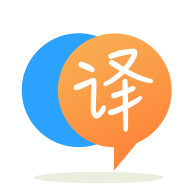
[英]Blazor WebAssembly - appsettings.json as dependency while registering services
[英]How to acess the appsettings in blazor webassembly
我正在尝试将 api url 保存在 appsettings 中。 但是, configuration.Propertiers 似乎是空的。 我不确定如何获得设置。 在 program.cs 中:
public static async Task Main(string[] args)
{
var builder = WebAssemblyHostBuilder.CreateDefault(args);
//string url = builder.Configuration.Properties["APIURL"].ToString();
foreach (var prop in builder.Configuration.Properties)
Console.WriteLine($"{prop.Key} : {prop.Value}" );
//builder.Services.AddSingleton<Service>(new Service(url));
builder.RootComponents.Add<App>("app");
await builder.Build().RunAsync();
}
当 blazor 尚不支持 wwwroot 文件夹中的 appsettings.json 时,此答案涉及 blazor 预览。 您现在应该在 wwroot 文件夹中使用 appsettings.json 和
WebAssemblyHostBuilder.Configuration
。 它还支持每个环境文件(appsettings.{env}.Json)。
我通过使用应用程序wwwroot文件夹中的settings.json文件存储解决了这个问题,并注册了一个任务来获取设置:
设置.cs
public class Settings
{
public string ApiUrl { get; set; }
}
wwwroot/settings.json
{
"ApiUrl": "https://localhost:51443/api"
}
程序.cs
public static async Task Main(string[] args)
{
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.Services.AddSingleton(async p =>
{
var httpClient = p.GetRequiredService<HttpClient>();
return await httpClient.GetJsonAsync<Settings>("settings.json")
.ConfigureAwait(false);
});
SampleComponent.razor
@inject Task<Settings> getsettingsTask
@inject HttpClient client
...
@code {
private async Task CallApi()
{
var settings = await getsettingsTask();
var response = await client.GetJsonAsync<SomeResult>(settings.ApiUrl);
}
}
这有以下优点:
Inkkiller 做到了。 您可以在没有 APIHelper 类的情况下简化对 IConfiguration 的调用,并从 WebAssemblyHostBuilder 直接在 Program.cs 中访问它。
应用设置:
{
"ServerlessBaseURI": "http://localhost:0000/",
}
程序.cs:
public static async Task Main(string[] args)
{
var builder = WebAssemblyHostBuilder.CreateDefault(args);
string serverlessBaseURI = builder.Configuration["ServerlessBaseURI"];
}
您也可以(wwwroot 中的 appsettings.json):
public class Program
{
public static async Task Main(string[] args)
{
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("app");
var url = builder.Configuration.GetValue<string>("ApiConfig:Url");
builder.Services.AddTransient(sp => new HttpClient { BaseAddress = new Uri(url) });
}
}
使用 ASP.NET Core 6.0 Blazor 配置。 Blazor WebAssembly 默认从以下应用设置文件加载配置:
例子:
wwwroot/appsettings.json
{
"h1FontSize": "50px"
}
Pages/ConfigurationExample.razor
@page "/configuration-example"
@using Microsoft.Extensions.Configuration
@inject IConfiguration Configuration
<h1 style="font-size:@Configuration["h1FontSize"]">
Configuration example
</h1>
警告 Blazor WebAssembly 应用中的配置和设置文件对用户可见。 不要将应用机密、凭据或任何其他敏感数据存储在 Blazor WebAssembly 应用的配置或文件中。
https://docs.microsoft.com/en-us/aspnet/core/blazor/fundamentals/configuration?view=aspnetcore-6.0
您还可以将值绑定到一个类。
public class ClientAppSettings
{
public string h1FontSize{ get; set; }
}
然后将此类添加为 Program.cs 中的 Singleton:
var settings = new ClientAppSettings();
builder.Configuration.Bind(settings);
builder.Services.AddSingleton(settings);
将命名空间添加到_Imports.razor
,然后在需要的地方注入以在 Visual Studio 中使用自动完成功能获取设置:
@inject ClientAppSettings ClientAppSettings
到目前为止,您可以使用IConfiguration
。
appsettings.json:
{
"Services": {
"apiURL": "https://localhost:11111/"
}
}
.
using Microsoft.Extensions.Configuration;
public class APIHelper
{
private string apiURL;
public APIHelper(IConfiguration config)
{
apiURL = config.GetSection("Services")["apiURL"];
//Other Stuff
}
}
Blazor WASM appsettings.json
如果wwwroot
文件夹中没有appsettings.json
,那么只需:
wwwroot
文件夹。这会将appsettings.json
添加到您的应用程序中。 打开appsettings.json
文件,您将在其中看到一个部分,用于数据库添加一个部分,就像我添加了apiinfo
:
{
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\MSSQLLocalDB;Database=_CHANGE_ME;Trusted_Connection=True;MultipleActiveResultSets=true"
},
"apiinfo":{
"apiurl": "your api url"
}
}
现在,当您想调用此部分时,只需注入配置并将其称为:
@inject Microsoft.Extensions.Configuration.IConfiguration config;
并调用apiurl
:
config.GetSection("apiinfo")["apiurl"].ToString()
作为一个例子,我有它像这样实现(客户端 Blazor):
appsettings.json:
{
"api": "https://www.webapiurl.com/"
"ForceHTTPS": false
}
然后,输入了配置类
public class APISetting
{
public string api { get; set; }
public bool ForceHTTPS { get; set; }
}
然后,在启动时加载:
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton(GetConfiguration());
}
public void Configure(IComponentsApplicationBuilder app )
{
app.AddComponent<App>("app");
}
public APISetting GetConfiguration()
{
using (var stream = System.Reflection.Assembly.GetExecutingAssembly().GetManifestResourceStream("appsettings.json"))
using (var reader = new System.IO.StreamReader(stream))
{
return System.Text.Json.JsonSerializer.Deserialize<APISetting>(reader.ReadToEnd());
}
}
}
同样在 .Net 5 & 6 中,您可以将值设置为静态类。
例子:
wwwroot/appsettings.json
"ServicesUrlOptions": {
"Url": "https://domain.gr/services" }
静态类
public static class ApplicationServicesSettings
{
public const string ServicesUrl = "ServicesUrlOptions";
public static ServicesUrlOptions ServicesUrlOptions { get; set; } = new ServicesUrlOptions();
}
public class ServicesUrlOptions
{
public string Url { get; set; }
}
最后绑定 Program.cs 的值
builder.Configuration.GetSection(ApplicationServicesSettings.ServicesUrl).Bind(ApplicationServicesSettings.ServicesUrlOptions);
在项目中之后,您可以通过以下方式访问密钥
ApplicationServicesSettings.ServicesUrlOptions.Url
创建设置类:
public class Settings
{
public string ApiUrl { get; set; }
}
在 wwwroot 文件夹中创建 settings.json:
{
"ApiUrl": "http://myapiurlhere"
}
并在 .razor 组件中像这样读取它:
@inject HttpClient Http
...
@code {
private string WebApuUrl = "";
protected override async Task OnInitializedAsync()
{
var response = await Http.GetFromJsonAsync<Settings>("settings.json");
WebApuUrl = response.ApiUrl;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.