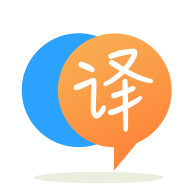
[英]C# LINQ Select objects with the same value of one property join values of other
[英]Assignment same property values to other
我有不同的类共享相同类型和名称的一些属性。 我希望为彼此分配相同的属性值。 我在以下伪代码的注释中更好地解释了我的意图。 在 C# 中可能吗?
想想有很多共同的属性,但在不相关的类中,我们是否必须一一赋值?
第二种情况是关于共享相同的属性,但其中一些可能可以为空,谁知道呢!
旁注:类已经存在,不能改变,不能触及。 有点sealed
。
不能使用nameof
运算符和两个 for 循环来完成吗? 如果匹配,比较属性名称,分配?
using System;
namespace MainProgram
{
class HomeFood
{
public DateTime Date { get; set; }
public string food1 { get; set; }
public string food2 { get; set; }
public int cucumberSize { get; set; }
}
class AuntFood
{
public string food2 { get; set; }
public int cucumberSize { get; set; }
public DateTime Date { get; set; }
public string food1 { get; set; }
// extra
public double? length { get; set; }
}
class GrandpaFood
{
public string? food2 { get; set; }
public int cucumberSize { get; set; }
public DateTime? Date { get; set; }
public string food1 { get; set; }
// extra
}
static class Program
{
public static void Main(string[] args)
{
var home = new HomeFood
{
Date = new DateTime(2020, 1, 1),
food1 = "cucumber",
food2 = "tomato",
cucumberSize = 123
};
var aunt = new AuntFood();
/*
First case: same types
Expected for-each loop
assigning a class's property values
to other class's property values
or for-loop no matter
foreach(var property in HomeFood's properties)
assign property's value to AuntFood's same property
*/
var home2 = new HomeFood();
var grandpa = new GrandpaFood
{
Date = new DateTime(2020, 1, 1),
food1 = "dfgf",
food2 = "dfgdgfdg",
cucumberSize = 43534
};
/*
Second case: similar to first case
with the exception of same type but nullable
or for-loop no matter
foreach(var property in GrandpaFood's properties)
assign property's value to GrandpaFood's same property
we don't care if it is null e.g.
Home2's same property = property's value ?? default;
*/
}
}
}
根据问题中的评论,这只是为了展示如何通过反思来完成。
免责声明,这只是关于如何使用反射同步属性的一个非常简化的示例。 它不处理任何特殊情况(修饰符、只读、类型不匹配等)
我强烈建议使用 automapper 来实现 qp 目标。
public class Type1
{
public string Property1 { get; set; }
public string Property2 { get; set; }
}
public class Type2
{
public string Property1 { get; set; }
public string Property3 { get; set; }
}
class Program
{
static void Main(string[] args)
{
var t1 = new Type1 { Property1 = "Banana" };
var t2 = new Type2();
var properties1 = typeof(Type1).GetProperties().ToList();
var properties2 = typeof(Type2).GetProperties().ToList();
foreach(var p in properties1)
{
var found = properties2.FirstOrDefault(i => i.Name == p.Name);
if(found != null)
{
found.SetValue(t2, p.GetValue(t1));
}
}
Console.WriteLine(t2.Property1);
}
}
简短的回答是,应用 OOP。 定义一个基本的Food
类,并在您拥有的任何特定食品类中从它继承。 您可以将所有共享道具放在基类中。
public class Food
{
public string food2 { get; set; }
// other shared stuff
}
class GrandpaFood : Food
{
// other specific stuff
}
正如其他人所说,使用一些面向对象的属性,例如继承实现接口的超类。
如果您要继承,请考虑使超类(您从中继承的)抽象。 这意味着超类本身不能被实例化,大大降低了违反里氏替换原则的风险。 也往往能更好地反映实际问题。 在您的示例中,情况也是如此,因为“食物”不是现实世界中的实际事物,而是一组事物。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.