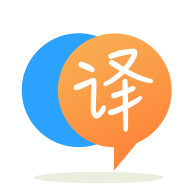
[英]How to improve performance of nested loops in javascript array manipulation?
[英]how to improve performance of nested for loops when comparing values in an array
var twoSum = function(nums, target) {
for(let i = 0; i < nums.length; i++){
for(let j = i + 1; j < nums.length; j++){
if(nums[i] + nums[j] === target){
return [nums.indexOf(nums[i]), nums.lastIndexOf(nums[j])]
}
}
}
}
function([3,5,3,7,2], 9) //It should return [3, 4] since 7 + 2 = 9
这是一个 Javascript 函数,它使用嵌套的 for 循环迭代数字数组,并找到一对,当求和时,它等于目标并返回它们的索引。 由于它有一个嵌套的 for 循环,我相信它具有 O(n^2) 或二次时间复杂度,我想知道是否有更快的方法来做到这一点。 我只是想获得第一次通过的解决方案,然后对其进行改进。 😁 提前致谢!
创建一个将每个元素映射到其最后一个索引的对象。 然后循环遍历每个元素,看看target - element
是否在具有不同索引的对象中。
function twoSums(nums, target) { const last_index = {}; nums.forEach((num, i) => last_index[num] = i); for (let i = 0; i < nums.length; i++) { const diff = target - nums[i]; if (diff in last_index && last_index[diff] != i) { return [i, last_index[diff]]; } } } const list = [3,5,3,7,2]; console.log(twoSums(list, 9)); console.log(twoSums(list, 6)); console.log(twoSums(list, 15));
查找一个对象的属性是 O(1),所以这个算法是 O(n)。
您可以使用嵌套map()
:
var twoSum = (nums, target)=> { // map trough list for first number nums.map( (val1, index1)=> { // map through list again for second number to compare to number returned from first map at each instance nums.map((val2, index2) => { // Ensure that index1 and index2 are not the same before you compare if(index1 != index2 && (val1 + val2 === target)) { alert([index1, index2]); return [index1, index2]; } }); } ); } twoSum([3,5,3,7,2], 9) //It should return [3, 4] since 7 + 2 = 9
注意:请记住,除非未找到匹配项,否则这不会遍历所有项目。 一旦找到匹配项,函数就会返回并退出。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.