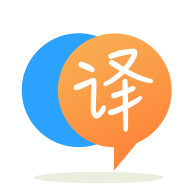
[英]How do I pass the response of one API as a request param in another API using Request-Promise
[英]How do I pass a parameter to API request 2, which I receive from API response 1 in react
我的 2 个 API 调用恰好同时发生,其中 API1 的响应将作为请求参数发送到 API2。 但是,该值未定义,因为直到那时才获取它。 有什么办法可以在反应中解决。
有多种方法可以解决这个问题,我将解释一种最新的也是最受追捧的解决问题的方法。
我相信您会在 JavaScript 中听说过 async/await,如果您还没有,我会建议您通过 MDN 文档中有关该主题的文档来了解 go。
这里有2个关键字, async && await ,我们一个一个来看看。
异步
在任何 function 之前添加 async 意味着一件简单的事情,而不是返回正常值,现在 function 将返回Promise
例如,
async function fetchData() {
return ('some data from fetch call')
}
如果您只需通过fetchData()在控制台中运行上述 function 即可。 您会看到,这个 function 有趣地返回了Promise ,而不是返回字符串值。
因此,简而言之,异步确保 function 返回 promise,并在其中包装非承诺。
等待
我敢肯定,到现在为止,您已经猜到了为什么我们在async之外还使用关键字 await ,仅仅是因为关键字await使 JavaScript 等到 promise (由 async 函数返回)稳定并返回其结果。
现在开始讨论如何使用它来解决您的问题,请按照以下代码片段进行操作。
async function getUserData(){
//make first request
let response = await fetch('/api/user.json');
let user = await response.json();
//using data from first request make second request/call
let gitResponse = await fetch(`https://api.github.com/users/${user.name}`)
let githubUser = await gitResponse.json()
// show the avatar
let img = document.createElement('img');
img.src = githubUser.avatar_url;
img.className = "promise-avatar-example";
document.body.append(img);
// wait 3 seconds
await new Promise((resolve, reject) => setTimeout(resolve, 3000));
img.remove();
return githubUser;
}
如您所见,上面的代码非常容易阅读和理解。 有关 JavaScript 中的 async/await 关键字的更多信息,另请参阅此文档。
Asyn/await 解决了你的问题:
const requests = async () =>{ const response1 = await fetch("api1") const result1 = await response1.json() // Now you have result from api1 you might use it for body of api2 for exmaple const response2 = await fetch("api2", {method: "POST", body: result1}) const result2 = await response1.json() }
如果您使用react hooks ,您可以使用Promise在 useEffect 中链接您的useEffect
调用
useEffect(() => {
fetchAPI1().then(fetchAPI2)
}, [])
fetch(api1_url).then(response => {
fetch(api2_url, {
method: "POST",
body: response
})
.then(response2 => {
console.log(response2)
})
})
})
.catch(function (error) {
console.log(error)
});
或者如果使用 axios
axios.post(api1_url, {
paramName: 'paramValue'
})
.then(response1 => {
axios.post(api12_url, {
paramName: response1.value
})
.then(response2 => {
console.log(response2)
})
})
.catch(function (error) {
console.log(error);
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.