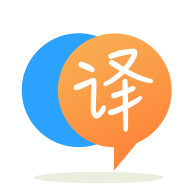
[英]how to get id from table which is generated dynamically using by using jquery and ajax?
[英]How to get submitted form id from many dynamically generated forms using jQuery on JS page
我有产品列表页面,其中所有列出的产品都有不同的表单名称和 id,使用 while 循环,如下面的代码段所述。 我想这样做,当用户单击特定产品的“添加”按钮时,应使用 AJAX/jQuery 提交该特定产品的表单。
product_listing.php
<script language="JavaScript" src="./product_listing.js"></script>
while loop to list products on page {
<form id="frm_add<?php print $rs_list->Fields("pkid"); ?>" name="frm_add<?php print $rs_list->Fields("pkid"); ?>" novalidate>
<input type="hidden" name="hdn_prodpkid" id="hdn_prodpkid" value="<?php print $rs_list->Fields("pkid"); ?>">
... other variables...
<button type="submit" name='btn_addtocart' id='btn_addtocart'>Add</button>
</form>
end of while loop }
我想要提交表单的 ID,以便我可以获取该提交表单的输入字段值。 但是在每次提交表单时,我都会得到最后一个表单的 ID。
那么如何在 JS 页面上获取唯一(仅提交)表单的 ID? 请指教。
当需要提交一个表单时,下面的代码可以完美运行。 在这种情况下,表单 ID 在 .php 和 .js 页面上都是预定义的。
product_listing.js
$(function() {
$("#"+NEED_SUBMITTED_FORM_ID_HERE+" input, #"+NEED_SUBMITTED_FORM_ID_HERE+" select").jqBootstrapValidation({
preventSubmit: true,
submitError: function($form, event, errors) {
...other code...
},
submitSuccess: function($form, event) {
event.preventDefault();
var hdn_prodpkid = $("input#hdn_prodpkid").val();
... other variables...
$.ajax({
url: "./product_addtocart_p.php",
type: "POST",
data: {
hdn_prodpkid: hdn_prodpkid,
... other variables...
},
cache: false,
success: function(data)
{
...other code...
}
}
});
});
-- 更新代码 --
product_listing.js
var id = '';
$(function() {
$("form").submit(function() {
var form = $(this);
var id = form.attr('id');
//alert(id + ' form submitted');
$("#"+id+" input, #"+id+" select").jqBootstrapValidation({
//alert(id + ' form submitted'); // Can't alert ID here
submitSuccess: function($form, event) {
event.preventDefault(); // prevent default submit behaviour
var hdn_prodpkid = $("input#hdn_prodpkid").val();
$.ajax({
url: "./product_addtocart_p.php",
type: "POST",
data: {
hdn_prodpkid: hdn_prodpkid
},
cache: false,
}) // End of Ajax
}, // End of submitSuccess
}); // End of jqBootstrapValidation
}); // End of form submit
}); // End of $(function())
当用户单击添加(添加到购物车)按钮时,将提交该按钮的父表单,因为您正在使用<button type="submit">Add</button>
。
type="submit"
属性用于表单提交。 如果您有多个 forms 然后,请使用 jQuery 获取提交的表单元素,如下所示 -
$("form").submit(function(){
var form = $(this);
var id = form.attr('id');
alert(id + ' form submitted');
});
当从您的product_listing.php
文件提交任何表单时,上述代码将运行并检索该提交表单的 ID。
之后,您可以运行 ajax 以对product_listing.js
文件进行其他计算。
- 更新 -
尝试使用以下示例
$(document).ready(function() {
var id; //For global access
$("form").submit(function(){
var form = $(this);
id = form.attr('id');
$("#"+id+" input, #"+id+" select").jqBootstrapValidation({
preventSubmit: true,
submitError: function($form, event, errors) {
...other code...
},
submitSuccess: function($form, event) {
event.preventDefault();
var hdn_prodpkid = $("input#hdn_prodpkid").val();
... other variables...
$.ajax({
url: "./product_addtocart_p.php",
type: "POST",
data: {
hdn_prodpkid: hdn_prodpkid,
... other variables...
},
cache: false,
success: function(data)
{
...other code...
}
}
});
});
});
alert(id);
现在我已经在 function 之外定义了 id 变量,因此您可以在 function 之外访问它。
希望这会奏效...
- 更新 -
我无法重现您提供的代码,所以我只建议您如何处理此问题。 首先,简化您的代码并检查哪些代码有效或哪些代码无效,然后,您必须检查为什么此代码无效。
如果可能的话,简化你的代码,让你知道为什么你的代码不工作。 我已经简化了你的代码如果可能的话,使用简化的代码。
谢谢
您的product_listing.js
var id = '';
$(function() {
$("form").submit(function(e) {
e.preventDefault(); // prevent default submit behaviour
var form = $(this);
id = form.attr('id');
var hdn_prodpkid = $("#id #hdn_prodpkid").val();
$.ajax({
url: "./product_addtocart_p.php",
type: "POST",
data: {
hdn_prodpkid: hdn_prodpkid
},
cache: false,
}) // End of Ajax
}); // End of form submit
}); // End of $(function())
请找到更改希望这有效
首先更新,按钮的代码 id 重复是无效的
通过 class 触发按钮的点击事件
<button class="submitButton" type="submit" name="btn_addtocart" id="btn_addtocart_".$rs_list->Fields("pkid"); ?>>添加
$(".submitButton").click(function () { var form = $(this).parent("form"); console.log(jQuery(form).attr("id")); });
HTML 中的标识符必须是唯一的,因此无论以下语句中具有hdn_prodpkid
id 的元素数量如何,都不要使用id="hdn_prodpkid"
var hdn_prodpkid = $("input#hdn_prodpkid").val();
将始终返回第一个元素的值。
我建议您不要使用hidden
元素,因为您实际上并没有将表单提交给 API,而是使用ajax()
调用。
立即解决方案(我不推荐这种方法)将使用
var hdn_prodpkid = $form.find("input#hdn_prodpkid").val();
使用自定义data-*
属性以保持任意信息的形式,即pkid
可以使用.data(key)
方法获取。
假设<input>
和<select>
是表单元素的后代,使用.find()
获取引用,然后对它们应用jqBootstrapValidation()
方法。
当使用jqBootstrapValidation()
提交表单时, submitSuccess($form, event)
回调方法给出了用户尝试提交的<form>
元素的引用(包装在 jQuery 中),即$form
。 它可用于获取所需后代元素的引用或从<form>
元素获取任意数据。
HTML
while () {
<form data-id="<?php print $rs_list->Fields("pkid"); ?>" novalidate>
<button type="submit">Add</button>
</form>
}
脚本
$("form").submit(function() {
var id= $(this).data('id'); //Example
$(this).find('input, select') //Target the descendant
.jqBootstrapValidation({
submitSuccess: function($form, event) {
//Perform the desired operation here to get the relvant data from thr form
event.preventDefault();
$.ajax({
url: "./product_addtocart_p.php",
type: "POST",
data: {
hdn_prodpkid: $form.data('id') //Get Arbitary data
},
cache: false,
})
}
});
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.