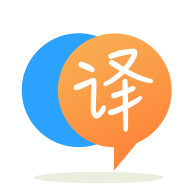
[英]Google sign_in_failed error in a Flutter app using firebase auth
[英]Unable to sign up using Firebase Auth in Flutter
我正在为我的应用程序使用 Firebase,我已经正确设置了所有内容,并且 Firebase Firestore 正常工作,我能够在那里读取和写入数据,但是当我尝试在 Z035489FF8D09241F5Z 中创建用户时,我收到此消息控制台消息:
I/BiChannelGoogleApi( 2228): [FirebaseAuth: ] getGoogleApiForMethod() returned Gms: com.google.firebase.auth.api.internal.zzaq@fc008c3
W/DynamiteModule( 2228): Local module descriptor class for com.google.firebase.auth not found.
I/FirebaseAuth( 2228): [FirebaseAuth:] Preparing to create service connection to gms implementation
I/lutter_firebase( 2228): type=1400 audit(0.0:1201): avc: denied { sendto } for path="/dev/socket/logdw" scontext=u:r:untrusted_app:s0:c512,c768 tcontext=u:r:init:s0 tclass=unix_dgram_socket permissive=1
E/GmsClientSupervisor( 2228): Timeout waiting for ServiceConnection callback com.google.firebase.auth.api.gms.service.START
E/GmsClientSupervisor( 2228): java.lang.Exception
E/GmsClientSupervisor( 2228): at com.google.android.gms.common.internal.zze.handleMessage(Unknown Source:53)
E/GmsClientSupervisor( 2228): at android.os.Handler.dispatchMessage(Handler.java:101)
E/GmsClientSupervisor( 2228): at com.google.android.gms.internal.common.zze.dispatchMessage(Unknown Source:8)
E/GmsClientSupervisor( 2228): at android.os.Looper.loop(Looper.java:164)
E/GmsClientSupervisor( 2228): at android.app.ActivityThread.main(ActivityThread.java:6541)
E/GmsClientSupervisor( 2228): at java.lang.reflect.Method.invoke(Native Method)
E/GmsClientSupervisor( 2228): at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
E/GmsClientSupervisor( 2228): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
W/DynamiteModule( 2228): Local module descriptor class for com.google.firebase.auth not found.
I/FirebaseAuth( 2228): [FirebaseAuth:] Preparing to create service connection to gms implementation
这是pubspec.yaml
文件:
name: chat_app_flutter_firebase
description: A new Flutter project.
version: 1.0.0+1
environment:
sdk: ">=2.3.0 <3.0.0"
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^0.1.2
cloud_firestore: ^0.13.5
firebase_auth: ^0.16.0
dev_dependencies:
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
auth_screen.dart
import 'package:flutter/material.dart';
import 'package:firebase_auth/firebase_auth.dart';
import 'package:flutter/services.dart';
import 'package:cloud_firestore/cloud_firestore.dart';
import '../widgets/auth/auth_form.dart';
class AuthScreen extends StatefulWidget {
@override
_AuthScreenState createState() => _AuthScreenState();
}
class _AuthScreenState extends State<AuthScreen> {
final _auth = FirebaseAuth.instance;
var _isLoading = false;
void _submitAuthForm(
String email,
String password,
String username,
bool isLogin,
BuildContext ctx,
) async {
AuthResult authResult;
try {
setState(() {
_isLoading = true;
});
if (isLogin) {
authResult = await _auth.signInWithEmailAndPassword(
email: email,
password: password,
);
} else {
authResult = await _auth.createUserWithEmailAndPassword(
email: email,
password: password,
);
await Firestore.instance
.collection('users')
.document(authResult.user.uid)
.setData({
'username': username,
'email': email,
});
}
} on PlatformException catch (err) {
var message = 'An error occurred, pelase check your credentials!';
if (err.message != null) {
message = err.message;
}
Scaffold.of(ctx).showSnackBar(
SnackBar(
content: Text(message),
backgroundColor: Theme.of(ctx).errorColor,
),
);
setState(() {
_isLoading = false;
});
} catch (err) {
print(err);
setState(() {
_isLoading = false;
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Theme.of(context).primaryColor,
body: AuthForm(
_submitAuthForm,
_isLoading,
),
);
}
}
auth_form.dart
import 'package:flutter/material.dart';
class AuthForm extends StatefulWidget {
AuthForm(
this.submitFn,
this.isLoading,
);
final bool isLoading;
final void Function(
String email,
String password,
String userName,
bool isLogin,
BuildContext ctx,
) submitFn;
@override
_AuthFormState createState() => _AuthFormState();
}
class _AuthFormState extends State<AuthForm> {
final _formKey = GlobalKey<FormState>();
var _isLogin = true;
var _userEmail = '';
var _userName = '';
var _userPassword = '';
void _trySubmit() {
final isValid = _formKey.currentState.validate();
FocusScope.of(context).unfocus();
if (isValid) {
_formKey.currentState.save();
widget.submitFn(_userEmail.trim(), _userPassword.trim(), _userName.trim(),
_isLogin, context);
}
}
@override
Widget build(BuildContext context) {
return Center(
child: Card(
margin: EdgeInsets.all(20),
child: SingleChildScrollView(
child: Padding(
padding: EdgeInsets.all(16),
child: Form(
key: _formKey,
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
TextFormField(
key: ValueKey('email'),
validator: (value) {
if (value.isEmpty || !value.contains('@')) {
return 'Please enter a valid email address.';
}
return null;
},
keyboardType: TextInputType.emailAddress,
decoration: InputDecoration(
labelText: 'Email address',
),
onSaved: (value) {
_userEmail = value;
},
),
if (!_isLogin)
TextFormField(
key: ValueKey('username'),
validator: (value) {
if (value.isEmpty || value.length < 4) {
return 'Please enter at least 4 characters';
}
return null;
},
decoration: InputDecoration(labelText: 'Username'),
onSaved: (value) {
_userName = value;
},
),
TextFormField(
key: ValueKey('password'),
validator: (value) {
if (value.isEmpty || value.length < 7) {
return 'Password must be at least 7 characters long.';
}
return null;
},
decoration: InputDecoration(labelText: 'Password'),
obscureText: true,
onSaved: (value) {
_userPassword = value;
},
),
SizedBox(height: 12),
if (widget.isLoading) CircularProgressIndicator(),
if (!widget.isLoading)
RaisedButton(
child: Text(_isLogin ? 'Login' : 'Signup'),
onPressed: _trySubmit,
),
if (!widget.isLoading)
FlatButton(
textColor: Theme.of(context).primaryColor,
child: Text(_isLogin
? 'Create new account'
: 'I already have an account'),
onPressed: () {
setState(() {
_isLogin = !_isLogin;
});
},
)
],
),
),
),
),
),
);
}
}
我以前曾使用较旧的 Flutter SDK 开发过类似的应用程序,并且一切正常。
当前 Flutter SDK 信息:
Flutter 1.17.0 • 通道稳定 • https://github.com/flutter/flutter.git
框架 • 修订版 e6b34c2b5c(9 天前) • 2020-05-02 11:39:18 -0700,引擎 • 修订版 540786dd51 工具 • Dart 2.8.1
GitHub 回购: GitHub 回购
任何帮助将不胜感激
问题出在我的模拟器上,在物理设备上运行它并且它工作。
我使用的是 Genymotion 模拟器,它没有安装 Play Store,所以我使用 GApps 安装了它们,这也解决了模拟器的问题。
此外,使用具有 Play 商店服务的 Android Studio 的模拟器也可以解决问题。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.