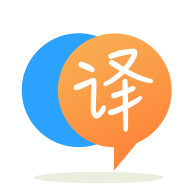
[英]How to read txt file with slashes “/” with scanner input and put them into arrays/strings
[英]How to read txt file with slashes “/” with scanner input and reads certain parts?
如何获取 txt 文件的某些部分并将它们放入 arrays 或字符串中?
我已经有一个代码可以读取 txt 文件中的 25 个项目。
try {
File file = new File("filepath");
Scanner sc = new Scanner(file);
String[] ids = new String[25];
String[] names = new String[25];//---- set your array length
String[] prices = new String[25];
String[] stocks = new String[25];
int counter = 0;
while (sc.hasNext()) {
String data = sc.nextLine();
if (data.contains("/")) {
String[] elements = data.split("/");
ids[counter] = elements[0].trim();
names[counter] = elements[1].trim();
prices[counter] = elements[2].trim();
stocks[counter] = elements[3].trim();
// other elements[x] can be saved in other arrays
counter++;
}
}
image.setIcon(graphicsconsole[productTable.getSelectedRow()]);
iteminfo.setText("" + names[productTable.getSelectedRow()]);
itemdescription.setText("Price: P " + prices[productTable.getSelectedRow()]);
itemstock.setText("Stocks: " + stocks[productTable.getSelectedRow()]);
} catch (FileNotFoundException ex) {
ex.printStackTrace();
} catch (ArrayIndexOutOfBoundsException exx) {
}
正如您所看到的,它现在被安排到 arrays 中,我将它们用于JTable
的选择列表。
如果我打印ids[counter] = elements[0].trim();
这将是:
00011
00012
00013
00014
00015
and so on...
问题是,如果我想获取 txt 文件的某个部分呢? 例如,不是从 ID 号00011
开始读取,而是希望它读取 ID 00012
等等?
txt文件内容:
00011 / Call of Duty: Modern Warfare / 2499 / 10
00012 / The Witcher 3: Wild Hunt / 1699 / 15
00013 / Doom Eternal / 2799 / 20
00014 / Outlast 2 / 1999 / 11
00015 / Forza Horizon 4 / 2799 / 5
如果我想在 ID 00011
之后获取 ID,则预期的 output 将是:
00012
00013
00014
00015
我尝试编辑int counter = 0
和counter++;
但它没有 output 任何东西。 任何帮助将不胜感激,谢谢!
请注意,以下解决方案会读取 memory 中的所有文件内容,请勿尝试将其用于非常大的文件。
File f = new File("filepath");
// read all lines
List<String> lines = Files.readAllLines(f.toPath());
lines.stream()
// skip lines before 00013 (change here to your needs)
.dropWhile(l -> !l.startsWith("00013"))
// ensure the lines have '/' character
.filter(l -> l.contains("/"))
.forEach(data -> {
// do something with each line, starting the one with 00013
String[] elements = data.split("/");
String id = elements[0].trim();
System.out.println(id);
});
// if you just want the IDs ...
lines.stream()
.dropWhile(l -> !l.startsWith("00013"))
.filter(l -> l.contains("/"))
// get the ID for each line
.map(l -> l.split("/")[0].trim())
// print it
.forEach(System.out::println);
// if you want the IDs to a List instead of printing ...
final List<String> ids = lines.stream()
.dropWhile(l -> !l.startsWith("00013"))
.filter(l -> l.contains("/"))
.map(l -> l.split("/")[0].trim())
.collect(Collectors.toList());
只是为了说明dropWhile
部分:
输入:
00011 / Call of Duty: Modern Warfare / 2499 / 10
00012 / The Witcher 3: Wild Hunt / 1699 / 15
00013 / Doom Eternal / 2799 / 20
00014 / Outlast 2 / 1999 / 11
00015 / Forza Horizon 4 / 2799 / 5
代码:
lines.stream()
.dropWhile(l -> !l.startsWith("00013"))
.forEach(System.out::println);
Output:
00013 / Doom Eternal / 2799 / 20
00014 / Outlast 2 / 1999 / 11
00015 / Forza Horizon 4 / 2799 / 5
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.