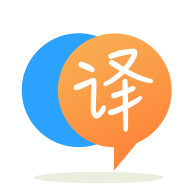
[英]How do I solve __WEBPACK_IMPORTED_MODULE_1__.default is undefined
[英]TypeError: react__WEBPACK_IMPORTED_MODULE_2___default(...) is not a function. How do I solve this?
当我收到以下错误时,我正在使用 React。
TypeError: react__WEBPACK_IMPORTED_MODULE_2___default(...) is not a function
我该如何解决这个问题? 我在下面添加了代码。
import React from 'react';
import LoginForm from './loginForm'
import useState from "react"
function LoginPage(){
const[details, setDetails] = useState({
username: '',
password: ''
});
function handleChange(event){
const updatedDetails = {...details, [event.target.name]: event.target.value};
setDetails(updatedDetails)
}
return(<LoginForm details={details} onChange={handleChange}/>)
};
export default LoginPage;
另一个组件是:-
import React from "react";
import 'bootstrap/dist/css/bootstrap.min.css';
import { Jumbotron, Container } from 'reactstrap';
import { Button, Form, FormGroup, Label, Input, FormText } from 'reactstrap';
function FormPage(props){
return (
<Jumbotron fluid>
<Container fluid>
<center><h1 className="display-3"> Log IN</h1></center>
<p className="lead">Please Enter your Details Here</p>
</Container><br />
<Container fluid>
<Form>
<FormGroup>
<Label for="username">Username</Label>
<Input type="textarea" name="username" id="username" placeholder="Enter your Username Here" value={props.details.username} onChange={props.onChange}></Input>
</FormGroup>
<FormGroup>
<Label for="password">Password</Label>
<Input type="password" name="password" id="password" placeholder="Enter your Password Here" value={props.details.username} onChange={props.onChange}></Input>
</FormGroup>
<Button color="success">Submit</Button>
</Form>
</Container>
</Jumbotron>
);
};
export default FormPage;
PS:我输入这个是因为 StackOverflow 要求我添加更多细节,因为我的问题主要是代码。 对不起。
我只是要指出你从“react”库导入两次
// You have: (look closely at libs you are importing from and how)
import React from 'react';
import LoginForm from './loginForm'
import useState from "react"
// Should be:
import React, { useState } from 'react';
import LoginForm from './loginForm'
// Why?
// Because useState is one of React libs' export default's apis / methods
// aka useState is just a part of the React default export but also is itself, an export
// React.useState() is how it would look if you just imported the React lib itself and nothing else
// how I personally handle any react apis is via ==>
import React, { useState } from 'react
// apart from loving seeing all libraries and individual apis imported
// as soon as I see a file to get a sense of its potential functionalities,
// read my detailed explanation below
在这里,您实际上是从整个 React 库中导入( export default react
)并简单地将其命名为随机字符串 useState,然后尝试使用它来使用真正的React.useState()
api/方法。
因此,您尝试以这种方式像实际的 React useState
api 一样使用 useState 绝对会导致错误,因为当您导入 react lib 的默认导入而不是作为其中一部分的 api 时,您基本上是在说这个require('react')()
该默认导出,或者它本身就是一个导出,您需要在导入语句中从 lib 解构,不确定是否相关,但您 100% 有格式错误的代码我不敢相信没有人提到这一点?
再举个例子,要让它像你一样工作(虽然 eslint 现在应该在你点击保存之前尖叫重复导入)你必须做useState.useState()
,这显然不是你想要的。 有些人不介意React.useState()
但我个人会避开你哈哈 jk,但从导入中破坏!!! 请 (:
出于所有原因,使用最佳编码标准对于成为团队中的优秀软件工程师甚至在您自己的个人项目中都是关键,并且绝对会增加您的 DX 和整体输出。
希望这对我的兄弟有所帮助。 我们都经历过这些小怪癖,一旦你学会了,把它添加到你的“我肯定知道的事情”列表中并继续运输
请添加更多信息。 就像您选择导入的 API/模块是什么以及它正在使用什么
export default function
对比
export function
你是怎么消费的?
您可能已将其导入为
import Something from './something';
而您的 something.js 可能看起来像:
export function Something(){
}
如果您将 webpack 更新到版本 5.xx,那么这可能与output.library 设置问题有关
因此,如果您在 webpack 输出中有库设置,请尝试将其删除:
output: {
path: path.resolve(__dirname, 'build'),
filename: 'painterro.commonjs2.js',
library: 'Painterro', // ⏪ this is the issue
libraryTarget: 'commonjs2',
},
将代码发布到引发此错误的文件中会很有用。 有了这些信息,您可能将某些内容作为默认导入导入,但没有从相关模块中导出为默认值。 尝试调查默认导出并查看其中一个是否没有默认导出。 您可以查看模块文档。
在类似的情况下,我在 Yarn 下运行 Next 时遇到上述错误。 当我添加一个新页面时,问题出现了。 唯一解决它的是运行yarn upgrade
。
导入 React, { Component } from "react"; 从'react-bootstrap'导入{按钮}; 从 './home' 导入历史记录 './home.css'
类首页扩展组件{渲染(){返回(
一个简单的应用程序显示反应按钮点击导航
<Button variant="btn btn-success" onClick={() => history.push('/Products')}>点击按钮查看产品); } }导出默认首页;
----它显示 TypeError: home__WEBPACK_IMPORTED_MODULE_2 _.default.push is not a function
我不知道这是否会对任何人有所帮助,但导致此错误的原因之一是您正在尝试初始化未定义的函数。 通常发生这种情况是因为您没有正确导入函数。 您可以尝试将函数作为 import {function} from "library" 而不是 import function from "library" 导入。 就我而言,发生错误是因为我从 next-cookies 导入了 getCookie 函数 - 从“cookies-next”导入 getCookie。 getCookie 不是导出默认函数。 我需要做的就是像导入它一样导入它 - import {getCookie} from 'cookies-next' 来解决问题。
安装新软件包后,您可能还没有重新运行该项目
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.