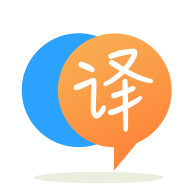
[英]How to move an apk file from root/data/app/ to storage/emulated/0 programmatically in Android Studio
[英]How to move a non media file from /storage/emulated/0/Download to getExternalFilesDir()
我创建了一个能够下载非媒体文件的应用程序。 现在我计划扩展应用程序以移动下载的文件(filetype=map)
。 我正在使用compileSdkVersion 29
和targetSdkVersion 29
。 这个https://developer.android.com/training/data-storage说我必须使用“存储访问框架”。 我使用了从这里截取的“ 如何在 android 10 上列出所有 pdf 文件? ”但它仅适用于媒体文件(uri 到文件)。 有人知道在哪里可以找到工作剪断吗?
为了复制像“whatever.map”这样的文件,必须在以下代码中进行哪些更改? 问题的重要部分是文件类型“map”,这意味着它是非媒体文件,mime 类型未知。 目标 sdk必须是29 。 当我 - 使用以下代码 - 选择像“whatever.png”这样的文件时,它可以工作。 但是在复制到 /storage/emulated/0/Download 之前将文件“whatever.map”重命名为“whatever.png”对于目标 sdk 29也是不可能的。
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/open_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="104dp"
android:layout_marginTop="144dp"
android:onClick="openFile"
android:text="Select"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/save_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="188dp"
android:layout_marginTop="280dp"
android:onClick="saveFile"
android:text="Copy"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="1.0"
app:layout_constraintStart_toEndOf="@+id/open_button"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
package org.hagerti.storagedemo;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.app.Activity;
import android.content.Intent;
import android.icu.text.SimpleDateFormat;
import android.net.Uri;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.channels.FileChannel;
import java.util.Date;
public class MainActivity extends AppCompatActivity {
final static String className = MainActivity.class.getSimpleName();
private static final int CREATE_REQUEST_CODE = 40;
private static final int OPEN_REQUEST_CODE = 41;
private static final int SAVE_REQUEST_CODE = 42;
final private static String myMimeType = "image/png";
//final private static String myMimeType = "text/map";
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
Uri currentUri = null;
if (resultCode == Activity.RESULT_OK) {
if (requestCode == SAVE_REQUEST_CODE) {
if (data != null) {
currentUri = data.getData();
writeFileContent(currentUri);
}
} else if (requestCode == OPEN_REQUEST_CODE) {
if (data != null) {
currentUri = data.getData();
try {
String content = readFileContent(currentUri);
} catch (IOException e) {
}
}
}
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void openFile(View view) {
Intent intent = new Intent(Intent.ACTION_OPEN_DOCUMENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
intent.setType(myMimeType);
startActivityForResult(intent, OPEN_REQUEST_CODE);
/**
* http://www.iana.org/assignments/media-types/media-types.xhtml
* Often used media types:
* image/jpeg
* audio/mpeg4-generic
* text/html
* audio/mpeg
* audio/aac
* audio/wav
* audio/ogg
* audio/midi
* audio/x-ms-wma
* video/mp4
* video/x-msvideo
* video/x-ms-wmv
* image/png
* image/jpeg
* image/gif
* .xml ->text/xml
* .txt -> text/plain
* .cfg -> text/plain
* .csv -> text/plain
* .conf -> text/plain
* .rc -> text/plain
* .htm -> text/html
* .html -> text/html
* .pdf -> application/pdf
* .apk -> application/vnd.android.package-archive
*/
}
private String readFileContent(Uri uri) throws IOException {
Log.e(className, " readFileContent uri.getPath()=" + uri.getPath());
InputStream inputStream =
getContentResolver().openInputStream(uri);
BufferedReader reader =
new BufferedReader(new InputStreamReader(inputStream));
StringBuilder stringBuilder = new StringBuilder();
String currentline;
while ((currentline = reader.readLine()) != null) {
stringBuilder.append(currentline + "\n");
}
inputStream.close();
return stringBuilder.toString();
}
public void saveFile(View view) {
Intent intent = new Intent(Intent.ACTION_OPEN_DOCUMENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
//intent.setType("text/plain");
intent.setType(myMimeType);
startActivityForResult(intent, SAVE_REQUEST_CODE);
}
private void writeFileContent(Uri uri) {
Log.e(className, " writeFileContent uri.getPath()=" + uri.getPath());
FileChannel inChannel = null;
FileChannel outChannel = null;
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
File expFile = new File("/storage/emulated/0/Android/data/org.hagerti.storagedemo/files" + File.separator + timeStamp + ".map");
try {
inChannel = new FileInputStream(getContentResolver().openFileDescriptor(uri, "r").getFileDescriptor()).getChannel();
outChannel = new FileOutputStream(expFile).getChannel();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
try {
inChannel.transferTo(0, inChannel.size(), outChannel);
} catch (IOException e) {
e.printStackTrace();
} finally {
if (inChannel != null)
try {
inChannel.close();
Log.e(className, " writeFileContent inChannel closed");
} catch (IOException e) {
e.printStackTrace();
}
if (outChannel != null)
try {
outChannel.close();
Log.e(className, " writeFileContent outChannel closed");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.