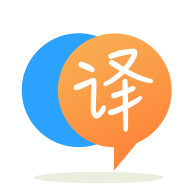
[英]How do I set a max quantity in darryldecode shopping cart in laravel?
[英]How can I store my Cart data to database in Laravel using DarrylDecode Cart functionalities
我试图通过制作一个小型电子商务网站项目来学习 Laravel 并为了实现购物车功能,我遇到了 DarrylDecode 购物车功能( https://github.com/darryldecode/laravelshoppingcart )
但很快我意识到用户的购物车数据存储在 session 中,每当用户注销并重新登录时,购物车数据就会丢失。 用户也无法从其他浏览器或其他设备访问购物车项目,因为它临时保存在特定浏览器的 session 上。 我想将相同的数据存储到数据库中并从那里访问它。 在文档中关于在数据库中存储数据的解释中很少有这方面的内容,但这并不那么清楚。 谁能给我一个关于如何实现这一目标的想法
Darryldecode cart 是一种在项目中实现购物车功能的双向方法。 就我而言,我正在尝试为愿望清单使用持久存储,这样当用户登录时,他们仍然会看到他们的愿望清单项目。 首先要做的是通过运行命令创建迁移
php artisan make:migration create_wishlist_storage_table
这将在 database/migration 目录中创建迁移文件,打开文件,并用这些代码行替换整个代码块。
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateWishlistStorageTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('wishlist_storage', function (Blueprint $table) {
$table->string('id')->index();
$table->longText('wishlist_data');
$table->timestamps();
$table->primary('id');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('wishlist_storage');
}
}
之后,运行php artisan migrate
命令。 This will create a wishlist_storage table in your database with columns id, wishlist_data, and the timestamps.The next thing is to create an eloquent model to handle our migration by running the command php artisan make:model DatabaseStorageModel
. 打开应用目录中的 DatabaseStorageModel.php 文件,并将整个代码块替换为以下代码行。
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class DatabaseStorageModel extends Model
{
//
/**
* Override eloquent default table
* @var string
*/
protected $table = 'wishlist_storage';
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'id', 'wishlist_data',
];
/**
* Mutator for wishlist_column
* @param $value
*/
public function setWishlistDataAttribute($value)
{
$this->attributes['wishlist_data'] = serialize($value);
}
/**
* Accessor for wishlist_column
* @param $value
* @return mixed
*/
public function getWishlistDataAttribute($value)
{
return unserialize($value);
}
}
接下来要做的是创建一个新的 class 以注入我们的购物车实例。 为此,使用您的应用命名空间创建一个名为 DatabaseStorage.php 的文件,然后粘贴这行代码。
<?php
namespace App;
use Darryldecode\Cart\CartCollection;
class DatabaseStorage {
public function has($key)
{
return DatabaseStorageModel::find($key);
}
public function get($key)
{
if($this->has($key))
{
return new CartCollection(DatabaseStorageModel::find($key)->wishlist_data);
}
else
{
return [];
}
}
public function put($key, $value)
{
if($row = DatabaseStorageModel::find($key))
{
// update
$row->wishlist_data = $value;
$row->save();
}
else
{
DatabaseStorageModel::create([
'id' => $key,
'wishlist_data' => $value
]);
}
}
}
命名文件和类的方式取决于您,但我正在解释我是如何做到的。 最后一步是使 DatabaseStorage class 成为我们购物车的默认存储。 运行命令
php artisan vendor:publish --provider="Darryldecode\Cart\CartServiceProvider" --tag="config"
在config目录下发布库配置文件名shopping_cart.php。 打开 shopping_cart.php 文件并替换
'storage'=>null,
和
'storage' => \App\DatabaseStorage::class,
您现在可以按照正常程序使用 controller 中的推车。
当我使用它时,我发现购物车现在对所有人都是公开的。 如果一个用户删除了该商品,则该商品将完全从购物车中删除,而其他拥有相同商品的用户则看不到这一点。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.