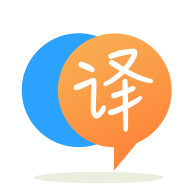
[英]How to subset an array using another one with boolean values using lodash
[英]How to swap array element from one position to another using lodash?
如何使用 lodash 库在 JavaScript 中将数组元素从一个位置交换到另一个位置? 像这样的东西:
_.swap(array, fromIndex, toIndex) //but this is not working
这是在线 lodash 测试器的链接,我在其中测试了一些方法,但都没有奏效
任何帮助将非常感激。 谢谢!
如果您只想交换数组中两个元素的索引位置,您可以使用原生 JavaScript 自己快速实现。 这是使用现代 ES6+ 语法的解决方案:
const swapArrayLocs = (arr, index1, index2) => {
[arr[index1], arr[index2]] = [arr[index2], arr[index1]]
}
如果你从未见过像我上面使用的那样的解构赋值,你可以在这里阅读它。 当您需要交换两个变量(或在这种情况下,两个数组索引)的值时,这是解决此类问题的一种特别有用的技术。
以防万一你需要支持像 Internet Explorer 这样的旧版浏览器,这里有一个 ES5 版本,它在语法上有点冗长:
var swapArrayLocs = function (arr, index1, index2) {
var temp = arr[index1];
arr[index1] = arr[index2];
arr[index2] = temp;
}
您还可以在任一方法中使用函数声明(而不是上面的函数表达式):
function swapArrayLocs(arr, index1, index2) {
var temp = arr[index1];
arr[index1] = arr[index2];
arr[index2] = temp;
}
上述所有用于实现您正在寻找的功能的方法都将以相同的方式使用 - 就像任何其他函数调用一样。 您将调用该函数,然后将要影响的数组以及要交换其值的两个数组索引传递给它。
const myArray = ["a", "b", "c", "d", "e", "f"];
swapArrayLocs(myArray, 0, 4);
// myArray is now: ["e", "b", "c", "d", "a", "f"]
这将操作数组,但我编写的函数不返回任何内容。 如果你想改变它,你可以在最后添加一个 return 语句来传回arr
或者可能是一个包含交换的两个元素的数组......无论你的特定用例需要什么。
由于 Array.splice 在新数组中返回删除的值,因此您可以将其写为:
const swapArrayLoc = (arr, from, to) => {
arr.splice(from, 1, arr.splice(to, 1, arr[from])[0])
}
使用临时变量。
const swapArrayLoc = (arr, from, to) => {
let temp = arr[to];
arr[to] = arr[from];
arr[from] = temp;
}
注意:这些方法会改变原数组,如果你不想改变它,复制到一个数组代替。
如果你想得到完整的数组结果......
const swapElementPosition = (arr: any[], indexFrom: number, indexTo: number) => {
const swappedIndices = [arr[indexFrom], arr[indexTo]] = [arr[indexTo], arr[indexFrom]]
arr.forEach((aV, aVIndex) => {
if (swappedIndices.indexOf(aV) === -1) {
swappedIndices[aVIndex] = aV;
}
})
return swappedIndices.filter((sI) => sI != null);
}
const a = new Date().toLocaleDateString().split('/').reverse();
const b = [12, 13, 14, 15, 16];
const aa = swapElementPosition(a, 2, 3);
const bb = swapElementPosition(b, 3, 4);
console.log(aa);
console.log(bb)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.