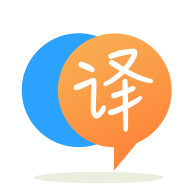
[英]passing pointer as argument in function (named: insertattail) still its not assignning the value
[英]Passing pointer to function and getting its value inside that function
下面的伪代码显示了我正在尝试实现的目标。 我是C ++和指针的新手,您可以从这个问题中猜到。 因此,我不知道该向函数[1]传递什么,从参数[2]中得到什么,以及用于进行比较的内容[3]。
请不要问为什么我传递一个指针而不是值本身,该函数仅接受此任务的指针。
我需要一些帮助。
main {
string someString = ..
function(pointer of the string above [1]*);
}
function (parameter[2]*) {
if (parameter[3]* == "Hello World") {
print("okay");
}
}
这取决于您使用的语言。
在C ++中,程序看起来像
#include <iostream>
#include <string>
void check( const std::string &s )
{
if ( s == "Hello World" )
{
std::cout << "okay" << '\n';;
}
}
int main()
{
std::string someString = "Hello World";
check( someString );
}
在C中,相应的程序看起来像
#include <stdio.h>
#include <string.h>
void check( const char *s )
{
if ( strcmp( s, "Hello World" ) == 0 )
{
puts( "okay" );
}
}
int main( void )
{
char someString[] = "Hello World";
check( someString );
}
也可以使用C ++编写使用字符数组的类似程序。
代替数组的声明
char someString[] = "Hello World";
您可以声明一个指向字符串文字的指针,例如
const char *someString = "Hello World";
您可以在此处选择两个选项:
通过引用传递变量,您将更改主字符串,除非您的函数接受指针为常量。 您可以通过执行以下操作来做到这一点:
main {
string someString = ...;
// Notice here we are passing the variable by reference
// We are passing the variable's address to the function
function(&somestring);
}
使用第二个选项,您可以执行以下操作:
main {
string* someString = new string(...);
// Pass it normally, as it will be accepted
function(someString);
}
在函数本身中,您可以按以下方式使用该值:
function(paramString) {
if(*paramString == "Hello World") {
do something..
}
}
请阅读有关函数参数和参数以及指针本身的更多信息。
以下链接将为您提供良好的开端。
https://www.w3schools.com/cpp/cpp_function_param.asp https://www.w3schools.com/cpp/cpp_pointers.asp
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.