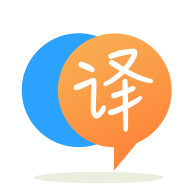
[英]JavaScript How to replace first element of an array with each element of another array
[英]Replace the empty element of an array with another array or with another element in javascript | javascript | array
假设我在 javascript 中有一个数组,内容如下。
let arr = [1, 2, , 4]; // [1, 2, empty, 4]
arr[5] = 6; // [1, 2, empty, 4, empty, 6]
如您所见,arr 变量在第 2 个和第 4 个 position 中有一个空值。 我想用提供的数组填充这些空值,就像填充一样
let replace_empty_with = [3, 5];
所以,首先,我想知道一个数组是否有空值,所以我使用以下方法
arr.includes(undefined);
// or
Object.values(arr).length == arr.length
现在如何用 replace_empty_with 数组变量的值填充原始数组(arr 变量)的空值?
所需的 output arr = [1, 2, 3, 4, 5];
我尝试使用数组的 forEach function 来了解特定元素是否具有未定义或 null 值,但我没有成功。
另外,我尝试使用 indexOf 来查找数组的第一个未定义元素,但它始终返回 -1 作为 indexOf(undefined) - state 表示数组中没有未定义的元素。
您已经创建了一个稀疏数组(一个有孔的数组)。 Array.forEach()
或Array.map()
等数组方法跳过了这个漏洞。
您可以使用Array.from()
迭代稀疏数组,并在原始数组中不存在当前索引 ( i
) 时通过从replace_empty_with
获取值来生成新数组:
const arr = [1, 2, , 4]; // [1, 2, empty, 4] arr[5] = 6; // [1, 2, empty, 4, empty, 6] const replace_empty_with = [3, 5]; const result = Array.from(arr, (v, i) => i in arr? v: replace_empty_with.shift() ) console.log(result);
您可以使用in
运算符来检测数组中的孔。
迭代它,并填写:
let arr = [1, 2, , 4, , 6] let replace_empty_with = [3, 5] for(let i=0; i<arr.length; i++){ if(.(i in arr) && replace_empty_with.length) arr[i] = replace_empty_with.shift() } console.log(arr)
请注意, in
运算符具有检测具有undefined
显式值的属性的优点(或缺点,具体取决于目标)。
也就是说,上面的代码不会在以下内容中填写任何内容:
let arr = [1, 2, undefined, 4, undefined]
要替换它们,请使用=== undefined
代替:
let arr = [1, 2, , 4, , 6] let replace_empty_with = [3, 5] for(let i=0; i<arr.length; i++){ if(arr[i].== (void 0) && replace_empty_with.length) arr[i] = replace_empty_with.shift() } console.log(arr)
可以像这样使用一个简单的 for 循环:
let arr = [1, 2, , 4]; // [1, 2, empty, 4] arr[5] = 6; // [1, 2, empty, 4, empty, 6] let replace_empty_with = [3, 5]; let nextPos = 0 for (let i=0; i<arr.length; i++) { if (arr[i] === undefined) { arr[i] = replace_empty_with[nextPos++] if (nextPos >= replace_empty_with.length) break } } console.log(arr)
您可以在有孔的数组之前使用...
扩展运算符,将孔转换为undefined
。
一旦你这样做了Array.prototype.map
可以用来检查是否存在undefined
的值,如果发现用替换数组中的值替换它:
let arr = [1, 2, , 4]; // [1, 2, empty, 4] arr[5] = 6; // [1, 2, empty, 4, empty, 6] let replace_empty_with = [3, 5]; function replaceEmpty(arr, replacer){ return [...arr].map(e => e === undefined? replacer.shift(): e); } console.log(replaceEmpty(arr, replace_empty_with));
您可以循环并检查是否有一些元素可以添加到数组中。
let array = [1, 2, , 4], replace = [3, 5]; for (let i = 0; replace.length; i++) { if (i in array) continue; array[i] = replace.shift(); } console.log(array);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.