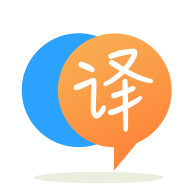
[英]I am getting Input string was not in a correct format although i used the same format over and over again
[英]API is getting called over and over again when I am using react hooks
我正在使用React Hooks
从现有的 API 获取数据。 这是我的代码
import React, { useState, useEffect } from "react";
export const People = () => {
const [people, setPeople] = useState([]);
const url = "http://127.0.0.1:5000/people";
async function fetchData() {
console.log("calling api .. ");
const res = await fetch(url);
res.json().then((res) => setPeople(res));
}
useEffect(() => {
fetchData();
});
return (
<div>
<ul>
{people &&
people.map((person) => <li key={person.id}>{person.name}</li>)}
</ul>
</div>
);
};
const Dashboard = (props) => {
return (
<div>
<People />
</div>
);
};
export default Dashboard;
我遇到的问题是这个 API 被一遍又一遍地调用。 你能告诉我我在这里做错了什么吗?
谢谢
目前,使用useEffect(callback)
将在每个 render上执行回调。
尝试将useEffect
与空的依赖项数组一起使用。
如果你想运行一个效果并且只清理一次,你可以传递一个空数组(
[]
)作为第二个参数。 这告诉 React 你的效果不依赖于 props 或 state 的任何值,所以它永远不需要重新运行。
useEffect
用例的其他答案。useEffect(() => {
fetchData();
}, []);
至于组件的rest,应该是这样的(认为):
// Should be on the outer scope as the URL is decoupled from the component's logic
const url = "http://127.0.0.1:5000/people";
export const People = () => {
const [people, setPeople] = useState([]);
useEffect(() => {
// This way fetchData won't re-assigned on every render
async function fetchData() {
console.log("calling api .. ");
const res = await fetch(URL);
// Consitstance, don't mix `then` and `await` syntax
const people = await res.json();
setPeople(people);
}
fetchData();
}, []);
return (
<div>
<ul>
{people &&
people.map((person) => <li key={person.id}>{person.name}</li>)}
</ul>
</div>
);
};
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.