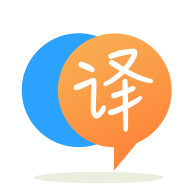
[英]How to parse objects that has a sealed class param in kotlin android development using Room?
[英]Android Room, how to save an entity with one of the variables being a sealed class object
我想在我的 Room 数据库中保存一个对象,其中一个变量可以是 on 类型或另一个。 我认为密封类是有意义的,所以我采用了这种方法:
sealed class BluetoothMessageType() {
data class Dbm(
val data: String
) : BluetoothMessageType()
data class Pwm(
val data: String
) : BluetoothMessageType()
}
甚至这个,但没有必要。 我发现这给了我更多错误,因为它不知道如何处理打开的 val,所以如果我找到第一个版本的解决方案,无论如何我都会很高兴。
sealed class BluetoothMessageType(
open val data: String
) {
data class Dbm(
override val data: String
) : BluetoothMessageType()
data class Pwm(
override val data: String
) : BluetoothMessageType()
}
然后是实体类
@Entity(tableName = MESSAGES_TABLE_NAME)
data class DatabaseBluetoothMessage(
@PrimaryKey(autoGenerate = true)
val id: Long = 0L,
val time: Long = Instant().millis,
val data: BluetoothMessageType
)
我还创建了一个 TypeConverter 来将其转换为字符串,因此我认为这不是问题。
首先,这可能吗? 我认为这应该以与抽象类类似的方式起作用,但我也没有设法找到一个可行的解决方案。 如果不可能,当我想保存一些可能是一种或另一种类型的数据(如果不是密封类)时,我应该采取哪种方法?
就我而言,我做了以下事情:
sealed class Status2() {
object Online : Status2()
object Offline : Status2()
override fun toString(): String {
return when (this) {
is Online ->"Online"
is Offline -> "Offline"
}
}
}
class StatusConverter{
@TypeConverter
fun toHealth(value: Boolean): Status2 {
return if (value){
Status2.Online
} else{
Status2.Offline
}
}
@TypeConverter
fun fromHealth(value: Status2):Boolean {
return when(value){
is Status2.Offline -> false
is Status2.Online -> true
}
}
}
@Dao
interface CourierDao2 {
@Insert(onConflict = OnConflictStrategy.REPLACE)
suspend fun insertStatus(courier: CourierCurrentStatus)
@Query("SELECT * FROM CourierCurrentStatus")
fun getCourierStatus(): Flow<CourierCurrentStatus>
}
@Entity
data class CourierCurrentStatus(
@PrimaryKey
val id: Int = 0,
var status: Status2 = Status2.Offline
)
它就像一个魅力
当我们尝试在我们的领域中使用多态时遇到了这样的问题,我们是这样解决的:
我们有一个看起来像这样的Photo
模型:
sealed interface Photo {
val id: Long
data class Empty(
override val id: Long
) : Photo
data class Simple(
override val id: Long,
val hasStickers: Boolean,
val accessHash: Long,
val fileReferenceBase64: String,
val date: Int,
val sizes: List<PhotoSize>,
val dcId: Int
) : Photo
}
Photo
里面有PhotoSize
,它看起来像这样:
sealed interface PhotoSize {
val type: String
data class Empty(
override val type: String
) : PhotoSize
data class Simple(
override val type: String,
val location: FileLocation,
val width: Int,
val height: Int,
val size: Int,
) : PhotoSize
data class Cached(
override val type: String,
val location: FileLocation,
val width: Int,
val height: Int,
val bytesBase64: String,
) : PhotoSize
data class Stripped(
override val type: String,
val bytesBase64: String,
) : PhotoSize
}
为了实现这一点,我们的数据模块有很多工作要做。 我将把这个过程分解成三个部分,让它看起来更容易:
所以,一般使用 Room 和 SQL,很难保存这样的对象,所以我们不得不想出这个想法。 我们的PhotoEntity
(这是我们域中Photo
的本地版本,如下所示:
@Entity
data class PhotoEntity(
// Shared columns
@PrimaryKey
val id: Long,
val type: Type,
// Simple Columns
val hasStickers: Boolean? = null,
val accessHash: Long? = null,
val fileReferenceBase64: String? = null,
val date: Int? = null,
val dcId: Int? = null
) {
enum class Type {
EMPTY,
SIMPLE,
}
}
我们的PhotoSizeEntity
看起来像这样:
@Entity
data class PhotoSizeEntity(
// Shared columns
@PrimaryKey
@Embedded
val identity: Identity,
val type: Type,
// Simple columns
@Embedded
val locationLocal: LocalFileLocation? = null,
val width: Int? = null,
val height: Int? = null,
val size: Int? = null,
// Cached and Stripped columns
val bytesBase64: String? = null,
) {
data class Identity(
val photoId: Long,
val sizeType: String
)
enum class Type {
EMPTY,
SIMPLE,
CACHED,
STRIPPED
}
}
然后我们有这个复合类将PhotoEntity
和PhotoSizeEntity
结合在一起,所以我们可以检索我们域模型所需的所有数据:
data class PhotoCompound(
@Embedded
val photo: PhotoEntity,
@Relation(entity = PhotoSizeEntity::class, parentColumn = "id", entityColumn = "photoId")
val sizes: List<PhotoSizeEntity>? = null,
)
所以我们的dao
应该能够存储和检索这些数据。 为了灵活起见, PhotoEntity
和PhotoSizeEntity
可以有两个daos
,而不是一个,但在这个例子中,我们将使用一个共享的,它看起来像这样:
@Dao
interface IPhotoDao {
@Transaction
@Query("SELECT * FROM PhotoEntity WHERE id = :id")
suspend fun getPhotoCompound(id: Long): PhotoCompound
@Transaction
suspend fun insertOrUpdateCompound(compound: PhotoCompound) {
compound.sizes?.let { sizes ->
insertOrUpdate(sizes)
}
insertOrUpdate(compound.photo)
}
@Insert(onConflict = OnConflictStrategy.REPLACE)
suspend fun insertOrUpdate(entity: PhotoEntity)
@Insert(onConflict = OnConflictStrategy.REPLACE)
suspend fun insertOrUpdate(entities: List<PhotoSizeEntity>)
}
解决了将数据保存到 SQL 数据库的问题之后,我们现在需要解决域实体和本地实体之间的转换问题。 我们的Photo
转换器又名适配器如下所示:
fun Photo.toCompound() = when(this) {
is Photo.Empty -> this.toCompound()
is Photo.Simple -> this.toCompound()
}
fun PhotoCompound.toModel() = when (photo.type) {
PhotoEntity.Type.EMPTY -> Photo.Empty(photo.id)
PhotoEntity.Type.SIMPLE -> this.toSimpleModel()
}
private fun PhotoCompound.toSimpleModel() = photo.run {
Photo.Simple(
id,
hasStickers!!,
accessHash!!,
fileReferenceBase64!!,
date!!,
sizes?.toModels()!!,
dcId!!
