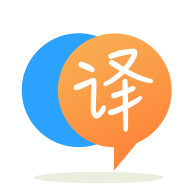
[英]FreeCodeCamp Javascript calculator test suite | I don't know why I can't pass this test
[英]Stuck on last two FreeCodeCamp Javascript Calculator tests on the test suite?
未通过的两个测试如下我无法弄清楚如何在代码中实现它并通过它们。
单击小数元素时,将出现一个“.”。 应该 append 到当前显示的值; 二 ”。” 一个数字不应该被接受
如果连续输入 2 个或更多运算符,则执行的运算应为最后输入的运算符(不包括负号 (-)。
到目前为止,我已经使用了 3 个回调函数,并且有一个计算器可以正常工作。 有任何想法吗? 提前致谢。 这是codepen链接:
https://codepen.io/nezmustafa123/pen/oNXwxmo javscript代码在这里。
//start with string
var tempMem = "";
const display = document.querySelector('#display');
document.querySelectorAll('[data-value]').forEach(el => {
el.onclick = e => {
if(display.innerText === "0") {
display.innerText = el.dataset.value
} else {
display.innerText += el.dataset.value;
}
}
})
document.querySelector('#equals').onclick = () => {
let result = eval(display.innerText);
display.innerText = result;
}
document.querySelector('#clear').onclick = () => {
display.innerText = 0;
}
可能为时已晚,但它对其他人有帮助。 我目前还不熟悉 JS DOM,因此我将使用简单的 JS 代码提供以下解决方案。
使用以下 function 处理输入,将多个 0 或小数减少为一个 0 或一位小数
const reduceMultipleZerosAndDecimalsToOne = (input) => {
const processedInput = [];
for (let i = 0; i < input.length; i++) {
const item = input[i];
if ((item.match(/[.]/g) || []).length > 1) {
// if item has multiple decimals between numbers 5.5.5
if (item.match(/^[0-9]\.[0-9]\.[0-9]$/)) {
const item2 = item.replace(/[.]/g, " ").trim().replace(/\s+/, "."); // replace multiple ... with one .
const item3 = item2.replace(/\s+/, ""); // replace remaning whitespace
// console.log(item3);
if (item3) {
processedInput.push(item3);
}
} else {
//if item has multiple decimals between numbers eg 5...55
// console.log(item);
const item2 = item.replace(/[.]/g, " ").trim().replace(/\s+/, "."); // replace multiple ... with one .
// console.log(item2);
if (item2) {
processedInput.push(item2);
}
}
} else if (item.match(/^0+/g)) {
const item2 = item.replace(/^0+/g, 0); // replace multiple 0ss before a number to one zero
if (item2) {
processedInput.push(Number(item2));
// console.log(item2);
}
} else if (Number(item) == 0) {
const item2 = Number(item); // convert multiple 0s to one 0
if (item2) {
processedInput.push(item2);
}
} else {
processedInput.push(item);
}
}
return processedInput;
};
在这里你也可以使用 function 使输入成为 -input 如果在 / 或 X 或 + 或 - 操作之后加上 - 符号。 请注意,我只在“5X-5”上测试了 function,因此请适当调整
let regex1;
let regex2;
let unWanted;
let wanted;
// a function to make input to be -X if preceded by - sign after /X+-
const correctFormatNegativeNumbers = (input, clickedMethods) => {
const regex1 = /[0-9],[\/|X|+|-],-,[0-9]/g; // test if input has negative number and is preceded with /X+-
const regex2 = /^(-,[0-9],[\/|X|+|-],[0-9])/g; // test if input has negative number and is followed with /X+-
const regex3 = /^(-,[0-9],[\/|X|+|-](.*?))/g; // test if input has a starting negative number and is followed with /X+- then anything
const regex4 = /((.*?)[\/|X|+|-],-,[0-9](.*?))/g; // test if input has negative number that is preceded with anyhting and /X+- and is followed with /X+-
if (regex3.test(input) || regex4.test(input)) {
const unWanted1 = "-,";
const wanted1 = "-";
const unWanted2 = ",-,";
const wanted2 = ",-";
const input2 = input
.slice()
.toString()
.replace(unWanted1, wanted1)
.replace(unWanted2, wanted2);
//drop - from methods
const newMethods = input2
.replace(/[0-9]|-[0-9]/g, "")
.replace(/,-,/g, ",")
.replace(/-,/g, "");
const processedItems = [input2.split(","), newMethods];
return processedItems;
// change -, input to -input
} else if (regex1.test(input)) {
console.log("Regex is regex1");
const unWanted = ",-,";
const wanted = ",-";
const input2 = input.slice().toString().replace(unWanted, wanted);
console.log(input2);
//drop - from methods
const newMethods = input2
.replace(/[0-9]|-[0-9]/g, "")
.replace(/,-,/g, ",")
.replace(/-,/g, "");
const processedItems = [input2.toString().split(","), newMethods];
return processedItems;
// change -, input to -input
} else if (regex2.test(input)) {
console.log("Regex is regex2");
const unWanted = "-,";
const wanted = "-";
const input2 = input.slice().toString().replace(unWanted, wanted);
// console.log(input2);
//drop - from methods
const newMethods = input2
.replace(/[0-9]|-[0-9]/g, "")
.replace(/,-,/g, ",")
.replace(/-,/g, "");
// console.log(newMethods);
const processedItems = [input2.split(","), newMethods];
return processedItems;
// change -, input to -input
} else if (
regex1.test(input) == false ||
regex2.test(input) == false ||
regex3.test(input) == false ||
regex4.test(input) == false
) {
console.log(input + " doesnt have regex");
// console.log(input);
const processedItems = [input.toString().split(","), clickedMethods];
return processedItems;
}
};
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.