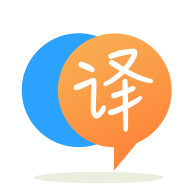
[英]How do I reference a variable in redux store from a component index.js (react.js)
[英]How can I access react redux state in index.js file?
我在 React 项目中使用 redux 进行状态管理,我在这样的组件中访问 redux reducer 文件状态:
组件.js
import { buyItem } from './itemActions';
import { connect } from 'react-redux';
function Comp(props) {
return (
<div>
<h3>{props.numOfItems}</h3>
<button onClick={() => props.buyItem('red')}>
Click
</button>
</div>
);
}
const mapState = (state) => {
return {
numOfItems: state.numOfItems
};
};
const mapDis = (dispatch) => {
return {
buyItem: (name) => dispatch(buyItem(name))
};
};
export default connect(mapState, mapDis)(Component);
这是包含所有状态的 reducer 文件:
import { BUY_ITEM } from './itemTypes';
const initialState = {
numOfItems: 0,
price: 0,
}
export const itemReducer = (state = initialState, action) => {
switch (action.type) {
case BUY_ITEM:
return {
...state,
price: state.price + state.products[action.payload].price,
};
default:
return state;
}
};
export default itemReducer;
这是 redux 操作文件:
import { BUY_ITEM } from './itemTypes';
export const buyItem = (name) => {
return {
type: BUY_ITEM,
payload: name
};
};
现在我想从 index.js 文件访问状态和操作,但没有导出默认方法,所以我可以从 redux 导入连接并将 index.js 连接到 redux。
这是 index.js 文件:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
如何从 index.js 文件访问状态和调用操作?
这更像是一个评论,但它只是说明你对 redux 感到困惑。
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import * as serviceWorker from './serviceWorker';
import { Provider } from 'react-redux';
import { createStore } from 'redux';
import { rootReducer } from './your.root.reducer';
import { App } from './App';
const store = createStore(rootReducer);
ReactDOM.render(
<React.StrictMode>
<Provider store={store}>
<App />
</Provider>
</React.StrictMode>,
document.getElementById('root')
);
// Now inside App.js
export const App = () => {
// ONLY NOW does it make sense to try to access the store/dispatch actions
}
只有在提供者的树中访问操作/状态才有意义
上面的组件树看起来(基本上)是这样的:
Root
-> Provider
-> App // NOW IT MAKES SENSE TO ACCESS STATE
对于 react-native 项目,您可以像这样访问它。 我试图访问用户保存的位置并像这样获取它。 干杯。
import notifee from '@notifee/react-native';
import {AppRegistry} from 'react-native';
import Geolocation from 'react-native-geolocation-service';
import 'react-native-gesture-handler';
import App from './App';
import {name as appName} from './app.json';
import {store} from './src/store/index';
store.subscribe(listener);
function listener() {
let state = store.getState();
console.log(state);
}
notifee.registerForegroundService(notification => {
return new Promise(() => {
Geolocation.watchPosition(
position => {
console.log(position);
},
error => {
console.log(error, 'Error');
},
{enableHighAccuracy: false, timeout: 15000},
);
});
});
AppRegistry.registerComponent(appName, () => App);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.