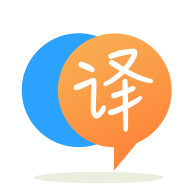
[英]Getting ID of all elements of a certain class into an array in javascript
[英]Getting All Elements In An Array (Javascript)
我正在尝试检查一个字符串是否包含我存储在数组中的某些单词......但是我是 JS 的新手,所以我不知道如何检查数组中的所有元素。
这是一个例子:
const fruits = ["apple", "banana", "orange"]
我实际上是在检查是否有人在聊天中发送脏话。
if(message.content.includes(fruits)){executed code}
但是我的问题是当我检查水果时它会做任何事情但是当我检查数组中的特定元素时fruits[0] //returns apple
它实际上会检查那个......所以我的问题/问题是我如何检查数组中所有元素的字符串,而不仅仅是苹果。
您对includes
的用法是错误的。
来自 MDN:
includes() 方法确定数组是否在其条目中包含某个值,并根据需要返回 true 或 false。
arr.includes(valueToFind[, fromIndex])
const fruits = ["apple", "banana", "orange"]; const swearWord = "orange"; // execute is available if (fruits.includes(swearWord)) console.log("Swear word exists."); else console.log("Swear word doesn't exist.");
要检查其他方式,如果字符串包含数组的脏话:
const fruits = ["apple", "banana", "orange"]; const swearWord = "this contains a swear word. orange is the swear word"; // execute is available if (checkForSwearWord()) console.log("Swear word exists."); else console.log("Swear word doesn't exist."); function checkForSwearWord() { for (const fruit of fruits) if (swearWord.includes(fruit)) return true; return false; }
你得到它相反的方式。 您必须在数据数组上使用 .includes 来检查数组是否包含您要查找的单词。
const fruits = ["apple", "banana", "orange"] console.log(fruits.includes("banana")) console.log(fruits.includes("something not in the array"))
反转它:
if(fruits.includes(message.content)){executed code};
Array.includes的文档。 您正在使用String.includes方法。 或者,您也可以使用indexOf
方法。
我会在这里使用交叉路口。 以防万一你不知道那是什么......
交集是两个 arrays 共有的元素。
例如
swearWords = ["f***", "s***"];
messageWords = ["I", "am", "angry...", "f***", "and", "s***"];
let intersection = messageWords.filter(x => swearWords.includes(x));
console.log(intersection) //-> ["f***", "s***"]
试试这个。
fruits.forEach(fruit => {
if (message.content.includes(fruit)) {
console.log('true')
return;
}
console.log('false')
})
希望这有帮助。
你可以尝试使用Array some方法
const fruits = ["apple", "banana", "orange"]
const containsFruit = fruit => message.content.includes(fruit);
if(fruits.some(containsFruit)) { executed code }
containsFruit 是一个 function,如果在 message.content 中找到水果,它将返回 true
如果发现数组中的任何项目包含在 message.content 中,fruits.some(containsFruit) 将为真
另一种方法是使用正则表达式。
对于大型消息字符串,这可能比在循环中调用 .includes() 与数组(每次都需要遍历整个字符串)更快。 然而,这应该被测试。
let fruits = ["apple", "banana", "orange"]
let fruits_regex = fruits.map(f => f.replace(/(?=\W)/g, '\\')).join('|'); // escape any special chars
// fruits_regex == "apple|banana|orange"
let message = 'apple sauce with oranges';
let matches = [ ...message.matchAll(fruit_regex) ]
// [
// ["apple", index: 0, input: "apple sauce with oranges", groups: undefined]
// ["orange", index: 17, input: "apple sauce with oranges", groups: undefined]
// ]
const fruits = ["apple", "banana", "orange"]
if(fruits.some(x => message.content.includes(x))){
/* executed code */
};
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.