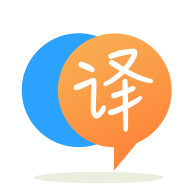
[英]How to read inputs from a file and to write outputs to another file in C
[英]How to read from file and write another in c
我有一个这样的输入文件:
This is 1nd Line. This is 2nd Line. This 3rd Line.
我需要 output 文件,例如
奇数行.txt:
This is 1nd Line. This 3rd Line.
EvenLines.txt:
This is 2nd Line.
这是我的代码。 并且没有按我的意愿工作。
char buf[256];
int ch;
int lines;
lines = 1;
FILE *myFile = fopen(argv[1], "r");
if (myFile == NULL) {
printf("Open error \n");
exit(-1);
}
FILE *outFile = fopen("oddlines.txt", "w");
FILE *outFile1 = fopen("evenlines.txt", "w");
while (fgets(buf, sizeof(buf), myFile) != NULL) {
if (ch == '\n')
lines++;
else
if ((lines % 2) == 0)
fputs(buf, outFile1);
else
fputs(buf, outFile);
}
fclose(myFile);
fclose(outFile);
fclose(outFile1);
}
if (ch == '\n')
这段代码没有意义。 你想找到输入字符吗? 如果是,您可以使用strcspn
function:
int pos = strcspn ( buf, "\n" );
这些lines
应该由0
而不是1
初始化:
int lines = 0;
while(fgets(buf, sizeof(buf),myFile))
{
lines++;
int pos = strcspn ( buf, "\n" ); // find the enter character
buf[pos] = ' '; // add space at the end of string
buf[pos+1] = '\0';
if ((lines%2)==0) // if even
fputs (buf,outFile1);
else // if odd
fputs (buf,outFile);
}
您应该检查fopen
function:
FILE *myFile = fopen(argv[1], "r");
if(!myFile) {return -1;}
FILE *outFile = fopen("oddlines.txt", "w");
if(!outFile) {return -1;}
FILE *outFile1 = fopen("evenlines.txt", "w");
if(!outFile1) {return -1;}
以及命令行的条件:
if(argc < 2 ) {
printf("usage: %s <input_filename>", argv[0]);
return-1;
}
完整代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char const *argv[]) {
char buf[256];
int lines = 0;
if(argc < 2 ) {
printf("usage: %s <input_filename>", argv[0]);
return-1;
}
FILE *myFile = fopen(argv[1], "r");
if(!myFile) {return -1;}
FILE *outFile = fopen("oddlines.txt", "w");
if(!outFile) {return -1;}
FILE *outFile1 = fopen("evenlines.txt", "w");
if(!outFile1) {return -1;}
while(fgets(buf, sizeof(buf),myFile)!=NULL)
{
lines++;
int pos = strcspn ( buf, "\n" );
buf[pos] = ' ';
buf[pos+1] = '\0';
if ((lines%2)==0)
fputs (buf,outFile1);
else
fputs (buf,outFile);
}
fclose(myFile);
fclose(outFile);
fclose(outFile1);
}
输入文件:
This is 1nd Line.
This is 2nd Line.
This is 3rd Line.
This is 4th Line.
This is 5th Line.
This is 6th Line.
output 文件:
$cat evenlines.txt
This is 2nd Line. This is 4th Line. This is 6th Line.
$cat oddlines.txt
This is 1nd Line. This is 3rd Line. This is 5th Line.
if (ch == '\n')
问题出在这一行。
#include <stdio.h>
#include<string.h>
void main()
{
int line=1;
char buf[256];
FILE *fp, *fp_Odd, *fp_Even;
fp=fopen("USERS.TXT", "r");
fp_Odd=fopen("ODD.TXT", "a");
fp_Even=fopen("EVEN.TXT", "a");
if(fp == NULL)
{
printf("Open error \n");
exit(-1);
}
while(fgets(buf, sizeof(buf), fp)!=NULL)
{
if(strcmp(buf, "\n")==0)
line++;
else if(line%2 != 0)
fprintf(fp_Odd, "%s", buf);
else
fprintf(fp_Even, "%s", buf);
line++;
}
fclose(fp);
}
您可以检查此代码。 我已经进行了必要的更改。 希望你能理解。
目前尚不清楚您是否希望 output 文件包含输入文件中的行,或者是否必须连接这些行,从而去除换行符。
您的代码不起作用,因为测试if (ch == '\n')
具有未定义的行为,因为ch
未初始化。 因此,行计数器未正确更新,所有行 go 到其中一个文件中。
计算行数实际上并不像计算循环的迭代次数那么简单,因为输入文件中的某些行可能比fgets()
使用的数组的长度长。 您应该在写入后通过测试刚刚写入的行是否实际包含换行符来更新行计数器。
这是修改后的版本:
#include <stdio.h>
#include <string.h>
int main(int argc, char *argv[]) {
char buf[256];
int lines = 1;
FILE *myFile, *outFile, *outFile1;
if (argc < 2) {
printf("Missing argument\n");
return 1;
}
if ((myFile = fopen(argv[1], "r")) == NULL) {
printf("Open error for %s\n", argv[1]);
return 1;
}
if ((outFile = fopen("oddlines.txt", "w")) == NULL) {
printf("Open error for %s\n", "oddlines.txt");
return 1;
}
if ((outFile1 = fopen("evenlines.txt", "w")) == NULL) {
printf("Open error for %s\n", "evenlines.txt");
return 1;
}
while (fgets(buf, sizeof(buf), myFile) != NULL) {
if (lines % 2 == 0)
fputs(buf, outFile1);
else
fputs(buf, outFile);
/* if a full line was read, increment the line number */
if (strchr(buf, '\n')
lines++;
}
fclose(myFile);
fclose(outFile);
fclose(outFile1);
return 0;
}
这是一个不使用fgets()
的更简单的版本:
#include <stdio.h>
int main(int argc, char *argv[]) {
int c, out;
FILE *myFile, *outFile[2];
if (argc < 2) {
printf("Missing argument\n");
return 1;
}
if ((myFile = fopen(argv[1], "r")) == NULL) {
printf("Open error for %s\n", argv[1]);
return 1;
}
if ((outFile[0] = fopen("oddlines.txt", "w")) == NULL) {
printf("Open error for %s\n", "oddlines.txt");
return 1;
}
if ((outFile[1] = fopen("evenlines.txt", "w")) == NULL) {
printf("Open error for %s\n", "evenlines.txt");
return 1;
}
out = 0; /* current output file is "oddlines.txt" */
while ((c = getc(myFile)) != EOF) {
putc(c, outFile[out]);
if (c == '\n')
out = 1 - out; /* change current output file */
}
fclose(myFile);
fclose(outFile[0]);
fclose(outFile[1]);
return 0;
}
如果您想从第三个 output 文件中去除元音,只需包含<string.h>
,将该文件打开到FILE *noVowelFile
并在while
循环中添加一个测试:
out = 0; /* current output file is "oddlines.txt" */
while ((c = getc(myFile)) != EOF) {
if (strchr("aeiouAEIOU", c) == NULL) {
putc(c, noVowelFile);
}
putc(c, outFile[out]);
if (c == '\n')
out = 1 - out; /* change current output file */
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.