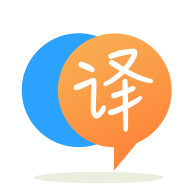
[英]Trying to build a reusable function to build dropdowns with JavaScript and JQuery
[英]Trying to build a recursion function using jQuery/JavaScript
我目前正在尝试构建一个函数,该函数遍历嵌套对象以查找匹配的值。 我创建了这个自定义代码来检查对象的每个级别。 问题如何多次重复该函数,直到它获取或匹配我正在寻找遍历多个级别的值。
let animalTree = { "animals":[ { "species":"Vertebrates", "types": [ { "category": "Fish", }, { "category": "Amphibians", }, { "category": "Reptiles", }, { "category": "Birds", }, { "category": "Mammals", } ] }, { "species":"Invertebrates", }, { "species":"Annelids", }, { "species":"Molluscs", }, { "species":"Nematodes", }, { "species":"Arthropods", }, ], } let scanTree = () => { let matchValue = "Vertebrates"; for (let i = 0; i < animalTree.length; i++){ if (animalTree[i].species == matchValue){ console.log('Found') } } } let jParse = JSON.stringify(animalTree); scanTree();
不确定您的键名可能是什么,因此使用Object.values()
提供通用函数 - 如果您只希望键名是物种,请告诉我 - 会简化
let animalTree = { "animals":[ { "species":"Vertebrates", "types": [ { "category": "Fish", }, { "category": "Amphibians", }, { "category": "Reptiles", }, { "category": "Birds", }, { "category": "Mammals", } ] }, { "species":"Invertebrates", }, { "species":"Annelids", }, { "species":"Molluscs", }, { "species":"Nematodes", }, { "species":"Arthropods", }, ], } let matchValue = "Birds"; let scan = (array) => { for(let item of array) { for(let value of Object.values(item)){ if(typeof value === "string" && value === matchValue) { return item; } else if (Array.isArray(value)) { return scan(value); } } } } let result = scan(animalTree.animals); console.log(result);
使用JSON.stringify()
和正则表达式来简化事情:
const scanTree = (str) => {
const arr = JSON.stringify(animalTree).match(/"\w+":\s?"\w+"/g);
const res = arr.filter((el) => el.indexOf(str) > -1);
return res.map(val => JSON.parse("{" + val + "}"));
}
const animalTree = { "animals":[ { "species":"Vertebrates", "types": [ { "category": "Fish", }, { "category": "Amphibians", }, { "category": "Reptiles", }, { "category": "Birds", }, { "category": "Mammals", } ] }, { "species":"Invertebrates", }, { "species":"Annelids", }, { "species":"Molluscs", }, { "species":"Nematodes", }, { "species":"Arthropods", }, ], }; const scanTree = (str) => { const arr = JSON.stringify(animalTree).match(/"\\w+":\\s?"\\w+"/g); const res = arr.filter((el) => el.indexOf(str) > -1); return res.map(val => JSON.parse("{" + val + "}")); } console.log(scanTree("Birds"));
let animalTree = {
"animals":[
{
"species":"Vertebrates",
"types": [
{
"category": "Fish",
},
{
"category": "Amphibians",
},
{
"category": "Reptiles",
},
{
"category": "Birds",
},
{
"category": "Mammals",
}
]
},
{
"species":"Invertebrates",
},
{
"species":"Annelids",
},
{
"species":"Molluscs",
},
{
"species":"Nematodes",
},
{
"species":"Arthropods",
},
],
}
let scanTree = () => {
var flag = 0;
let matchValue = "Vertebrates";
for(let i = 0 ; i < animalTree.animals.length ; i++ ){
if(animalTree.animals[i].species == matchValue){
flag = 1;
console.log("found");
break;
}
else{
flag = 0;
}
}
if(!flag){
console.log("not found")
}
}
//let jParse = JSON.stringify(animalTree);
scanTree();
试试这个。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.