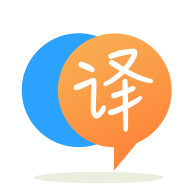
[英]How can I use a fake/mock/stub child component when testing a component that has ViewChildren in Angular 10+?
[英]Angular Unit Testing - Mock Child Component using Stub
我正在尝试对具有要模拟的子组件的组件进行单元测试。 我不想使用 NO_ERRORS_SCHEMA 导致相关的缺点。 我正在尝试使用存根/虚拟子组件。 代码如下:
父组件.ts
@Component({
selector: 'parent',
template: `
<!-- Does other things -->
<child></child>
`
})
export class ParentComponent { }
子组件.ts
@Component({
selector: 'child',
template: '<!-- Does something -->'
})
export class ChildComponent {
@Input() input1: string;
@Input() input2?: number;
}
parent.component.spec.ts
describe('ParentComponent', () => {
let component: ParentComponent;
let fixture: ComponentFixture<ParentComponent>;
let router: Router;
@Component({
selector: 'child',
template: '<div></div>'
})
class ChildStubComponent {
@Input() input1: string;
@Input() input2: number;
}
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ ParentComponent, ChildStubComponent ],
imports: [
AppRoutingModule, HttpClientModule, BrowserAnimationsModule,
RouterTestingModule.withRoutes(routes)
],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(ParentComponent);
component = fixture.componentInstance;
router = TestBed.get(Router);
spyOnProperty(router, 'url', 'get').and.returnValue('/somePath');
fixture.detectChanges();
});
it('should create', () => {
component.ngOnInit();
expect(component).toBeTruthy();
});
});
我遇到的问题是 angular 抱怨以下错误:
Failed: Template parse errors:
More than one component matched on this element.
Make sure that only one component's selector can match a given element.
如果有帮助,我正在使用 Angular 版本 8。 到目前为止,我所看到的所有地方都表明您以与我现在相同的方式存根子组件。 使用 ngMocks 目前不是一种选择。
编辑尝试使用 ngMock 但这只有同样的问题。 任何帮助将不胜感激!
解决方案
得到它的工作。 问题是 AppRoutingModule 已经导入了真正的组件。
对于嵌套组件,我们可以像这样模拟它们: https : //angular.io/guide/testing-components-scenarios#stubbing-unneeded-components
总之,你的parent.component.spec.ts应该是:
import { HttpClientTestingModule} from '@angular/common/http/testing';
import { Component } from '@angular/core';
describe('ParentComponent', () => {
let component: ParentComponent;
let fixture: ComponentFixture<ParentComponent>;
let router: Router;
@Component({selector: 'child', template: ''})
class ChildStubComponent {}
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ ParentComponent, ChildStubComponent ],
imports: [
AppRoutingModule, HttpClientTestingModule, BrowserAnimationsModule,
RouterTestingModule.withRoutes(routes)
],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(ParentComponent);
component = fixture.componentInstance;
router = TestBed.get(Router);
spyOnProperty(router, 'url', 'get').and.returnValue('/somePath');
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.