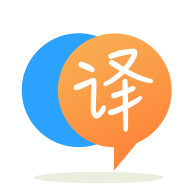
[英]Base class template instantiation depending on derived class constructor argument type
[英]Base constructor calls derived constructor depending on input - Choose object sub-type at runtime
假设我有一个 Human 类:
class Human {
public:
bool isFemale;
double height;
Human(bool isFemale, double height) {
this->isFemale = isFemale;
this->height = height;
}
};
和派生类,例如女性和男性,它们实现自己的方法。 在 C++11 中,我有没有办法在运行时根据人类构造函数的输入来确定人类应该是哪种“子类型”(男性或女性)? 我在他们各自的班级中为男性和女性设置了不同的行为。 我想要做的是在运行时确定 Human 是女性类型还是男性类型,具体取决于构造函数输入,因此我可以(之后)根据其类型应用适当的行为。 理想的情况是始终调用 Human 构造函数,并在运行时根据在构造函数中输入的参数选择适当的子类型。 如果可能的话,我想应该扭曲“人类”构造函数,但我不确定如何......
通过调用Human
的构造函数,您只能创建一个Human
对象。
据我了解,您希望根据在运行时获得的性别输入来创建Male
或Female
对象。 然后,您可能应该考虑使用工厂函数来实现这一点。
例如,如果您将Male
和Female
定义为:
struct Male: public Human {
Male(double height): Human(false, height) {}
// ...
};
struct Female: public Human {
Female(double height): Human(true, height) {}
// ...
};
然后,您可以使用以下工厂函数make_human()
:
std::unique_ptr<Human> make_human(bool isFemale, double height) {
if (isFemale)
return std::make_unique<Female>(height);
return std::make_unique<Male>(height);
}
它在运行时根据传递给isFemale
参数的参数决定是创建Female
还是Male
对象。
请记住将Human
的析构函数设为virtual
因为Male
和Female
类都从Human
公开继承。
请注意,您的设计允许使用isFemale = "true"
Male
,您可以在内部委托此操作避免错误标志,出于示例目的,您可以执行以下操作: Live Sample
#include <iostream>
class Human {
private:
int height;
protected:
bool isFemale;
public:
Human(){};
Human(int height, bool isFemale = false) : height(height) {}
virtual ~Human(){}
};
class Female : public Human {
public:
Female(int height) : Human(height, true) {
std::cout << "I'm a new Female, my height is " << height << std::endl;
}
};
class Male : public Human {
public:
Male(int height) : Human(height, false) {
std::cout << "I'm a new Male, my height is " << height << std::endl;
}
};
您可以调用适当的构造函数,例如:
int main() {
char gender;
Human h;
std::cout << " enter m/f: ";
std::cin >> gender;
switch(gender){
case 'm':
case 'M':
h = Male(186);
break;
case 'f':
case 'F':
h = Female(175);
break;
}
}
如果您想利用多态性,请查看此线程。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.