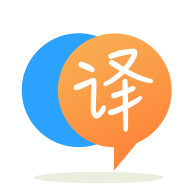
[英]How can I retrieve the value in a Hashmap stored in an arraylist type hashmap?
[英]How can I use a value I chose from the Arraylist on Hashmap?
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.Scanner;
public class Project {
public static void main(String[] args) {
TheMenu();
}
public static List<String> initPizzas() {
ArrayList<String> thePizza = new ArrayList<String>();
thePizza.add("Cheese");
thePizza.add("Mixed");
thePizza.add("Mushroom");
thePizza.add("Meat");
return thePizza;
}
public static void viewAllPizza(List<String> pizzas,String CustomerRef[]) {
ListIterator<String> it = pizzas.listIterator(); //to enumerate the pizzas in the menu
while(it.hasNext()) {
System.out.println(it.nextIndex() + " " + it.next());
}
}
public static void addPizza(List<String> pizzas, String CustomerRef[]) {
Scanner input = new Scanner(System.in);
String Exit = "E";
while(true) {
System.out.println("Enter the pizza you would like to add and E to exit");
String choice = input.next();
if(choice.equals(Exit)) {
break;
}else {
pizzas.add(choice);
; }
}
}
public static void TheMenu() {
String Customer[] = new String[10];
Scanner input = new Scanner(System.in);
List<String> Pizzas = initPizzas();
String option;
do {
System.out.println("\nMenu");
System.out.println("V: Views all Pizza");
System.out.println("A: To add a Pizza to the list");
System.out.println("O: To order Pizza");
System.out.println("Q: To exit");
option = input.next();
if (option.charAt(0) == 'V' )
{
viewAllPizza(Pizzas,Customer);
}
if (option.charAt(0) == 'A' )
{
addPizza(Pizzas,Customer);
}
if (option.charAt(0) == 'O') {
orderPizza(Pizzas, Customer);
}
}while (option.charAt(0) != 'Q');
}
public static void orderPizza(List<String> viewAllPizza,String CustomerRef[]) {
Map<String, Integer> counts = new HashMap<String, Integer>();
for(String str: viewAllPizza()) {
if(counts.containsKey(str)) {
counts.put(str, counts.get(str) + 1);
}else {
counts.put(str, 1);
}
}
for(Map.Entry<String, Integer> entry : counts.entrySet()) {
System.out.println(entry.getKey() + " = " + entry.getValue());
}
}
}
如果我犯了太多错误,我深表歉意,但我对 java 没有太多经验。 我一直在尝试使用 Hashmap 来订购我从 Arraylist 中选择的披萨,并使用扫描仪保留我订购的数量,但我无法弄清楚。
假设我想订购 4 块肉和 1 块奶酪比萨(我想使用扫描仪输入这些值)
-
Orders
-
Meat 4
Cheese 1
但是当我使用 orderPizza 方法时,它会取 Arraylist 中的所有比萨饼并添加 +1(有问题的部分)
-
Orders
-
Cheese 1
Mixed 1
Mushroom 1
Meat 1
实现一个新方法addPizzaForOrder
以将披萨添加到您要订购的披萨列表中。 您需要在该方法中更新counts
map。
public static void addPizzaForOrder(String CustomerRef[],
Map<String, Integer> counts) {
Scanner input = new Scanner(System.in);
String Exit = "E";
while(true) {
System.out.println("Enter the pizza you would like to order and E to exit");
String choice = input.next();
if(choice.equals(Exit)) {
break;
}else {
if(counts.containsKey(choice)) {
counts.put(choice, counts.get(choice) + 1);
} else {
counts.put(choice, 1);
}
}
}
}
public static void orderPizza(Map<String, Integer> counts,String CustomerRef[]) {
Map<String, Integer> counts = new HashMap<String, Integer>();
addPizzaForOrder(Customer, counts);
for(Map.Entry<String, Integer> entry : counts.entrySet()) {
System.out.println(entry.getKey() + " = " + entry.getValue());
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.