)
}
private fun Photo.Empty.toCompound(): PhotoCompound {
val photo = PhotoEntity(
id,
PhotoEntity.Type.EMPTY
)
return PhotoCompound(photo)
}
private fun Photo.Simple.toCompound(): PhotoCompound {
val photo = PhotoEntity(
id,
PhotoEntity.Type.SIMPLE,
hasStickers = hasStickers,
accessHash = accessHash,
fileReferenceBase64 = fileReferenceBase64,
date = date,
dcId = dcId,
)
val sizeEntities = sizes.toEntities(id)
return PhotoCompound(photo, sizeEntities)
}
对于PhotoSize
,它看起来像这样:
fun List<PhotoSize>.toEntities(photoId: Long) = map { photoSize ->
photoSize.toEntity(photoId)
}
fun PhotoSize.toEntity(photoId: Long) = when(this) {
is PhotoSize.Cached -> this.toEntity(photoId)
is PhotoSize.Empty -> this.toEntity(photoId)
is PhotoSize.Simple -> this.toEntity(photoId)
is PhotoSize.Stripped -> this.toEntity(photoId)
}
fun List<PhotoSizeEntity>.toModels() = map { photoSizeEntity ->
photoSizeEntity.toModel()
}
fun PhotoSizeEntity.toModel() = when(type) {
PhotoSizeEntity.Type.EMPTY -> this.toEmptyModel()
PhotoSizeEntity.Type.SIMPLE -> this.toSimpleModel()
PhotoSizeEntity.Type.CACHED -> this.toCachedModel()
PhotoSizeEntity.Type.STRIPPED -> this.toStrippedModel()
}
private fun PhotoSizeEntity.toEmptyModel() = PhotoSize.Empty(identity.sizeType)
private fun PhotoSizeEntity.toCachedModel() = PhotoSize.Cached(
identity.sizeType,
locationLocal?.toModel()!!,
width!!,
height!!,
bytesBase64!!
)
private fun PhotoSizeEntity.toSimpleModel() = PhotoSize.Simple(
identity.sizeType,
locationLocal?.toModel()!!,
width!!,
height!!,
size!!
)
private fun PhotoSizeEntity.toStrippedModel() = PhotoSize.Stripped(
identity.sizeType,
bytesBase64!!
)
private fun PhotoSize.Cached.toEntity(photoId: Long) = PhotoSizeEntity(
PhotoSizeEntity.Identity(photoId, type),
PhotoSizeEntity.Type.CACHED,
locationLocal = location.toEntity(),
width = width,
height = height,
bytesBase64 = bytesBase64
)
private fun PhotoSize.Simple.toEntity(photoId: Long) = PhotoSizeEntity(
PhotoSizeEntity.Identity(photoId, type),
PhotoSizeEntity.Type.SIMPLE,
locationLocal = location.toEntity(),
width = width,
height = height,
size = size
)
private fun PhotoSize.Stripped.toEntity(photoId: Long) = PhotoSizeEntity(
PhotoSizeEntity.Identity(photoId, type),
PhotoSizeEntity.Type.STRIPPED,
bytesBase64 = bytesBase64
)
private fun PhotoSize.Empty.toEntity(photoId: Long) = PhotoSizeEntity(
PhotoSizeEntity.Identity(photoId, type),
PhotoSizeEntity.Type.EMPTY
)
而已!
要将密封类保存到 Room 或 SQL,无论是作为Entity
还是作为Embedded
对象,您需要拥有一个包含所有属性的大数据类,来自所有密封变体,并使用Enum
类型来指示变体类型稍后用于域和数据之间的转换,或者如果您不使用 Clean Architecture,则在您的代码中进行指示。 坚硬,但坚固而灵活。 我希望Room
有一些注释可以生成这样的代码来摆脱样板代码。
PS:这个类取自Telegram的方案,他们也解决了与服务器通信时的多态问题。 在此处查看他们的 TL 语言: https ://core.telegram.org/mtproto/TL
PS2:如果你喜欢 Telegram 的 TL 语言,可以使用这个生成器从scheme.tl
文件生成 Kotlin 类: https ://github.com/tamimattafi/mtproto
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.