时,一旦我尝试渲染它。 我收到一条错误消息,告诉我“无法获取未定义或空引用的属性‘标签’”有人可以帮忙吗?
这是我的代码:
食谱应用
import React from "react";
import "bootstrap/dist/css/bootstrap.min.css";
import Form from "./components/form";
import Recipes from "./components/Recipes";
import "./App.css";
export default class RecipeApp extends React.Component {
state = {
recipes: []
};
getRecipe = async event => {
event.preventDefault();
const recipeName = event.target.recipeName.value;
const API_CALL = await fetch(
`https://api.edamam.com/search?q=${recipeName}&app_id=${API_ID}&app_key=${API_KEY}&from=0&to=10&calories=591-722&health=alcohol-free`
);
const data = await API_CALL.json();
this.setState({
recipes: data.hits
});
console.log(this.state.recipes);
};
render() {
return (
<div className="App">
<header>
<h1>Find Your Recipe</h1>
</header>
<Form getRecipe={this.getRecipe} />
<Recipes
recipes={this.state.recipes}
/>
</div>
);
}
}
路由器
import React from "react"
import { BrowserRouter, Switch, Route } from "react-router-dom";
import RecipeApp from "../recipe-app"
import Recipe from "./Recipe"
const Router = () => {
return (
<BrowserRouter>
<Switch>
<Route path="/" component={RecipeApp} exact/>
<Route path="/recipe/:label" component={Recipe} />
</Switch>
</BrowserRouter>
)
}
export default Router
食谱
import React from "react"
import { Link } from "react-router-dom"
const Recipes = ({ recipes }) => (
<div id="container">
<div className="row">
{recipes.map( (item) => {
return(
<div key={item.recipe.label} className="col-md-4" style={{marginBottom: "2rem"}}>
<div id="item-box">
<img
src={item.recipe.image}
alt={item.recipe.label} />
<div id="item-text">
<h3>{item.recipe.label.length > 20 ? `${item.recipe.label}` : `${item.recipe.label.substring(0, 25)}...`}</h3>
<p><i>publisher: {item.recipe.source}</i></p>
</div>
<button>
<Link
to={ {
pathname: `/recipe/${item.recipe.label}`,
state: { recipe: item.recipe.label }
} }>view recipe
</Link>
</button>
</div>
</div>
)
})}
</div>
</div>
)
export default Recipes;
食谱
import React from "react"
class Recipe extends React.Component {
state = {
activeRecipe: []
}
componentDidMount = async () => {
const title = this.props.location.state.recipe
const request = await fetch(
`https://api.edamam.com/search?q=${title}&app_id=${API_ID}&app_key=${API_KEY}&from=0&&calories=591-722&health=alcohol-free`
);
const response = await request.json();
this.setState({ activeRecipe: response.hits[0] })
}
render() {
const recipe = this.state.activeRecipe
console.log(recipe.recipe.label)
return(
<div></div>
)
}
}
export default Recipe
这是错误信息:
The above error occurred in the <Recipe> component:
in Recipe (created by Context.Consumer)
in Route (at Router.js:11)
in Switch (at Router.js:9)
in Router (created by BrowserRouter)
in BrowserRouter (at Router.js:8)
in Router (at src/index.js:7)
SCRIPT5007: SCRIPT5007: Unable to get property 'label' of undefined or null reference
main.chunk.js (115,5)
0: Unable to get property 'label' of undefined or null reference```
因为当组件启动时,recipes state 是 = []。 您调用服务器的 API 是异步的。 第一次渲染Component,这个API还没有给你返回数据,第二次,你就会得到这个数据。
在这里: {recipes.map( (item) => { })}
,recipes 在 Component 的第一次渲染中清空,所以当你访问item.recipe.label
时。 您将收到错误Unable to get property 'label' of undefined or null
。
要解决此问题,请确保食谱具有如下数据:
recipes.length > 0 && recipes.map( (item) => { ... })
或者您可以像这样使用默认参数:
{recipes.map( (item) => {
const { recipe = {} } = item;
// use default parameter here to make sure recipe always is an object
// then, use recipe.label will be no errors
return(
<div key={recipe.label} className="col-md-4" style={{marginBottom: "2rem"}}>
...
</div>
)
})}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.