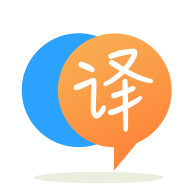
[英]How to access a member of a struct which is passed into a function as pointer to pointer parameter?
[英]How to access a pointer struct's member variables?
我已经有一段时间没有在 C++ 编程了,所以我一直在努力改进。 我有一个非常简单的登录 function,但是它不起作用。 登录 function 调用getUserAttempts()
返回指向包含用户详细信息(例如用户名和密码)的结构的指针。 但是,每当我尝试访问指针的结构变量时,程序就会崩溃。 我觉得我错过了一些明显的东西,但不能指望它。
#include <iostream>
#include <string>
#include <stdio.h>
#include <stdlib.h>
#include <unordered_map>
typedef std::unordered_map<std::string, std::string> unorderedMap;
typedef struct Credentials {
std::string username;
std::string password;
} structCred;
void login();
structCred* getUserAttempts();
void login() {
unorderedMap credentialMap;
credentialMap["username"] = "mypassword123";
structCred *p;
for (int i = 0; i < 3; i++) {
p = getUserAttempts();
auto it = credentialMap.find(p->username);
std::cout << it->first;
std::cout << it->second;
}
return;
}
structCred *getUserAttempts() {
structCred credentialAttempt;
std::string username, password;
std::cout << "Please enter your username: ";
std::getline(std::cin, credentialAttempt.username);
std::cout << "Please enter your password: ";
std::getline(std::cin, credentialAttempt.password);
return &credentialAttempt;
}
int main() {
std::cout << "Welcome..." << std::endl;
login();
return 0;
}
return &credentialAttempt;
这将返回一个指向函数局部变量credentialAttempt
的指针。 这不起作用,因为 function 返回时, function 本地立即被破坏,因此 function 返回指向不再存在的指针。 因此,取消引用返回的指针是未定义的行为。
相反,只需按值返回 object:
structCred getUserAttempts() {
structCred credentialAttempt;
std::string username, password;
std::cout << "Please enter your username: ";
std::getline(std::cin, credentialAttempt.username);
std::cout << "Please enter your password: ";
std::getline(std::cin, credentialAttempt.password);
return credentialAttempt;
}
在此 function 中:
structCred *getUserAttempts() {
structCred credentialAttempt;
// ...
return &credentialAttempt;
}
您正在将地址返回给在 function 末尾死亡的局部变量。 访问 memory 是未定义的行为。
相反,您应该为它分配 memory :
structCred *getUserAttempts() {
structCred *credentialAttempt = new structCred;
// ...
return credentialAttempt;
}
请注意,像credentialAttempt.username
这样的语句必须变成credentialAttempt->username
。
但是,返回原始指针通常不是一个好主意,因为无法知道释放 memory 的责任是谁。 相反,请使用unique_ptr
来为您释放 memory:
std::unique_ptr<structCred> getUserAttempts() {
auto credentialAttempt = make_unique<structCred>();
// ...
return credentialAttempt;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.