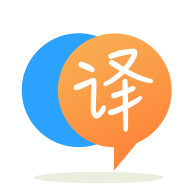
[英]Using jQuery or JavaScript to find multiples and remainder of 2 numbers
[英]Find consecutive numbers in multiples arrays JavaScript
我的数组结构如下:
[
[8,[1]],
[15,[2]],
[20,[3]],
[23,[4,41]],
[497,[18]],
[1335,[38]],
[2092,[39,55,61]],
[3615,[5]],
[4121,[14]],
[5706,[39,55,61]],
[5711,[62]],
[5714,[63]],
[5719,[64]],
[6364,[38]]
]
我使用此答案中修改后的代码来查找连续数字,但我无法对其进行调整以从具有多个值的数组中找到连续数字
这是我的代码:
const a = [
[8,[1]],
[15,[2]],
[20,[3]],
[23,[4,41]],
[497,[18]],
[1335,[38]],
[2092,[39,55,61]],
[3615,[5]],
[4121,[14]],
[5706,[39,55,61]],
[5711,[62]],
[5714,[63]],
[5719,[64]],
[6364,[38]]
];
// this variable will contain arrays
let finalArray = [];
// Create a recursive function
function checkPrevNextNumRec(array) {
let tempArr = [];
// if the array contaon only 1 element then push it in finalArray and
// return it
if (array.length === 1) {
finalArray.push(array);
return
}
// otherside check the difference between current & previous number
for (let i = 1; i < array.length; i++) {
if (array[i][1][0] - array[i - 1][1][0] === 1) {
// if current & previous number is 1,0 respectively
// then 0 will be pushed
tempArr.push(array[i - 1]);
} else {
// if current & previous number is 5,2 respectively
// then 2 will be pushed
tempArr.push(array[i - 1])
// create a an array and recall the same function
// example from [0, 1, 2, 5, 6, 9] after removing 0,1,2 it
// will create a new array [5,6,9]
let newArr = array.splice(i);
finalArray.push(tempArr);
checkPrevNextNumRec(newArr)
}
// for last element if it is not consecutive of
// previous number
if (i === array.length - 1) {
tempArr.push(array[i]);
finalArray.push(tempArr)
}
}
}
checkPrevNextNumRec(a)
这里的结果,如你所见,所有包含在[i][1][0]
中的连续数字的表格都被分组
[
[
[8,[1]],
[15,[2]],
[20,[3]],
[23,[4,41]]
],
[
[497,[18]]
],
[
[1335,[38]],
[2092, [39,55,61]]
],
[
[3615,[5]]
],
[
[4121,[14]]
],
[
[5706,[39,55,61]]
],
[
[5711,[62]],
[5714,[63]],
[5719,[64]]
],
[
[6364,[38]]
]
]
但我需要字段 5706 也包含在 5711、5714 和 5719 中,但显然它不包含在[i][1][0]
我想受到这篇文章的启发,但我无法正确集成它
你能帮助我吗?
谢谢!
您需要检查分组值的最后一个元素。
const array = [[8, [1]], [15, [2]], [20, [3]], [23, [4, 41]], [497, [18]], [1335, [38]], [2092, [39, 55, 61]], [3615, [5]], [4121, [14]], [5706, [39, 55, 61]], [5711, [62]], [5714, [63]], [5719, [64]], [6364, [38]]], grouped = array.reduce((r, a) => { var last = r[r.length - 1]; if (!last || last[last.length - 1][1][0] + 1 !== a[1][0]) r.push(last = []); last.push(a); return r; }, []); console.log(grouped);
.as-console-wrapper { max-height: 100% !important; top: 0; }
基本上,这里的逻辑是迭代input
并在每次迭代时将其与前一次迭代的数据值进行比较,即: prevData
。
在每次迭代中,我使用Array.prototype.map
创建一个新数组,该数组包含可能是连续的值(相对于前一次迭代中的数据),只需向prevData
每个项目添加 1。
下一步是循环该数组以查看是否遇到连续值 - 一旦我们这样做,我们就可以break
因为无需继续检查。
最后是我们使用单个if/else
应用分组逻辑。
const a = [ [8, [1]], [15, [2]], [20, [3]], [23, [4, 41]], [497, [18]], [1335, [38]], [2092, [39, 55, 61]], [3615, [5]], [4121, [14]], [5706, [39, 55, 61]], [5711, [62]], [5714, [63]], [5719, [64]], [6364, [38]] ]; function group(input) { let prev; return input.reduce((accum, el) => { let hasConsecutive = false; const [key, current] = el; if (prev == null) { prev = current; } const consecutives = prev.map(n => n + 1); for (let i = 0; i < consecutives.length; i += 1) { if (current.includes(consecutives[i])) { hasConsecutive = true; break; } } if (prev && hasConsecutive) { accum[accum.length - 1].push(el); } else { accum.push([el]); } prev = current; return accum; }, []); } console.log(group(a));
这是通过美化器运行的结果:
[
[
[8, [1]],
[15, [2]],
[20, [3]],
[23, [4, 41]]
],
[
[497, [18]]
],
[
[1335, [38]],
[2092, [39, 55, 61]]
],
[
[3615, [5]]
],
[
[4121, [14]]
],
[
[5706, [39, 55, 61]],
[5711, [62]],
[5714, [63]],
[5719, [64]]
],
[
[6364, [38]]
]
]
以下代码枚举数组中的每个项目。
对于每个项目,枚举其子数组以查看以下项目是否适合序列。
如果符合顺序,则将下一项附加到临时变量,然后我们继续下一项。
如果在没有检测到序列的延续的情况下到达子数组中的最后一项,我们结束当前序列,开始一个新序列并继续下一项。
const data = [ [8,[1]], [15,[2]], [20,[3]], [23,[4,41]], [497,[18]], [1335,[38]], [2092,[39,55,61]], [3615,[5]], [4121,[14]], [5706,[39,55,61]], [5711,[62]], [5714,[63]], [5719,[64]], [6364,[38]] ] function sequences(data) { const result = [] let sequence = [data[0]] for(let x=0; x<data.length-1; x++) { const [,sub] = data[x] for(let y=0; y<sub.length; y++) { const currSubV = sub[y] const [,nextSub] = data[x+1] if(nextSub.some((nextSubV) => currSubV+1 === nextSubV)) { sequence.push(data[x+1]) break } if(y === sub.length-1) { result.push(sequence) sequence = [data[x+1]] } } } return result } for(let s of sequences(data)) console.log(JSON.stringify(s).replace(/\\s/g))
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.