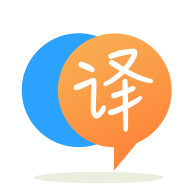
[英]How can I convert all components of a vector into absolute value using a subprogram?
[英]How can I get the maximum value of all the components in a vector?
我刚刚编写了一个程序,它保存用户输入的 4 分量向量(使用名为 save_vector 的save_vector
)并打印它(使用名为 print_vector 的print_vector
)。 此外,它还创建了一个名为vabs
的新向量,该向量具有相同的分量但绝对值(正)。
现在我试图通过使用 function maximum value
在vabs
向量中找到最大值。 但是,即使我在向量中输入非零分量,我也只能得到这个结果: 0.000000
。 你能帮我弄清楚我错过了什么吗? 谢谢: :-)
#include <stdio.h>
void print_vector(int N,float * V);
void save_vector(int N,float * V);
void absolute_values(int N, float * V);
void maximum_value(int N, float * VABS);
int main(void)
{
const int n=5;
int i;
float v[n],vabs[n];
puts("Enter the 5 components of the vector:");
save_vector(n, v);
puts("\nThe vector is:");
print_vector(n, v);
puts("\nThe absolute vector is:");
absolute_values(n, v);
puts("\nThe maximum value is:");
maximum_value(n, vabs);
return 0;
}
void save_vector(int N, float * V)
{
int i;
for(i=0;i<N;i++)
scanf("%f",V+i);
}
void print_vector(int N, float * V)
{
int i;
for(i=0;i<N;i++)
printf(" %.2f ",*(V+i));
}
void absolute_values(int N, float * V)
{
int i;
float VABS[N];
for(i=0;i<N;i++)
{
VABS[i]=((V[i]<0)?-V[i]:V[i]);
printf(" %f", VABS[i]);
}
}
void maximum_value(int N, float * VABS)
{
int i;
float maximum;
maximum = VABS[0];
for (i = 1; i < 5; i++)
{
if (VABS[i] > maximum)
{
maximum = VABS[i];
}
}
printf(" %f", maximum);
}
该代码未按预期工作,因为:
您将一个空向量vabs
传递给 function 应该找到 max_value。
In the function that assigns the absolute values, your VABS
vector is local, it will be lost as the function returns, you need to pass the main
vabs
vector to the function as an argument so that the changes can be saved and used to find the最大值:
void absolute_values(int N, float *V, float *VABS) //pass vabs as argument
{
int i;
//float VABS[N]; this is local, it's lost when the function returns
for (i = 0; i < N; i++)
{
VABS[i] = ((V[i] < 0) ? -V[i] : V[i]);
printf(" %f", VABS[i]);
}
}
主要是:
int main(void)
{
//...
puts("\nThe absolute vector is:");
absolute_values(n, v, vabs);
puts("\nThe maximum value is:");
maximum_value(n, vabs);
return 0;
}
这是固定代码的运行示例。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.