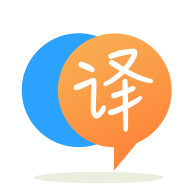
[英]converting a string of comma seperated values to a multidimensional javascript array?
[英]How to match array with comma seperated values in Javascript?
我有两个数组,一个用于类别,另一个用于产品。 产品以逗号分隔的字符串形式包含多个类别。 现在我想匹配一个特定的类别并将匹配的产品添加到每个类别的数组中。
我想将类别与 product_category 相匹配。
这是数组
产品
[
{
"id": 1,
"product_title": "Product 1",
"product_size": null,
"product_author": 5,
"description": "Test Description",
"product_category": "Shirts,Pents,Salwar",
"createdAt": "2020-02-08T11:42:15.000Z",
"updatedAt": "2020-02-08T11:42:15.000Z"
},
{
"id": 4,
"product_title": "Product 2",
"product_size": null,
"product_author": 5,
"description": "Test Description",
"product_category": "Pents,Salwar",
"createdAt": "2020-02-08T11:42:15.000Z",
"updatedAt": "2020-02-10T07:08:23.000Z"
}
]
类别
[
{
"id": 1,
"category_name": "Shirts",
"createdAt": "2020-02-08T04:59:59.000Z",
"updatedAt": "2020-02-10T06:50:05.000Z"
},
{
"id": 9,
"category_name": "Pents",
"createdAt": "2020-02-08T04:59:59.000Z",
"updatedAt": "2020-02-10T06:50:05.000Z"
}
]
我尝试但不起作用的代码
this.categories.forEach(cat => {
this.products.filter(prod => {
if (prod.product_category.split(',').indexOf(cat.category_name) !== -1) {
this.categories.push(prod);
}
});
});
请帮我解决这个问题。
任何解决方案表示赞赏!
您可以在categories
上使用.map()
为其添加products
属性。 此属性将是products
上.filter()
的结果。
const categoriesWithProducts = categories.map(cat => {
return {
...cat,
products: products.filter(prod => {
return prod.product_category.split(',').includes(cat.category_name);
})
};
});
``
你不需要filter()
您可以使用另一种forEach()
与includes()
和Object.assign()
var products = [ { "id": 1, "product_title": "Product 1", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Shirts,Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-08T11:42:15.000Z" }, { "id": 4, "product_title": "Product 2", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-10T07:08:23.000Z" } ]; var categories = [ { "id": 1, "category_name": "Shirts", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z" }, { "id": 9, "category_name": "Pents", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z" } ] categories.forEach((cat,i) => { products.forEach(prod => { if (prod.product_category.split(',').includes(cat.category_name)) { Object.assign(categories[i], prod); } }); }); console.log(categories);
其中一种方法是创建一个新数组,您可以在其中推送映射到特定类别的产品:
var products = [ { "id": 1, "product_title": "Product 1", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Shirts,Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-08T11:42:15.000Z" }, { "id": 4, "product_title": "Product 2", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-10T07:08:23.000Z" } ]; var categories = [ { "id": 1, "category_name": "Shirts", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z" }, { "id": 9, "category_name": "Pents", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z" } ] var productCategories = []; categories.forEach((cat)=> { var productCategory = {}; productCategory.category = cat; productCategory.products = []; products.forEach(prod => { if (prod.product_category.indexOf(cat.category_name) !== -1) { productCategory.products.push(prod); } }); productCategories.push(productCategory); console.log(productCategories); });
尝试这个
categories.forEach(function(c){
c.products=products.filter(function(p){
var reg=new RegExp(c.category_name,'gi');
return reg.test(p.product_category)
})
})
没有正则表达式
categories.forEach(function(c){
c.products=products.filter(function(p){
return (p.product_category.split(',').indexOf(c.category_name)!==-1)
})
})
您将获得每个category
的products
您可以轻松地遍历所有类别,然后遍历所有产品。 对于每个产品,检查类别名称是否存在,如果存在,则将产品添加到类别产品:
this.categories.forEach(cat => {
cat.products = [];
this.products.forEach(prod => {
if (prod.product_category.split(',').indexOf(cat.category_name) !== -1) {
cat.products.push(prod);
}
});
});
请注意,我将过滤器更改为 forEach。
您可以使用Array#filter
使用Array#some
过滤类别名称上的产品
let products = [{"id":1,"product_title":"Product 1","product_size":null,"product_author":5,"description":"Test Description","product_category":"Shirts,Pents,Salwar","createdAt":"2020-02-08T11:42:15.000Z","updatedAt":"2020-02-08T11:42:15.000Z"},{"id":4,"product_title":"Product 2","product_size":null,"product_author":5,"description":"Test Description","product_category":"Pents,Salwar","createdAt":"2020-02-08T11:42:15.000Z","updatedAt":"2020-02-10T07:08:23.000Z"}] let catagories = [{"id":1,"category_name":"Shirts","createdAt":"2020-02-08T04:59:59.000Z","updatedAt":"2020-02-10T06:50:05.000Z"},{"id":9,"category_name":"Pents","createdAt":"2020-02-08T04:59:59.000Z","updatedAt":"2020-02-10T06:50:05.000Z"}] let catagoryNames = new Set(catagories.map(e => e.category_name)); let filtered = [...products].filter(e => e.product_category.split(',').some(cat => catagoryNames.has(cat))); console.log(filtered)
试试这个代码。我希望它会帮助你。
product = [ { "id": 1, "product_title": "Product 1", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Shirts,Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-08T11:42:15.000Z" }, { "id": 4, "product_title": "Product 2", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-10T07:08:23.000Z" } ] categories=[ { "id": 1, "category_name": "Shirts", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z", }, { "id": 9, "category_name": "Pents", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z", } ] this.categories.forEach(cat => { cat['product'] = [] this.product.filter(prod => { if (prod.product_category.split(',').indexOf(cat.category_name) !== -1) { cat['product'].push(prod); } }); }); console.log(this.categories)
const products = [ { "id": 1, "product_title": "Product 1", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Shirts,Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-08T11:42:15.000Z" }, { "id": 4, "product_title": "Product 2", "product_size": null, "product_author": 5, "description": "Test Description", "product_category": "Pents,Salwar", "createdAt": "2020-02-08T11:42:15.000Z", "updatedAt": "2020-02-10T07:08:23.000Z" } ]; const categories = [ { "id": 1, "category_name": "Shirts", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z" }, { "id": 9, "category_name": "Pents", "createdAt": "2020-02-08T04:59:59.000Z", "updatedAt": "2020-02-10T06:50:05.000Z" } ]; const matchedProducts = []; products.map(prod=>{ const isCateoryMatched = categories.some(c=>{ return prod.product_category.includes(c.category_name) }); if(isCateoryMatched ){ matchedProducts.push(prod); } }) //if you want to push the matched products into categories categories.push(...matchedProducts) console.log('matchedProducts',matchedProducts); console.log('categories',categories);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.