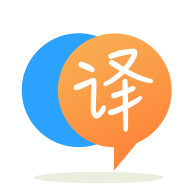
[英]Change JtextField depending on JcomboBox Selected using database data - Java
[英]Java: Using ArrayList with a JComboBox and JTextField
我目前正在尝试创建一个GUI,该GUI从包含汇率的.txt文件中获取信息。
文本文件包含3个不同的值,并用','分隔。
值是:货币名称,汇率,货币符号。
我正在运行程序,以便将这3个值存储到3个不同的ArrayLists中
该程序还将使用货币名称更新JComboBox。
但是,我现在需要该程序根据用户在JComboBox中选择的货币名称来选择正确的汇率和货币符号。
例如,如果用户选择USD(arrayCurrency)并将5输入到JTextField中,则程序需要选择正确的汇率(arrayRate)。
但是,我现在需要该程序根据用户在JComboBox中选择的货币名称来选择正确的汇率和货币符号。
这不完全是一个问题...我假设您要输入以下内容:
如何从JComboBox获取值? -> yourJCBox.getSelectedItem();
现在有了该字符串-您需要确定如何将汇率映射到美元金额。 无论是2d,3d阵列还是您设计的对象:
public class myCurrency() {
String currnecy;
String symbol;
Double rate;
public myCurrency(String c, String s, Double r) {
// Constructor stuff
}
// Getters and setters
}
现在,您有了所有需要检查的想法,以检查是否应显示某些汇率和符号的货币。
您可以通过以下方式轻松管理它:
Map
而不是List
下面是示例代码:
import java.util.HashMap;
import java.util.Map;
class Currency {
String code;
double convertionRate;
String symbol;
Currency(String code, double convertionRate, String symbol) {
this.code = code;
this.convertionRate = convertionRate;
this.symbol = symbol;
}
public double getConvertionRate() {
return convertionRate;
}
public void setConvertionRate(double convertionRate) {
this.convertionRate = convertionRate;
}
public String getSymbol() {
return symbol;
}
public void setSymbol(String symbol) {
this.symbol = symbol;
}
}
public class Main {
public static void main(String[] args) {
Map<String, Currency> currencyMap = new HashMap<String, Currency>();
// Populate currencyMap with values read from the .txt file e.g.
currencyMap.put("USD", new Currency("USD", 1.60, "$"));
// Replace the above line with currencyMap.put(currency_code-from-file,new
// Currency(currency_code-from-file,conversion_rate-from-file,currency_symbol-from-file));
// and use it inside the loop which you must be using to read the content from .txt file
// Getting the corresponding conversion rate and symbol for a currency e.g. USD
Currency currency = currencyMap.get("USD");
// Replace the above statement with Currency
// currency=currencyMap.get(String.valueOf(JComboBox.getSelectedItem()) in your
// program.
double convertionRate = currency.getConvertionRate();
String symbol = currency.getSymbol();
// Use convertionRate and symbol in your calculation
}
}
更新:由于您在评论中提到您难以将货币加载到currencyMap
,因此我添加了以下更新,只是为了演示如何将货币从.txt文件加载到currencyMap
:
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
class Currency {
String code;
double convertionRate;
String symbol;
Currency(String code, double convertionRate, String symbol) {
this.code = code;
this.convertionRate = convertionRate;
this.symbol = symbol;
}
public double getConvertionRate() {
return convertionRate;
}
public void setConvertionRate(double convertionRate) {
this.convertionRate = convertionRate;
}
public String getSymbol() {
return symbol;
}
public void setSymbol(String symbol) {
this.symbol = symbol;
}
}
public class Main {
Map<String, Currency> currencyMap = new HashMap<String, Currency>();
public static void main(String[] args) {
Main main = new Main();
main.populateCurrencyMap();
// Getting the corresponding conversion rate and symbol for a currency e.g. USD
Currency currency = main.currencyMap.get("USD");
if (currency != null)
System.out.println("Rate: " + currency.getConvertionRate() + ", Symbol: " + currency.getSymbol());
}
void populateCurrencyMap() {
File file = new File(
"/Users/arvind.avinash/Documents/workspace-spring-tool-suite-4-4.4.0.RELEASE/AdHoc/src/currency2.txt");
try {
BufferedReader in = new BufferedReader(new InputStreamReader(new FileInputStream(file), "UTF8"));
String line = in.readLine().trim();
while (line != null) {
String[] parts = line.split(",");
String currency = parts[0].trim();
double rate = Double.parseDouble(parts[1].trim());
String symbol = parts[2].trim();
currencyMap.put(currency, new Currency(currency, rate, symbol));
line = in.readLine();
}
in.close();
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
输出:
Rate: 1.6, Symbol: $
currency2.txt的内容:
USD, 1.60, $
EUR, 1.41, €
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.