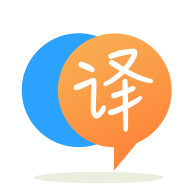
[英]Using Recursion in JavaScript to shift Array elements to different positions
[英]How to insert an element into all positions in an array using recursion?
我需要使用递归完成以下任务:
声明一个函数
insert_all_positions
,它接受参数:一个元素 x 和一个数组 arr。 函数必须返回一个数组数组,每个数组对应于 arrs,其中 x 插入到一个可能的位置。 也就是说,如果 arr 是长度 N,那么结果是一个包含 N + 1 个数组的数组。
比如insert_all_positions (10, [1,2,3])
就是数组:
[[10,1,2,3],
[1,10,2,3],
[1,2,10,3],
[1,2,3,10]]
到目前为止我有这个代码:
function insert_all_positions (x, arr) {
if (arr.length === 1) {
return arr.concat(x)
}
else {
}
}
这是一个纯粹的递归。
function f(x, A){ return A.length ? [[x, ...A]].concat( f(x, A.slice(1)).map(e => [A[0]].concat(e))) : [[x]] } var x = 10 var A = [1, 2, 3] console.log(JSON.stringify(f(x, A)))
您可以通过克隆使用递归,并在索引 ( i
) 位置添加x
元素,然后使用相同的参数调用函数并增加i
:
function insert_all_positions (x, arr, i = 0) { const clone = [...arr] // clone the array clone.splice(i, 0, x) // add the item at place i return i < clone.length - 1 ? // if still under the full length [clone, ...insert_all_positions(x, arr, i + 1)] // return current and next item/s : [clone] // return only current } const result = insert_all_positions (10, [1,2,3]) console.log(result)
为了在没有递归的情况下解决这个问题,我将使用Array.from()
,并创建一个长度为原始arr
length + 1 的数组:
function insert_all_positions (x, arr) { return Array.from({ length: arr.length + 1 }, (_, i) => [...arr.slice(0, i), x, ...arr.slice(i)] ); } const result = insert_all_positions (10, [1,2,3]) console.log(result)
您不必使用递归来执行此操作。 但是这个函数应该返回你正在寻找的东西:
function insert_all_positions (x, arr) {
const arrays = [];
for (let i = 0; i <= arr.length; i++) {
const value = [...arr.slice(0, i), x, ...arr.slice(i)];
arrays.push(value);
}
return arrays
}
您可以将结果添加为可以传入的参数。然后您将能够执行以下操作:
x
的新数组
x
的正确位置是结果的当前长度这一事实 - 首先它是0
,下次它是1
,等等。insert_all_position
提供新结果作为参数。这看起来像这样
//create an empty array for `result` initially function insert_all_positions (x, arr, result = []) { //terminate - we're done if (arr.length + 1 == result.length) return result; //create a new array from `arr` with `x` in its proper place const insertIndex = result.length; const newArray = arr .slice(0, insertIndex) .concat(x) .concat(arr.slice(insertIndex)); //construct the new result const newResult = [...result, newArray]; //recursive call return insert_all_positions(x, arr, newResult) } console.log(insert_all_positions(10, [1,2,3]))
纯递归。
递归从插入的值
10
作为空数组的最小数组。空数组是退出条件,如果数组中没有更多可用项,则仅返回带有插入值的嵌套数组。
对于每个递归步骤,使用递归调用的结果扩展具有所需值的数组,并且该数组被映射为插入值或为递归调用切割的数组的第一个值。
这里递归的结果是右下矩阵。
result comment ----------- ---------------------------------- 10 insert(10, []), no more recursions 10| 3 insert(10, [3]) --+-- 3|10 > result of the recursion 10| 2 3 insert(10, [2, 3]) --+----- 2|10 3 \\ result of the recursion 2| 3 10 / 10| 1 2 3 insert(10, [1, 2, 3]) --+-------- 1|10 2 3 \\ result of the recursion 1| 2 10 3 / 1| 2 3 10 /
function insert(x, array) { if (!array.length) return [[x]]; return [array, ...insert(x, array.slice(1))].map((a, i) => [i ? array[0] : x, ...a]); } insert(10, [1, 2, 3]).forEach(a => console.log(...a));
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.