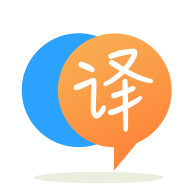
[英]AutoMapper: Map single object to IEnumerable/Collection of children while passing properties
[英]Automapper - map properties to IEnumerable
我正在使用 .NetCore 和 EntityFramework Core 创建一个 Web API。 我有一个基于这个模型的对象:
public partial class Person
{
public int Person_Id { get; set; }
public string Nickname { get; set; }
public string City { get; set; }
}
我有这样的资源:
public string Property { get; set; }
public string V1 { get; set; }
public string V2 { get; set; }
我想使用 Automapper 将模型的每个属性(Person_Id、Nickname、City)映射到资源的 IEnumerable,以便我实现如下目标:
[
{
Property: Person_Id,
V1: null,
V2: null
},
{
Property: Nickname,
V1: null,
V2: null
},
{
Property: City,
V1: null,
V2: null
}
]
因此每个属性都成为 IEnumerable 中的一个新条目。 我该怎么做呢?
这种逻辑是非常自定义的,我认为 Automapper 无法处理它,仅仅因为它不是 1:1 映射而是 1:3(如果您想在Person
类上拥有更多属性,甚至可以更多)。 所以我建议你用 Reflection 来做,而不是浪费时间用 Automapper 来做。 不要误会我的意思 - Automapper 是最好的 1:1 映射,但我从未见过它用于这种情况。 只是去自定义逻辑:
public partial class Person
{
public int Person_Id { get; set; }
public string Nickname { get; set; }
public string City { get; set; }
}
public partial class Resource
{
public string Property { get; set; }
public string V1 { get; set; }
public string V2 { get; set; }
}
class Program
{
static void Main(string[] args)
{
var item = new Person()
{
Person_Id = 1,
City = "New York",
Nickname = "John"
};
PropertyInfo[] props = typeof(Person).GetProperties(BindingFlags.Public | BindingFlags.Instance);
List<Resource> result = new List<Resource>();
foreach (var prop in props)
{
result.Add(new Resource()
{
Property = prop.Name,
V1 = prop.GetValue(item).ToString(),
V2 = null
});
}
// result is now [ { Property: Person_Id, V1: "1", V2: null }, { Property: Nickname, V1: "John", V2: null }, { Property: City, V1: "New York", V2: null } ]
}
}
当然,您可以通过不遍历属性来加速该过程,而只需使用nameof(Person.Person_Id)
等对属性名称进行硬编码
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.