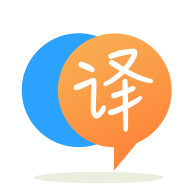
[英]How to fix this implicitly convert error in c# with if-else statement?
[英]C# - Convert Switch statement to If-Else
我在完成这项特定任务时遇到了一些麻烦。 获取 switch 语句并将其转换为 if-else。 该程序利用列表框来选择位置并显示相应的时区。
if (cityListBox.SelectedIndex != -1)
{
//Get the selected item.
city = cityListBox.SelectedItem.ToString();
// Determine the time zone.
switch (city)
{
case "Honolulu":
timeZoneLabel.Text = "Hawaii-Aleutian";
break;
case "San Francisco":
timeZoneLabel.Text = "Pacific";
break;
case "Denver":
timeZoneLabel.Text = "Mountain";
break;
case "Minneapolis":
timeZoneLabel.Text = "Central";
break;
case "New York":
timeZoneLabel.Text = "Eastern";
break;
}
}
else
{
// No city was selected.
MessageBox.Show("Select a city.");
通过这种方法,您可以摆脱switch
或if-else
语句
创建一个类来表示时区
public class MyTimezone
{
public string City { get; set; }
public string Name { get; set; }
}
创建时区列表并将其绑定到列表框
var timezones = new[]
{
new MyTimezone { City = "Honolulu", Name = "Hawaii-Aleutian" },
new MyTimezone { City = "San Francisco", Name = "Pacific" },
// and so on...
}
cityListBox.DisplayMember = "City";
cityListBox.ValueMember = "Name";
cityListBox.DataSource = timezones;
然后在要使用选定时区的代码中
var selected = (MyTimeZone)cityListBox.SelectedItem;
timeZoneLabel.Text = selected.Name;
因为Name
属性用作ValueMember
您可以使用SelectedValue
属性。
// SelectedValue can bu null if nothing selected
timeZoneLabel.Text = cityListBox.SelectedValue.ToString();
因此,在大多数编程语言中, switch
语句和if-else
语句几乎是相同的(一般来说;对于某些语言的某些编译器,switch 可能更快,尤其是我不确定 C#)。 Switch
或多或少是if-else
语法糖。 无论如何,与您的 switch 对应的 if-else 语句看起来像这样:
if (city == "Honolulu") {
timeZoneLabel.Text = "Hawaii-Aleutian";
} else if (city == "San Francisco") {
timeZoneLabel.Text = "Pacific";
} else if (city == "Denver") {
timeZoneLabel.Text = "Mountain";
}
... etc
那有意义吗?
我建议将switch
转换为Dictionary<string, string>
,即单独的数据(城市及其时区)和表示( Label
、 ListBox
等):
private static Dictionary<string, string> s_TimeZones = new Dictionary<string, string>() {
{"Honolulu", "Hawaii-Aleutian"},
{"San Francisco", "Pacific"},
//TODO: add all the pairs City - TimeZone here
};
那么您可以按如下方式使用它(两个if
s):
if (cityListBox.SelectedIndex >= 0) {
if (s_TimeZones.TryGetValue(cityListBox.SelectedItem.ToString(), out string tz))
timeZoneLabel.Text = tz;
else
timeZoneLabel.Text = "Unknown City";
}
else {
// No city was selected.
MessageBox.Show("Select a city.");
...
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.